Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial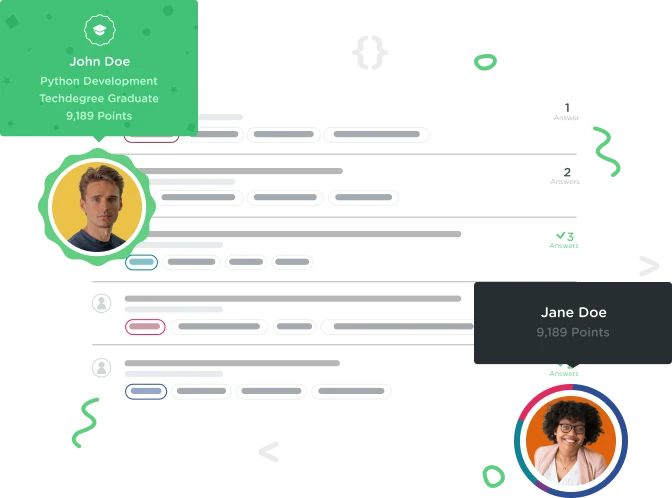
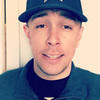
Vince Brown
16,249 PointsPhp Template not rendering when sent in email
I am trying to send an email to the user and support team when the support form is filled out. Currently the email gets sent but the template does not get rendered. Here is the file that parses and send the emails.
<?php
// Sanitize Data
$data = $_POST;
$user_name = filter_var( $data[ 'name' ], FILTER_SANITIZE_STRING );
$user_email = filter_var( $data[ 'email' ], FILTER_SANITIZE_EMAIL );
$user_phone = filter_var( $data[ 'phone' ], FILTER_SANITIZE_STRING );
$user_message = filter_var( $data[ 'message' ], FILTER_SANITIZE_STRING );
if ( is_ajax() )
{
if ( isset( $data['action'] ) && !empty( $data['action'] ) ) {
//if action value exists
$action = $data[ 'action' ];
switch( $action ) { //Switch case for value of action
case "support-request": support_request( $user_name, $user_email ); break;
}
}
}
class OHDC_Email
{
// Render view template
public function render( $file, $data = false )
{
$file = dirname( __FILE__ ) . 'emails/views/checkout-support-' . $file . '.php';
if ( !file_exists( $file ) )
{
return false;
}
if ( $data )
{
extract( $data );
}
ob_start();
include $file;
$body = ob_get_contents();
ob_end_clean();
return $body;
}
public function send( $to, $subject, $file, $data )
{
// Always set content-type when sending HTML email
$headers = "MIME-Version: 1.0" . "\r\n";
$headers .= "Content-type:text/html;charset=UTF-8" . "\r\n";
// More headers
$headers .= 'From: <program@100daychallenge.com>' . "\r\n";
// render email view
$body = $this->render( $file, $data );
mail( $to, $subject, $body, $headers );
}
}
// Verify ajax request
function is_ajax()
{
return isset( $_SERVER[ 'HTTP_X_REQUESTED_WITH' ] ) && strtolower( $_SERVER[ 'HTTP_X_REQUESTED_WITH' ] ) == 'xmlhttprequest';
}
// Send support emails return response with status to ajax call.
function support_request( $user_name, $user_email )
{
$to = $user_email;
// send email to prospect
$prospect_email = new OHDC_Email();
$prospect_subject = 'Challenge Support Request';
$prospect_file = 'prospect';
$prospect_data = array();
$prospect_data['name'] = $user_name;
$prospect_email->send( $to, $prospect_subject, $prospect_file, $prospect_data );
// send email to support team
$response = array(
'status' => 'success',
'name' => $user_name
);
$output = json_encode( $response );
exit($output);
die();
}
?>
Problem
Currently the email gets sent and a success response gets returned but the email is sent with an empty body
any help would be much appreciated Here is a snapshot of the working directory http://cl.ly/1w1J1S140x3M
and here is a link to a gist of the template - https://gist.github.com/vincebrown/97fc33937ab506064a66
1 Answer

Matthew Brock
16,791 Points1st thing to look at is to see if the file actually exists, because if it does not then the body is returned as false. In your render function change this
if ( !file_exists( $file ) )
{
return false;
}
to maybe this
if ( !file_exists( $file ) )
{
return "File not found: " . $file;
}
at least to debug
also you are not doing anything with this code in the render function
if ( $data )
{
extract( $data );
}
Vince Brown
16,249 PointsVince Brown
16,249 PointsThanks Matthew! Return file not found showed me debug suggestions show me I was missing a / in the file path As for the extracting of data That is setting the vars I set in the template to values from the submitted forms. Everything is working great now.
thanks again!