Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial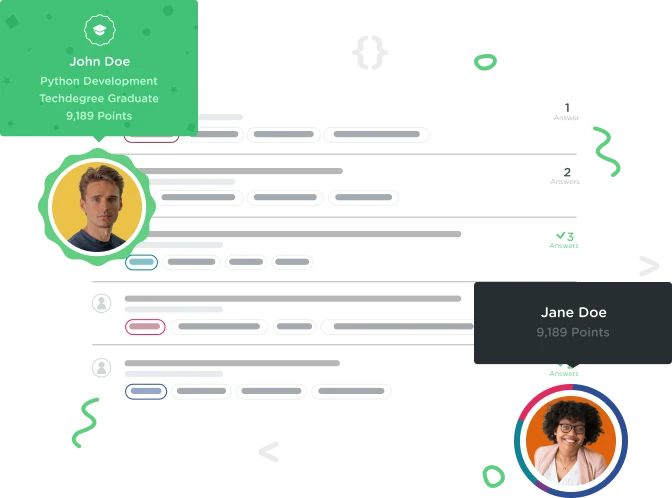
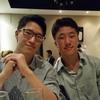
David Choi
8,536 PointsPHP - Variable value not being saved after redirect
I have been practicing some basic PHP skills learned in the Basic PHP track. Specifically, I am practicing inputting data from a form. Here is my code (ignore the bad syntax, this is just a test file I made quickly):
<?php
if ($_SERVER["REQUEST_METHOD"] == "POST") {
$test = $_POST["test"];
header("location:test.php?status=done");
exit;
} ?>
<html>
<head>
</head>
<body>
<form action="test.php" method="POST">
<?php if (isset($_GET["status"]) && $_GET["status"] == "done") {
echo $test;
} ?>
<input type="text" name="test" id="test">
<input type="submit">
</form>
</body>
</html>
However, everytime I submit the form and the browser is redirected, the value of $test is not set. How do I fix this?
2 Answers

Serhii Lihus
6,352 PointsHi there davidchoi2 !
In order to see a problem, you need to go step by step ;)
1) When form is submitted, data sent via POST method to the same page.
<form action="test.php" method="POST">
<?php if (isset($_GET["status"]) && $_GET["status"] == "done") {
echo $test;
} ?>
<input type="text" name="test" id="test">
<input type="submit">
</form>
2) If statement checks for request method, in our case it is POST method
if ($_SERVER["REQUEST_METHOD"] == "POST")
2.1) Assigning value from $_POST['test'] to $test variable
2.2) Making redirect test.php?status=done with status parameter via GET request
3) Passing first condition, cause our request parameters is GET and result will be false
4) Passing second condition because it contain status param.
5) $test variable will be undefined because we haven't declare it.
Answer lies in this step (2.2). All you need to do, is send value of test via GET request.
$test = $_POST["test"] // <-- remove this line
header("location:test.php?status=done&&test=".$_POST['test']);
...
<?php if (isset($_GET["status"]) && $_GET["status"] == "done") {
echo $_GET['test'];
} ?>
Best regards.

Serhii Lihus
6,352 PointsNo, you cannot send both POST and GET request at same time. One request = One method. But of course you can send multiply POST requests as for example.
David Choi
8,536 PointsDavid Choi
8,536 PointsBut doesn't this imply that you can't use POST and GET at the same time? However, I thought that they were two separate arrays. And if we look at the videos in Treehouse, they always use POST and GET at the same time. For example in the Build A Basic PHP Website course, you use POST to get the data from the form submission, and $_GET to redirect the page. So, why isn't this working here?
BTW Thanks for your reply, but I just wanted a more thorough explanation.