Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial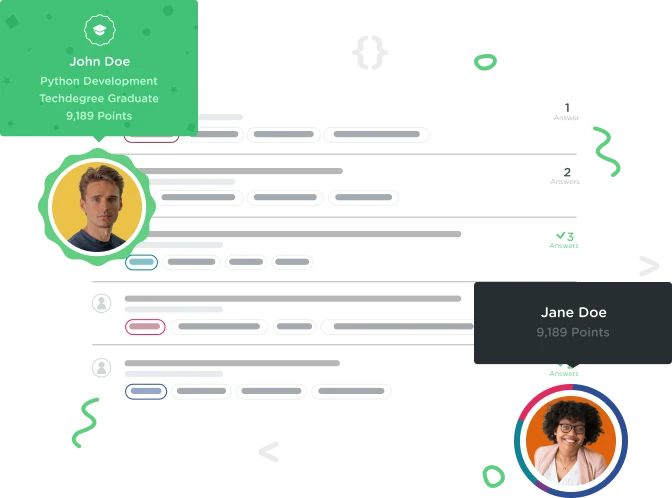
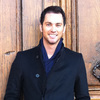
M Fen
9,858 PointsPHP: Working with functions Question 4 of 9. Tell me why i'm wrong!
I got this question right but I only marginally understand why so I'm hoping someone can clear this up.
Why is does the below code output 6? I thought it was 3. I put my logic in comments. What did I get wrong? I'm guessing it has something to do with the $output but I'm not sure what.
<?php
$numbers = array(1,2,3,4); // creates an array of numbers
$total = count($numbers); //counts the numbers starting from 0 So the final count is actually 3.
$sum = 0; //creates a variable called $sum and assigns it the number 0.
$output = ""; // creates a varialbe called $output and assigns it a blank string
$i = 0; // creates a variable called $i and assigns it a number 0.
foreach($numbers as $number) { // loops through the $numbers variable, one at a time and calls each one a $number
$i = $i + 1; // takes the $i variable and adds 1 (which seems to end up at 1 since 0+1=1. )
if ($i < $total) { // if the result of i + 1 (which according to my logic is 1) is less than the total of $numbers (which i believe #numbers is 3) so it is less... then
$sum = $sum + $number; // add the $sum ( I believe is 0 since nothing changed that variable) to the total of $number (which I still believe is 3.)
}
}
echo $sum; // according to my logic is 3. But it's 6. Why?
?>
2 Answers

Gareth Borcherds
9,372 PointsHere is the correction your logic.
<?php
$numbers = array(1,2,3,4); // creates an array of numbers
$total = count($numbers); //this function doesn't count the numbers starting at 0, it actually counts the number of elements in the array, so starting at 0 it counts, 0, 1, 2, 3 which means the total here is equal to 4 not 3.
$sum = 0; //creates a variable called $sum and assigns it the number 0.
$output = ""; // creates a variable called $output and assigns it a blank string
$i = 0; // creates a variable called $i and assigns it a number 0.
foreach($numbers as $number) { // loops through the $numbers variable, one at a time and calls each one a $number this is true, which means because there are 4 elements in the array, this loop will cycle through 4 times.
$i = $i + 1; //The first time $i will be set to 1, the second time through 2, the third time 3, and the fourth time 4
if ($i < $total) { //this if statement will pass the first three times through, but will fail on the 4th because $i will be for and $total is 4 so $i is not less than $total
$sum = $sum + $number;//Given that this statements runs three times the result each of the three times will be
//1 = 0 + 1 1 is the new value of sum, the old value was 0 and we added 1 to it because the first time through our for loop we use the first array element which is one
//3=1+2 this time we add 2 to the old value because we are on the second array element
//6=3+3 this time we add three because we use the 3rd array element,
}
}
echo $sum; //the value will be 6 as shown above. We never add 4 to the string because of the failed if statement $i<$total which shows the correct number and how the logic runs through this code.
?>
I hope this helps
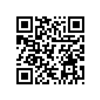
Mike Wojtkowski
8,245 PointsYou have 4 elements in array so $total = count($numbers) will return 4 in this case your foreach will be executed 3 times
i=1 number=1 if ($i < $total) is true sum = 1
i=2 number=2 if ($i < $total) is true sum = 1 + 2 = 3
i=3 number=3 if ($i < $total) is true sum = 3 + 3 = 6
i=4 number=4 if ($i < $total) is false
Gareth was 1 min faster :)