Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial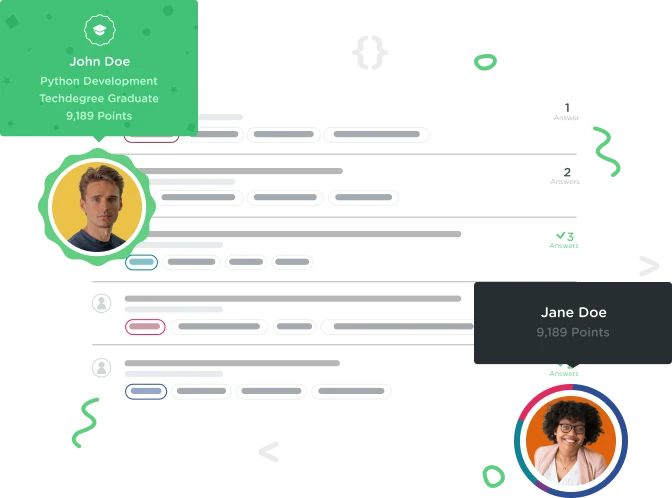

foobarbaz
4,977 PointsphpMailer error? --Mailer error: Could not execute: /usr/sbin/sendmail -t -i ? Does anyone know how to fix this?
I was setting up phpMailer and I checked out the newest example from gitHub and so my code looks like this:
<?php
if( $_SERVER["REQUEST_METHOD"] == "POST") {
$name = trim($_POST["name"]);
$email = trim($_POST["email"]);
$message = trim($_POST["message"]);
/************************ Validation of data *************************/
if( $name == "" || $email == "" || $message == "" )
{
echo "You must specify a value for name.";
exit();
}
foreach( $_POST as $value ) { //For people trying to send messages from your email with your form
if( stripos($value, 'Content-Type:') !== FALSE) {
echo "There was a problem with the information you submitted.";
exit();
}
}
if( $_POST["address"] != "" ) { //For spambots and screen scrapers
echo "Your form submission has an error.";
exit();
}
require('inc/phpmailer/PHPMailerAutoload.php');
$mail = new PHPMailer();
$mail->isSendmail();
if( !$mail->ValidateAddress($email)) {
echo "You must specify a valid email address.";
exit();
}
$email_body = "";
$email_body = $email_body ."<p>". "Name: ".$name."</p>";
$email_body = $email_body ."<p>". "E-mail: ".$email."</p>";
$email_body = $email_body ."<p>"."Message: ".$message."</p>";
date_default_timezone_set('America/New_York');
$mail->SetFrom($email, $name);
$recipientAddress = "myemail@gmail.com";
$mail->AddAddress( $recipientAddress, "myname" );
$mail->Subject = " Contact Form Submission | " . $name;
$mail->MsgHTML($email_body);
if(!$mail->Send()){
echo "Mailer error: " . $mail->ErrorInfo;
exit();
} else {
echo "Message sent!";
}
header("Location: contact.php?status=thanks");
exit;
}
?>
This code is different from the code in the video, but it matches up to the best of my knowledge to the newest sendmail.phps example given in the zip file on gitHub.
I get the error when I fill in all the fields with valid information.
Does anyone know what might be causing the error: Mailer error: Could not execute: /usr/sbin/sendmail -t -i ?
Let me know if you need more informaiton.
Thanks.
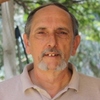
Randolph Judy
Courses Plus Student 28,198 PointsI;ve tested the code below to setup sending with the Gmail SMTP server. I ran this code using XAMPP. I just personalized the applicable lines. I didn't try to send any attachments, but I did successfully send an HTML email to my Outlook account. HTML emails are one place where using tables for alignment is not considered bad practice.
<?php
require_once('phpmailer/class.phpmailer.php');
include("phpmailer/class.smtp.php"); // Received error when I didn't preload this class
$mail = new PHPMailer();
$body = file_get_contents('contents.html');
$body = eregi_replace("[]",'',$body);
$mail->IsSMTP(); // telling the class to use SMTP
$mail->SMTPAuth = true; // enable SMTP authentication
$mail->SMTPSecure = "tls"; // sets the prefix to the server
$mail->Host = "smtp.gmail.com"; // sets GMAIL as the SMTP server
$mail->Port = 587; // set the SMTP port for the GMAIL server
$mail->Username = "yourusername@gmail.com"; // GMAIL username
$mail->Password = "yourpassword"; // GMAIL password
$mail->SetFrom('name@yourdomain.com', 'First Last');
$mail->AddReplyTo("name@yourdomain.com","First Last");
$mail->Subject = "PHPMailer Test Subject via smtp (Gmail), basic";
$mail->AltBody = "To view the message, please use an HTML compatible email viewer!"; // optional, comment out and test
$mail->MsgHTML($body);
$address = "whoto@otherdomain.com";
$mail->AddAddress($address, "John Doe");
$mail->AddAttachment("images/phpmailer.gif"); // attachment
$mail->AddAttachment("images/phpmailer_mini.gif"); // attachment
if(!$mail->Send()) { echo "Mailer Error: " . $mail->ErrorInfo; } else { echo "Message sent!"; } ?>
1 Answer

shezazr
8,275 Pointscan you check if this path exists: /usr/sbin/sendmail
tim221
18,657 Pointstim221
18,657 PointsIt looks like there are no errors with the PHP code but PHPMailer is having a problem running sendmail on the web server. Is your web server set up to send email this way?
One way to proceed is to set up the script using the GMail (or SMTP) PHPMailer example. This will allow you to confirm that everything else is working as expected.
I hope that helps!