Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial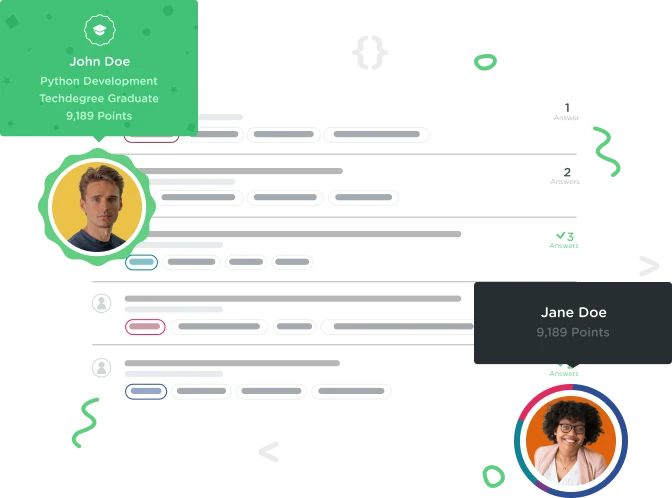
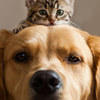
Devin Scheu
66,191 PointsPhython Testing
Question: Add a doctest to average() that tests the function with the list [1, 2]. Because of how we test doctests, you'll need to leave a blank line at the end of your doctest before the closing quotes.
My Code:
def average(num_list):
"""Return the average for a list of numbers
>>> numb_list = (1, 2)
1.5
"""
return sum(num_list) / len(num_list)
2 Answers

Majdi Zrelli
Courses Plus Student 19,262 Pointsthis the right answer:
def average(num_list): """Return the average for a list of numbers
>>> average([1,2])
1.5
"""
return sum(num_list) / len(num_list)

Ken Alger
Treehouse TeacherDevin;
You are not testing the function, average
. The test needs to test average()
with the given list of numbers to get a result of 1.5
. Right now your code is assigning a value to numb_list
and testing if that is equal to 1.5.
Post back if you are still stuck.
Ken
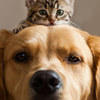
Devin Scheu
66,191 PointsHey can you show me code (Doesn't have to be these challenges) step by step, i'm still not understanding this fully.

Ken Alger
Treehouse TeacherDevin;
Let's look at a different function to test. This will be silly because it simply renames another function, but hopefully it will make some sense.
def add_a_list(num_list):
return sum(num_list)
Pointless, right? For our purposes though it should work. We need to test that if we do `add_a_list([5,3]) that we get the number 8 returned. Our test then, would look similar to:
def add_a_list(num_list):
""" Return the sum of a list of numbers
>>> add_a_list([5,3])
8
"""
return sum(num_list)
The Doctest then will run through the test code, inside the triple quotes, and test if our function returns what we are expecting it to. If it does, great. If it doesn't, we will get an error. Again, for this example it isn't probably super important. What if we are doing some more advanced math though?
That's probably too close to the original challenge for an example, but at the moment it's all I've got.
Post back if you're still stuck.
Ken
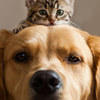
Devin Scheu
66,191 PointsWith your help I figured it out! I was trying to set the argument inside the function, instead of passing the argument into the function in the test. On a side note, you need a space after your add_a_list([5,3]). Thanks again for your help Ken!