Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial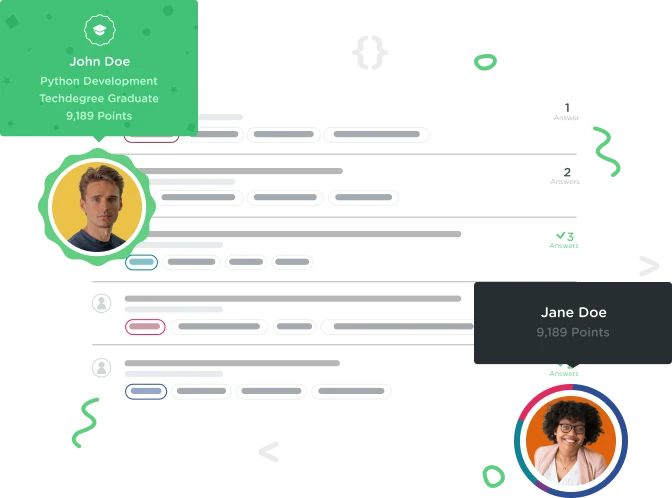

Amit Ghosh
9,314 PointsPlacing Towers on Path
Hi There, I am having a problem in the return statement, if you can please help.
The Tower class: public class Tower { private readonly MapLocation _location; private readonly Path _path;
public Tower( MapLocation location, Path path)
{
if (path.OnPath(location))
_location = location;
else
throw new Exception(location.X + "," + location.Y + "is on path");
}
OnPath Method in Path class:
public bool OnPath( MapLocation loc)
{
foreach (MapLocation onPath in _path)
{
if (onPath.X != loc.X && onPath.Y != loc.Y)
return true;
}
}
I can't return a value for onPath method. I have tried it a couple of different ways but the stuff at the same place. Can someone help me get through this barrier?
Thanks in Advance, Amit.
3 Answers
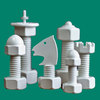
Steven Parker
230,274 PointsIt looks like the "OnPath" method still needs another return after the loop, probably with a "false" value. That way, if the location is not found in the loop, the method still returns a valid boolean result, and "false" would indicate that the location is not on the path..
As it is, I'd expect the compiler is saying "not all paths return a value", right?

Christopher Coscina
5,611 Pointswhat Steven said.... you need to return false if the loop finishes without returning true. The loop goes through every location on the path (one location in each iteration of the loop) to check if the map location passed as an argument to OnMap is the same as one of the locations on the path (the argument is the value put into the parenthesis when you call the function). It figures out if it's on the path by comparing the X and Y coordinates. If it finds a match, then it returns true. If it loops through all locations on the path and doesn't find a match for the location passed to the function, then it should return false because that means it isn't on the path. Your code is close to correct:
public bool OnPath(MapLocation location)
{
foreach (MapLocation onPath in _path)
{
if (onPath.X != location.X && onPath.Y != location.Y)
return true;
}
return false;
}

Amit Ghosh
9,314 PointsYou won't believe I did the same, in the morning and it was still giving me compiler errors. Or maybe I thought I did. I did the changes now and it works. Thanks a lot for the help. Also is this is the best way to return a true and false for a boolean function. Just want to know the best practices before i make it a habit.
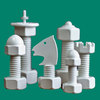
Steven Parker
230,274 PointsI'd say this method is an example of "good practice" for a case where a loop is used in testing. The "return true" ends the method as soon as the outcome is determined, and the "return false" handles the case where the loop doesn't find anything.
Amit Ghosh — You can mark the question solved by choosing a "best answer".
Happy coding!