Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial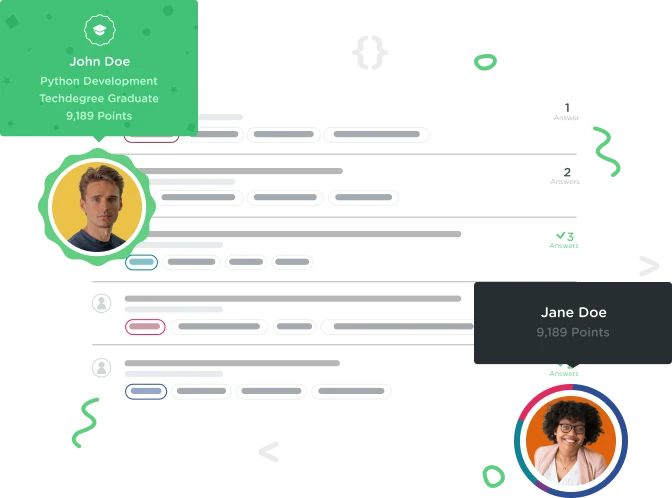
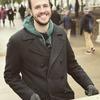
Christian Fincher
3,065 PointsPlacing Towers on Path Challenge
I'm having some difficulty with the challenge and I'm really trying to focus on improving my skills in this language. Unfortunately, I had to view other's attempts at this before I could get the juice flowing but I'm trying to do it my way.
I chose to perform a bool check in my Tower Constructor to pull the answer from the method I created in the Path class. I created a readonly _path object in my class to call that function from the Path class. Using the if/else, I either make the location or get an exception statement.
public Tower(MapLocation location)
{
bool locationCheck;
locationCheck = _path.TowerOnPath(location);
if(locationCheck)
_location = location;
else
throw new OutOfBoundsException("Tower cannot be placed on path.");
}
My TowerOnPath method is a bool method and as follows. I was originally had the type listed as Path for the argument which give me an Operator error during my if-check. It gave me some headaches but I took a step back and corrected that lol
public bool TowerOnPath(MapLocation path)
{
for(int i = 0; i < _path.Length; i++)
{
if (path == _path[i])
{
return true;
}
}
return false;
}
Should this execute and hit the exception if the location lands on the path?
1 Answer
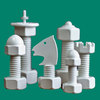
Steven Parker
229,786 PointsSince the location
is a complex object, without extra code a simple equality comparison (==) will only test for identity (reference equality) and not equivalence (value equality). You could implement the IEquatable
interface, which involves creating several methods, but for this task it's easier to compare the coordinates of the objects directly.
Christian Fincher
3,065 PointsChristian Fincher
3,065 PointsI'm definitely not in the ballpark to add any additional code than what I need to at least at this point (lol).
I forgot that comparing the two using the = was a viable way to test this as well and should be helpful! I'll make that adjustment now. Thanks!
Edit - So I think I may have jumped the gun on what you were speaking about. I appreciate you wording it in this way for teaching purposes. So I thought I was comparing the coordinates of the objects through the TowerOnPath when I passed the location. When I performed this, aren't I already comparing the MapLocation objects as a value? I understand that == tests for the reference equality, but testing as a value can't be done in an if conditional so I'm missing a step it seems...
Steven Parker
Steven Parker
229,786 PointsSteven Parker
229,786 PointsYou could do something like this to test the inner values:
if (path.X == _path[i].X && path.Y == _path[i].Y)