Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial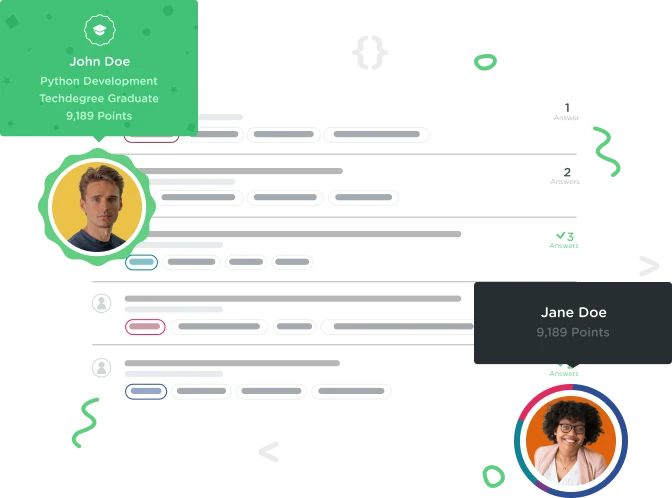

Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 Points'Play Again' button not being shown
The 'Play Again' text is not showing up in mChoice2
in StoryActivity.java
, and you can press both the buttons even if they're not visible and then the contents of Page 0 are shown and the whole story is repeated and I again have to press the invisible buttons, and I go to back to Page 0. No errors are shown. Can you help?
Here is my updated code:
package com.hariidaran.signalsfrommars.model;
import android.graphics.drawable.Drawable;
public class Page {
private Drawable mImage;
private String mText;
private Choice mChoice1;
private Choice mChoice2;
private Boolean mIsFinal = false;
public Page(Drawable imageId, String text, Choice choice1, Choice choice2) {
mImage = imageId;
mText = text;
mChoice1 = choice1;
mChoice2 = choice2;
if (mChoice1 == null && mChoice2 == null) {
mIsFinal = true;
}
}
public Drawable getImage() {
return mImage;
}
public void setImage(Drawable imageId) {
mImage = imageId;
}
public String getText() {
return mText;
}
public void setText(String text) {
mText = text;
}
public Choice getChoice1() {
return mChoice1;
}
public void setChoice1(Choice choice1) {
mChoice1 = choice1;
}
public Choice getChoice2() {
return mChoice2;
}
public void setChoice2(Choice choice2) {
mChoice2 = choice2;
}
public Boolean getFinal() {
return mIsFinal;
}
public void setFinal(Boolean aFinal) {
mIsFinal = aFinal;
}
}
package com.hariidaran.signalsfrommars.model;
import android.content.Context;
import android.content.res.TypedArray;
import com.hariidaran.signalsfrommars.R;
public class Story {
private Page[] mPages;
private TypedArray mImageIds;
private String[] mTexts;
private String[] mChoices1;
private String[] mChoices2;
private int[] mNextPages1;
private int[] mNextPages2;
public Story(Context context) {
mImageIds = context.getResources().obtainTypedArray(R.array.image_ids);
mTexts = context.getResources().getStringArray(R.array.text);
mChoices1 = context.getResources().getStringArray(R.array.choices_1);
mChoices2 = context.getResources().getStringArray(R.array.choices_2);
mNextPages1 = context.getResources().getIntArray(R.array.nextPages_1);
mNextPages2 = context.getResources().getIntArray(R.array.nextPages_2);
mPages = new Page[7];
for (int i = 0; i < mPages.length; i++) {
if (mChoices1[i].equals("(nothing)")) {
mChoices1[i] = null;
}
if (mChoices2[i].equals("(nothing)")) {
mChoices2[i] = null;
}
mPages[i] = new Page(
mImageIds.getDrawable(i),
mTexts[i],
new Choice(mChoices1[i], mNextPages1[i]),
new Choice(mChoices2[i], mNextPages2[i]));
}
}
public Page getPage(int pageNumber) {
return mPages[pageNumber];
}
}
package com.hariidaran.signalsfrommars.ui;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
import com.hariidaran.signalsfrommars.R;
import com.hariidaran.signalsfrommars.model.Page;
import com.hariidaran.signalsfrommars.model.Story;
public class StoryActivity extends Activity {
private String mName;
private Page mPage;
private Story mStory;
private ImageView mStoryImage;
private TextView mText;
private Button mChoice1;
private Button mChoice2;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_story);
Intent intent = getIntent();
mName = intent.getStringExtra(getString(R.string.key_name));
if (mName.equals("")) {
mName = "Friend";
}
mStory = new Story(this);
mStoryImage = (ImageView) findViewById(R.id.storyImage);
mText = (TextView) findViewById(R.id.text);
mChoice1 = (Button) findViewById(R.id.choice1);
mChoice2 = (Button) findViewById(R.id.choice2);
loadPage(0);
}
private void loadPage(int pageNumber) {
mPage = mStory.getPage(pageNumber);
mStoryImage.setImageDrawable(mPage.getImage());
String pageText = mPage.getText();
pageText = String.format(pageText, mName);
mText.setText(pageText);
if (mPage.getFinal()) {
mChoice1.setVisibility(View.GONE);
mChoice2.setText("PLAYAGAIN");
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
finish();
}
});
} else {
mChoice1.setText(mPage.getChoice1().getText());
mChoice2.setText(mPage.getChoice2().getText());
mChoice1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
loadPage(mPage.getChoice1().getNextPage());
}
});
mChoice2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
loadPage(mPage.getChoice2().getNextPage());
}
});
}
}
}
1 Answer

Seth Kroger
56,413 PointsThere's a difference between a null object, and an object with a null member. In your Page constructor you have:
if (mChoice1 == null && mChoice2 == null) {
mIsFinal = true;
}
but in the Story constructor where you are populating the pages you have
for (int i = 0; i < mPages.length; i++) {
if (mChoices1[i].equals("(nothing)")) {
mChoices1[i] = null;
}
if (mChoices2[i].equals("(nothing)")) {
mChoices2[i] = null;
}
mPages[i] = new Page(
mImageIds.getDrawable(i),
mTexts[i],
new Choice(mChoices1[i], mNextPages1[i]),
new Choice(mChoices2[i], mNextPages2[i]));
}
Where you are always constructing Choices, hence they will never be null.
Hariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsHariidaran Tamilmaran
iOS Development Techdegree Student 19,305 PointsThanks a lot, Seth! My app finally works!
Seth Kroger
56,413 PointsSeth Kroger
56,413 PointsFantastic!