Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial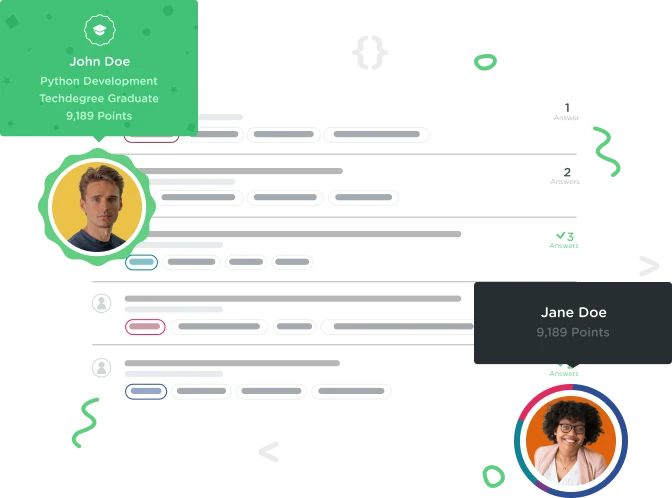

Gabriel Ward
20,222 Points.play() and .stop() methods
I'm a bit confused by this line in the code,
this.songs[this.nowPlayingIndex];
found in the add() and stop() methods of the Playlist Object constructor. Am I correct in thinking that
this.songs[this.nowPlayingIndex];
will always be
this.songs[0];
?

Gabriel Ward
20,222 PointsHi Jonathan,
Here's the full code
function Playlist() {
this.songs = [];
this.nowPlayingIndex = 0;
}
Playlist.prototype.add = function(song) {
this.songs.push(song);
};
Playlist.prototype.play = function() {
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.play();
};
Playlist.prototype.stop = function(){
var currentSong = this.songs[this.nowPlayingIndex];
currentSong.stop();
};
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
} else {
this.play();
}
};

Gabriel Ward
20,222 PointsOk but I'm a bit confused about this.nowPlayingIndex. If it's set to 0, this means that the first song in the array has this.nowPlayingIndex of 0, and then the second song in the array has this.nowPlayingIndex of 1, song three has this.noPlayingIndex of 2, and so on?
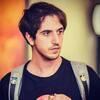
Jonathan Leon
18,813 PointsExactly what you said! :)

Gabriel Ward
20,222 Pointsok and the .stop and .play methods, they're native to Javascript? Because I notice that in the .stop and .play prototype definitions, we've not written any code per se that stops or plays the songs. we've just gotten the current song and then added .stop/.play.
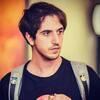
Jonathan Leon
18,813 PointsIf I'm correct they were defined in the beginning in the songs.js file. It made me upset when I realised they were two different functions. but it is because songs is an instance of the Songs constructor which has his own prototypes (stop and play a song)

Gabriel Ward
20,222 PointsOk so .stop and .play have the same definition in songs.js and playlist.js?
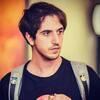
Jonathan Leon
18,813 PointsIf I'm correct Stop and Play in the two files are totally different functions, in order to "work" with functions from different files you need to import them which you will learn in this stage.
the purpose of the stop and play in the song.js will be revealed later on, they will change the class to apply CSS to the song list item to make it appear as if it is playing.

Gabriel Ward
20,222 PointsSo at this point I don't need to get too worried about how the .stop and .play methods in playlist.js work, because at the moment I don't understand how they do anything.
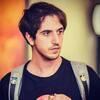
Jonathan Leon
18,813 PointsI wouldn't say this, I'd say you should try to "visualize" what happens in the program as you work through it, and maybe preview changes with console.log and console commands.
I really hope I'm not wrong about the method requiring, I will look into it right now.
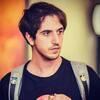
Jonathan Leon
18,813 PointsI was wrong, the reason the functions are "different" are because they are a prototype function of Songs. and song is an instance of the Songs constructor function. therefore the Songs.prototype.play()/stop() functions will be called only on instances of the Songs constructor function. You defined it in the code as :
var songs = new Songs();

Gabriel Ward
20,222 PointsYeah I understand the .stop and .play methods in the song.js file because they have some definition, i.e. isPlaying = true/false. But in the playlist.js they simply appear as currentSong.stop(); currentSong.play();
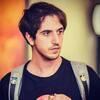
Jonathan Leon
18,813 PointsOhhhhhhh
Its because it's inside the play\stop functions accordingly so it can't call the function from inside the same function :]]]]
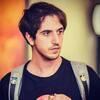
Jonathan Leon
18,813 PointsI'm gonna need to revise that course , thanks Gabriel and goodluck :)

Gabriel Ward
20,222 PointsOk yeah. I'll have to give this time to sink in in my brain. I'm not getting where the definition for .stop() and .play() in playlist.js come from.
1 Answer
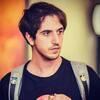
Jonathan Leon
18,813 PointsGreat Gabriel, I can't see anything wrong there , but regarding your question, the instance in which playlist.nowPlayingIndex is redefined is here:
Playlist.prototype.next = function() {
this.stop();
this.nowPlayingIndex++;
if(this.nowPlayingIndex === this.songs.length) {
this.nowPlayingIndex = 0;
} else {
this.play();
}
Basically what it says is: when playlist.prototype.next is called:
- call stop on the playlist (which defines currentSong as the current playingindex and calls stop on currentsong) -increment (add 1) to nowPlayingIndex
- if nowPlayingIndex equals to the maximum numbers of songs in the array, set it back to zero (after last item, it goes back to first) -else, call play function on the playlist (which is similar to stop)
It was pretty weird for me too when I went through this course, but a great way to understand how the program works would be to throw in "console.log(playlist.nowPlayingIndex)" inside the play and stop functions and watch the console while using the playlist.
Best of luck :)
Jonathan Leon
18,813 PointsJonathan Leon
18,813 PointsIt would be great if you could paste the whole code but from the top of my head nowPlayingIndex refers to the currently selected song, and when the "next()" function runs in the event of a click on the next button. nowplayingindex increments by one.