Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial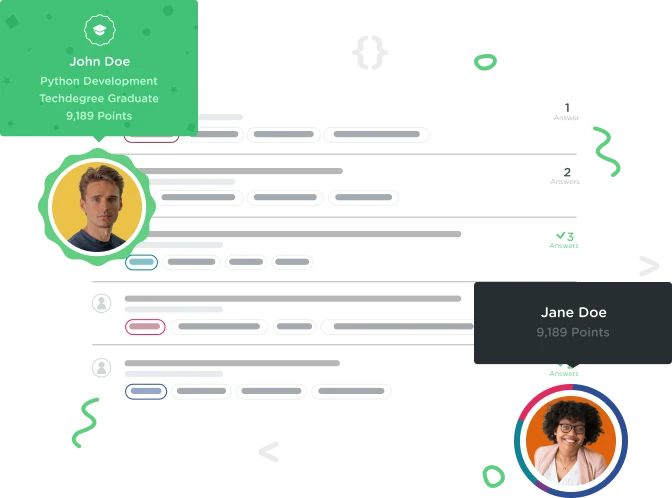

Courtney Pope
Courses Plus Student 1,384 PointsPlayer not defined
import random
CELLS = [(0, 0), (0, 1), (0, 2),
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
monster = random.choice(CELLS)
door = random.choice(CELLS)
start = random.choice(CELLS)
if monster == door or monster == start or door == start:
return get_locations()
return monster, door, start
def move_player(player, move):
x, y = player
if move == 'LEFT':
y -= 1
elif move == 'RIGHT':
y += 1
elif move == 'UP':
x -= 1
elif move == 'DOWN':
x += 1
return x,y
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
if player[1] == 0:
moves.remove('LEFT')
if player[1] == 2:
moves.remove('RIGHT')
if player[0] == 0:
moves.remove('UP')
if player[0] == 2:
moves.remove('DOWN')
return moves
monster, door, start = get_locations()
while True:
moves = get_moves(player)
print("Welcome to The Dungeon")
print("You are currently in room {}".format(player))
print("You can move {}".format(moves))
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == QUIT:
break
if move in moves:
player = move_player(player, moves)
else:
print("** Stop walking into walls**")
continue
if player == door:
print("You've escaped")
break
if player == monster:
print("You were eaten")
break
Keep getting player not defined, but it should be defined as x, y
3 Answers
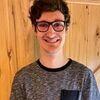
Trent Christofferson
18,846 Pointsit might be because you set y = player and then did if statements changing y but that does not change the value of player. This may or may not be why just throwing ideas out there.

Courtney Pope
Courses Plus Student 1,384 Pointsx, y = player
thats how it was done in the video

Jenna Dalgety
7,791 PointsCourtney,
I had the same problem. Change out all start variables for player variables.
Courtney Pope
Courses Plus Student 1,384 PointsCourtney Pope
Courses Plus Student 1,384 PointsIve edited the question so it can be read better