Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial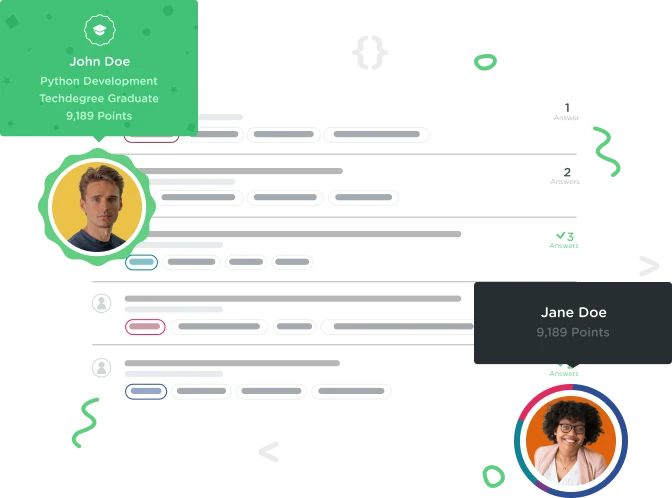
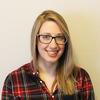
Ashley Boucher
Treehouse TeacherPlayer Properties Brainstorming Discussion
Share your notes, thoughts, and answers to the brainstorming questions for Player Property Brainstorming.
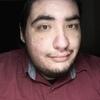
Ruben Ponce
Full Stack JavaScript Techdegree Student 12,035 PointsThe Player class would have a turns between player 1 and player 2. The tokens will have to probably be divided between the total space of the board, and player1/2 will have their own distinct colors. To keep track of whose turn it is, you could have a fixed first turn on player 1, and after his turn it will alternate between player 1 and 2 after they take their respective turns. Or set the first turn to a random number between one and two, and continue the alternation between them from there.

Shaun Wong
14,489 PointsBrainstorming ideas for player class, maybe something like this?
class Player
props
id: 1 ? 2 (set player id after pressing start for p1 or p2)
playerName: name
tokensToWin/score: 0 (4 to win)
totalWins: 0
isTurn: false;
totalTokens: based on board variation
methods
assignColor(id = 1 ? "Red" : "Blue");
checkTokensToWin()
endTurn()
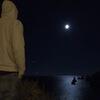
tapio
Full Stack JavaScript Techdegree Graduate 25,832 Pointsproperties
token-color: red || yellow (to differentiate between players) token-total: 21 (if the board is 6 by 7) players turn: something boolean, maybe a switch statement or an if statement...
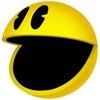
Richard Eldridge
8,229 PointsIs it actually necessary to track a number of tokens? It's like tic-tac-toe; there are only a limited number of spaces, and it's turn based, so the max number of tokens is set by default. One player wins, and the game is over, or it's a draw and the board is full. I think some properties would include a player name (or other identifier like 'Player 1'), a token color, if it is that player's turn. I guess once a player places a token, you could set a variable (i.e. 'isTurn' = true/false). It would have to set both players, and that value would be used to run other functions of game play, and maybe highlighting the active player on the screen. Anyway, just kind of stream-of-consciousness-ing this thing.
I suppose setting an ID for the player could either be set by defalut (like Player 1 and Player 2), or it could be an input (Enter Player 1's name) which could then be marked up on the screen. It wouldn't necessarily change any variables in the game logic, I don't think. I would just be a visual thing. It would have to be reset, though, when the game is over. Anyway, that's what I got.
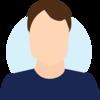
Mike Hatch
14,940 PointsI wrote mine as:
class Player {
id = this.id
name = this.name
tokens = this.tokens
}
Yikes! The next video shows I was a bit off. Maybe it's the React courses I just took. You don't need to explicitly define the constructor
method in React. I also had it backwards. It's this.id = id
.

Félix Guérin
17,910 PointsProperties I think the Player class should have:
- Player ID: string; (chosen by the user)
- Player color: string; (chosen by the user)
- Player token number: number; (Same for both players at the start)
- Player token color: string; (Chosen by user, maybe the same as player color?)
- Player turn: boolean;

Marta Lewandowska
4,988 PointsSome of my notes :)
class Player(){ constructor(){ this.name -> to visually make a differents of the players (player 1 & player 2) this.id -> to set a turn and to asign - win/lost status to it isTurn -> true/false isTurnColor -> when Turn === true change the color to yellow (from blue)
moves ()-> numbers of moves that the player made (half of the places at the board) -> as it is changing during the
game we should create and update it with the help of set
}
}
21 Answers
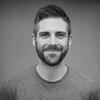
Daniel Cranney
11,609 PointsI tried to keep my quite simple and easy for me to follow.
I would start with something like this....
class Player {
constructor (playerName, tokensRemaining, tokenColor, isTurn ) {
}
}
I would probably then have a method that counts down the tokensRemaining (taking 1 off for each turn), and isTurn would be a boolean.
The playerName and tokenColor, however, would not change after they are set.
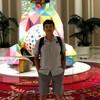
YONGJIN KIM
Full Stack JavaScript Techdegree Graduate 20,287 Pointsturn: boolean color: red || yellow name: string tokenCount: 21

Hugo Javadi
14,559 PointsI think we need: an id with an int value (either 1 or 2); isTurn with a boolean value (I'm not sure if the game class is for running the game, or a game helper. If its a game helper it can have a setter method on it to set the isTurn value to either true or false when necessary); tokensRemaining with an int value; token (an instance of the token class, which will have a colour parameter in its constructor.) Somewhere in the program we need a MAX_TURNS const, possibly in the player class.

Nick Huemmer
Front End Web Development Techdegree Graduate 26,840 PointsHow to differentiate between players - use either their first name (that they enter and is stored as a variable) or use a default player ID that is assigned - player 1 or player 2.
Number of tokens/pieces - Players tokens will be stored in a variable that will be decremented with every turn. The number of tokens will be declared as a variable and decremented with each turn.
Each player's tokens will be differentiated by an assigned color.
You can keep track of turns using game cycles or sessions. The cycles or sessions (turns) are the same number as the maximum number of tokens that a player has unless someone has gotten four in a row of course.

mouhamadoudiouf
14,915 PointsHere are some ideas I found:
Properties
Players: score: turn: boolean (true or false)
To the 'turn' propriety can help toggling between the two players by setting it to "true" or "false"
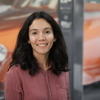
Berlin Galvan
7,145 PointsHere are properties I thought of:
- name (string)
- id (integer)
- tokenColor (string)
- isTurn (boolean)
As for the token amount, I'm thinking Player is set with an amount of tokens half the amount of spaces available on the board. So if there are 42 spaces, then 21 for each player.
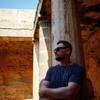
mersadajan
21,306 PointsI think there are not many properties necessary for the player. He needs an ID to identify him, his chosen color and a value that determines if it's his turn. A necessary method would be placing the token. I think it is possible to cut this down even further by setting the user ID to the string of the players color. There are after all only two colors. The turn could be tracked in the Game class as the player only plays every second turn and the method could use the color string in the id to give the token it's color.
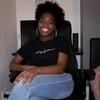
Kaleshe Alleyne-Vassel
8,466 Pointsconstructor(name){ this.name = name; this.token = null; this.isTurn = false; } }
My plan for the Player Object. I don't think it's necessary to set a number of tokens as the game is supposed to end when the board is filled up or someone wins. The colour of the token will be set by the token class I believe and the turn state set to false by default then determined by the game?

Richard Preske
Courses Plus Student 4,356 PointsPlayers name - player1 and player2 in constructor
tokens stored in this.tokens = 13 considering a 5x5 player grid board or just tokens
represent colored tokens with style property, red, yellow?
true/false for player's turn
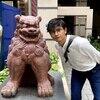
Carlos Chavez
5,691 Points/**
* Differentiate between players through colors or numbers.
* instantiating a player using the class Player
* a token can have a coordinate or identifiable position on the Board
* Every token can have an id and be created using the token class
* The tokens will have different colors, the colors of the players
* Monitoring whose turn it is can be done highlighting the next token to be played, and its color will hint to the player next up.
*/

Hussein Ammar
11,106 PointsI could think of : isTurn: Method as Getter/setter since it is an excellent way to express logic here name: as a property player 1 player 2 Id: as a property token: as a property win: as a method Getter/setter Game: as a method maybe it can be 2/3 game picture: as a property color: as a property

Matt Coale
Full Stack JavaScript Techdegree Graduate 17,884 PointsHere are some of my brainstorming notes in preparation for the game:
/*
class Player {
constructor ()
player: [1 or 2]
turns_remaining: [turns remaining]
player_turn: (true/false)
if (player_turn === true)
it's player1's turn
} else {
it's player2's turn
}
}
*/

Simon Coates
8,209 PointsNot sure it makes sense for the player to know about the turn. If the turn flipping is reliant on the other player, it seems some other class would need to coordinate. I guess you could actually assign a player to a collection of tokens such that the initial order implicitly has a sense of whose turn it is (and also access to player color). I'm sure I'm going to see the solution and think "oh, of course!". So for now, I'd just have name.

Joseph Bertino
Full Stack JavaScript Techdegree Student 14,652 PointsHere is what I would include in my Player constructor
constructor (name, id) {
this.name = name;
this.id = id;
}
I include the name
attribute for aesthetic appeal, as it's more fun to play against a human being than "Player1".
As for distinguishing between players for the purposes of gameplay and turn-taking, providing a unique ID should be sufficient (elsewhere you can maintain the map between id and token color). The id for the player (and thus their color and turn order) can be chosen randomly, or based on the turn order of the previous game.
I don't believe you need to worry about the number of tokens a player "has". The player need not worry how many tokens they still possess, nor how many they played...all that matters is whether the played tokens currently form a winning line.
The player's tokens will be distinguished visually from the other player by mapping the player's ID to a color (black/white, blue/red, whatever pair of colors you want)
I would keep track of the player's turn in the Game object rather than in the player objects. That way you only need one attribute, this.activePlayer
, rather than an attribute per-player, this.isMyTurn
.

Ryan Ekvall
3,889 PointsIf we assign 21 tokens to each player (7x6 game board = 42 spaces/2 = 21 tokens per player) then we can use this information to generate a method to determine whose turn it is.
If Player1's tokens are equal to Player2's tokens then it's Player1's turn. If Player1's tokens are fewer than Player2's tokens then it's Player2's turn.
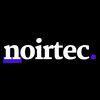
emmanuel egunjobi
4,475 PointsKeeping it simple
playerID = assign user to player 1 or player 2 tokens = a set amount of tokens
class Player {
constructor(playerID, tokens) {
this.playerID = playerID; ///String
this.tokens = tokens; /// number
}
}

Kylie Soderblom
3,890 PointsI believe it is sufficient to have name property only (if allowing players to personalize or simply Player1 || Player 2 if not) and not id as well. I can't see needing both. You just need one property name to identify which player. Note: This assumption was wrong. She recommends both name and id. Time will tell. A boolean "Is Turn" to identify the active player and prevent the inactive player from pitching a token or whatever. I'm still leaning toward a property for Tokens Remaining which may trigger the end of the game. However, "Total Tokens Used" could be included in the Game class instead. The other reason for having tokens, is somewhere, maybe the board, you have to record the position of the tokens and color to identify a winner should there be four in a row. To summarize: name id color is Turn tokens
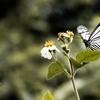
Justin Kao
5,724 Pointsclass Player {
constructor(name) {
this.name = name
this._id = id // not repeat
this.color = color // not repeat
this.isTurn = false
this.isWin = false
}
}
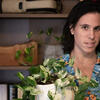
Chris Adams
Front End Web Development Techdegree Graduate 29,422 PointsTaking my turn at brainstorming properties for the Player class!
class Player {
constructor(playerName, playerColour) {
this.playerName = playerName ;
this.playerColour = playerColour ;
this.playerTurn = false;
this.playerTokens = //an integer representing the total number of tokens;
this.playerWinHistory = 0;
}
}
So the Player class will create a Player object. Users will enter a name and select a colour, which is fed into the constructor. The object is instantiated with the playerTurn set to false to avoid conflict with the second player. The playerToken count is generated based on the board size (I'm guess, I don't know this game). Win history is set to 0.

Fahim Ahmed Xec
Full Stack JavaScript Techdegree Graduate 24,565 PointsFor player class, I think we should have the below properties
- Player 1 (red)
- Player 2 (yellow)
- Turns
- Tokens

Ethan Jarvey Ocampo
11,922 PointsI think the players can be differentiated by name (i.e. 'Player 1','Player 2') Visually I think they should be distinguished by the color of the tokens. For each player, I think they should have a number of tokens equal to half of the total number of spaces. These can be stored in a property and use a setter method to reduce the number as game play continues. I think knowing whose turn it is can be a global property or variable of the Game object. Or it could be a property of the Player object, but I think it is slightly more complex than necessary.
Jeffrey Sevinga
8,497 PointsJeffrey Sevinga
8,497 PointsMy take on brainstorming about the Player class