Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial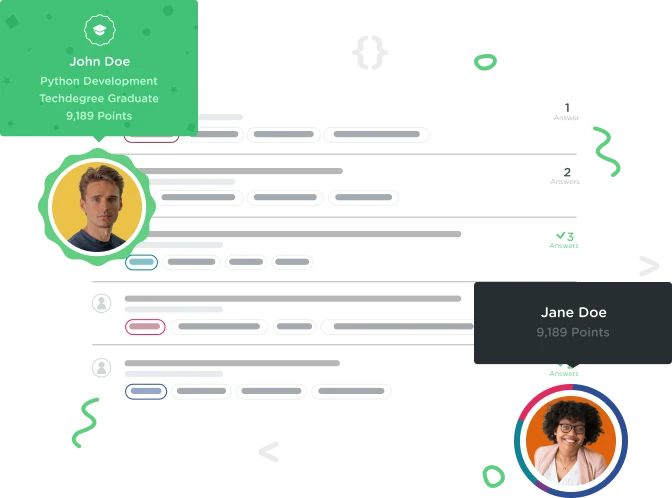

Jacob Domina
Courses Plus Student 1,767 Pointsplayer Randomly dies and error message appears.
After adding the Start and End My player randomly dies and doesn't respawn. Also get an error saying "missing reference Exception". I don't understand how this happened, I reviewed the videos to make sure my codes were incomplete some where, however there was no errors.
1 Answer

Tyler Corum
3,514 PointsDon't give up! I recommend to work at a problem for 15-20 minutes max. If you cannot solve the issue, you need to provide relevant information, such as... when does the player die (hint: it's not random). Is it after you press spacebar, is it while you're moving, are you anywhere close to the bird, is it after 3 or 5 seconds (i.e. is it consistent)?. The code isn't written to respawn the player except for when the proper exit is taken when playerHealth.alive == false (a single = sign sets values; a double == sign operator compares values, check this). Double check your PlayerHealth class file too. Here is my working GameState.cs file and PlayerHealth.cs file. You'll have to check the connections in the Game Manager Game State (script) in the Inspector in Unity, too:
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
using UnityEngine.UI;
using UnityEngine.SceneManagement;
public class GameState : MonoBehaviour {
private bool gameStarted = false;
[SerializeField]
private Text gameStateText;
[SerializeField]
private GameObject player;
[SerializeField]
private BirdMovement birdMovement;
[SerializeField]
private FollowCamera followCamera;
private float restartDelay = 3f;
private float restartTimer;
private PlayerMovement playerMovement;
private PlayerHealth playerHealth;
// Use this for initialization
void Start () {
Cursor.visible = false;
playerMovement = player.GetComponent<PlayerMovement>();
playerHealth = player.GetComponent<PlayerHealth>();
// Prevent the player from moving at the start of the game
playerMovement.enabled = false;
// Prevent bird from moving at start of game
birdMovement.enabled = false;
// Prevent the follow camera from moving to the game position
followCamera.enabled = false;
}
// Update is called once per frame
void Update () {
// If the game is not started and the player presses the spacebar...
if (gameStarted == false && Input.GetKeyUp (KeyCode.Space))
{
// ...start the game.
StartGame();
}
// If the player is no longer alive...
if (playerHealth.alive == false)
{
// ...end the game...
EndGame();
// ...incrememt a timer to count up to restarting...
restartTimer = restartTimer + Time.deltaTime;
// ...and if it reaches the restart delay...
if (restartTimer >= restartDelay)
{
// ...then reload the currently loaded scene
SceneManager.LoadScene(SceneManager.GetActiveScene().buildIndex);
}
}
}
private void StartGame()
{
// Set the game state
gameStarted = true;
// Remove the start text
gameStateText.color = Color.clear;
// Allow the player to move
playerMovement.enabled = true;
// Allow the bird to move
birdMovement.enabled = true;
// Allow the camera to move
followCamera.enabled = true;
}
private void EndGame()
{
// Set the game state
gameStarted = false;
// Show the game over text
gameStateText.color = Color.white;
gameStateText.text = "Game Over!";
// Remove the player from the game
player.SetActive(false);
}
}
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerHealth : MonoBehaviour
{
public bool alive;
[SerializeField]
private GameObject pickupPrefab;
// Use this for initialization
void Start()
{
alive = true;
}
void OnTriggerEnter(Collider other)
{
// ...If the collider other is tagged with player...
// ...aka if the bird runs into the player...
if (other.CompareTag("Enemy") && alive == true)
{
alive = false;
// Create the pickup particles
Instantiate(pickupPrefab, transform.position, Quaternion.identity);
//// ...decrease total number of flies...
//FlySpawner.totalFlies = 0;
//// ...update the score...
//ScoreCounter.score = 0;
//Destroy(gameObject);
}
}
}
Keep in mind in my PlayerHealth class I was messing around with the code before I watched the video in how I would reset things. It is commented out, but I'm leaving it in my codeblock .