Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial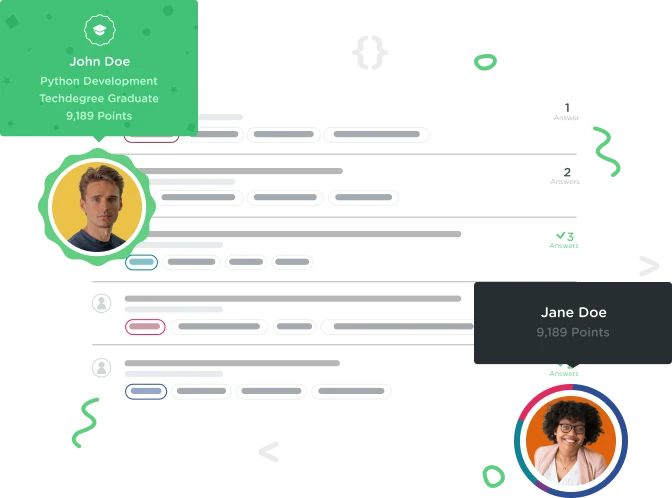

Jose Coto
11,466 PointsPlayer regex
I'm having problems to complete the task of generating the class Player. Help will be appreciated.
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.match(r"(?P<last_name>[\w]+\s?[\w]+?),\s(?P<first_name>[\w]+\s?[\w]+?):\s(?P<score>[\d]+)", string, re.M)
class Player
def __init__(self):
for match in players.finditer(string):
self.last_name = match.group("last_name")
self.first_name = match.group("first_name")
self.score = match.group("score")
4 Answers

Iain Simmons
Treehouse Moderator 32,305 PointsCheck your syntax for creating a class and the indentation for the __init__
method within it.

Jose Coto
11,466 PointsThank you for catching my mistakes. I wasn't sure if you could use the init with a loop but it turns that you can! By the way do you have any clues on how to get all the last names and not the last one? Thats the result that I have when I instantiate the class. Thanks again!
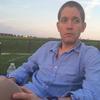
Drew Butcher
33,160 PointsI think it's just indentation and a colon.... try
class Player():
def __init__(self):
for match in players.finditer(string):
self.last_name = match.group("last_name")
self.first_name = match.group("first_name")
self.score = match.group("score")

Iain Simmons
Treehouse Moderator 32,305 PointsOkay, so a couple things regarding your regex syntax:
- If you only have one thing in the character class (between the square brackets
[
...]
), then you don't need them, since they will match any of the multiple characters within them. - Using
+?
together after a character or group makes it a lazy quantifier, which basically means it will return as few matches as possible. Try using the asterisk/star symbol (*
), since it means 'zero or more', but is also 'greedy', and therefore will return as many matches as possible.