Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial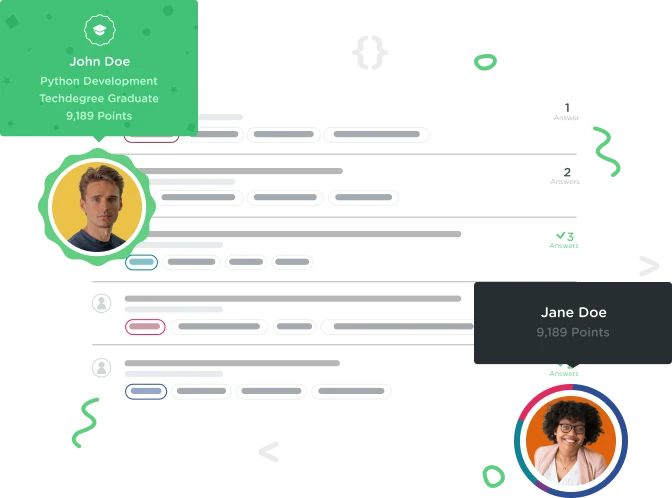

Mathew Yangang
4,433 Pointsplayer_move
I thought the wall was 0, and hp will reduce to 5, but code still not passing
# EXAMPLES:
# move((1, 1, 10), (-1, 0)) => (0, 1, 10)
# move((0, 1, 10), (-1, 0)) => (0, 1, 5)
# move((0, 9, 5), (0, 1)) => (0, 9, 0)
def move(player, direction):
x, y, hp = player
if move(player) == 0:
hp -= 5
return x, y, hp
2 Answers
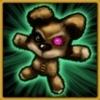
Dave Harker
Courses Plus Student 15,510 PointsHi Mathew Yangang,
I've got to step away for a while, so I'll put this here just in case ...
- If you've not tried working through the challenge with my previous answer but want to then
STOP READING NOW
However ...
- If you've hit a snag, or just want to see another solution
SCROLL DOWN
I've well and truly over commented this code to explain what is happening at each step.
I hope it helps, or at the very least demonstrates another way the challenge can be tackled.
def move(player, direction):
# assign player x_pos, y_pos, and hp to local variables
x, y, hp = player
# assign proposed new player x and y axis modifications to local variables
proposed_x_mod, proposed_y_mod = direction
# attempts to modify player position (either x or y depending on axis pos passed in)
def modify_position(pos, pos_mod, hp):
# check for good/valid/non-damaging move first
if pos + pos_mod >= 0 and pos + pos_mod <= 9:
pos += pos_mod # move to modified position on axis
else: # out of bounds move detected (wall hit)
# no matter which wall was hit, they lose hp. So let's fix that up first
hp -= 5
# now let's see which wall was hit and adjust player position to axis wall position
if pos + pos_mod < 0:
pos = 0 # they hit the 0 wall, so let's set them to the 0 position (not OoB)
else: # no need for condition check here
pos = 9 # they obviously hit the 9 wall instead, so let's set that position
# return the position and hp resulting from move
return pos, hp
# call modify_position on axis only if there was an axis position modification proposed
if proposed_x_mod != 0: # if it equaled 0 then the player position isn't changing on this axis
# if there was a proposed position change, let's determine how it impacts the player
x, hp = modify_position(x, proposed_x_mod, hp)
if proposed_y_mod != 0: # as above but for the proposed_y_mod (checking other axis)
y, hp = modify_position(y, proposed_y_mod, hp)
# return players x, y axis position and current hp after move
return x, y, hp
Best of luck moving forward. Happy coding
Dave
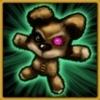
Dave Harker
Courses Plus Student 15,510 PointsHi Mathew Yangang,
The axis has a range between 0 and 9 for each axis (x and y).
With 0 and 9 being the wall. You'll need to check if:
- the new axis position move is within allowable range (0-9) after modification to position is taken into account
- if it is, modify the position by the position modification
- if it isn't reduce the player hp (5) and then set the axis position to the wall they hit (if they went below 0, set to 0 - if above 9, set to 9) on whatever axis the move was made against
Rather than duplicating code, I'd suggest writing an internal function that does it (axis independent), then just check if the new x move changes the player x position, if it does, call that internal function on the x axis ... same for y (hope that makes sense). That way you're only checking and modifying if there has been a position change request for that particular axis. I can write it up for you and comment it all for you if you like ... but I'd recommend having a think about that first
If you're still stuck, let me know. ( we can work through the code )
Dave
Mathew Yangang
4,433 PointsMathew Yangang
4,433 PointsThanks a lot Dave, this was very helpful. I really appreciate
Sam Frampton
3,796 PointsSam Frampton
3,796 PointsThanks for posting this! Really helped me to move forward.