Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial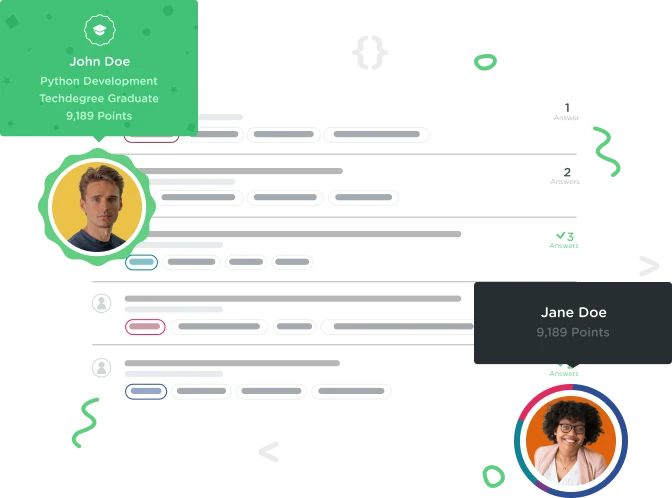

Blake Handson
1,642 PointsPlayer's position is not updating after they choose a direction to move in. Why ?
import random
CELLS = [(0, 0), (0, 1), (0, 2), #CAPS = Constant
(1, 0), (1, 1), (1, 2),
(2, 0), (2, 1), (2, 2)]
def get_locations():
# monster = random
monster = random.choice(CELLS)
# door = random
door = random.choice(CELLS)
# start = random
start = random.choice(CELLS)
if monster == door or monster == start or door == start:
return get_locations()
return monster, door, start ## return monster, door and start if they aren't in the
#same position
# if monster, door, or start are the same, do it again
# return monster, door, start
#passes in players current location and there request move direction i.e (0,1) & ('LEFT')
def move_player(player, move):
#player is - (x,y)
# Get the player's current location
x, y = player
#using elif here because only one can be true and they
##might not type left, right, up or down so it allows you to
# If move is LEFT, y - 1
if move == 'LEFT':
y -= 1
# If move is RIGHT, y + 1
elif move == 'RIGHT':
y += 1
# If move is UP, x - 1
elif move == 'UP':
x -= 1
# If move is DOWN, x + 1
elif move == 'DOWN':
x += 1
return player
def get_moves(player):
moves = ['LEFT', 'RIGHT', 'UP', 'DOWN']
# if player's y is 0, remove LEFT
if player[1] == 0:
moves.remove('LEFT')
# if player's y is 2, remove RIGHT
if player[1] == 2:
moves.remove('RIGHT')
# if player's x is 0, remove UP
if player[0] == 0:
moves.remove('UP')
# if player's x is 2, remove DOWN
if player[0] == 2:
moves.remove('DOWN')
return moves
monster, door, player = get_locations()
while True:
moves = get_moves(player)
print("Welcome to the dungeon!")
print("You're currently in room {}".format(player)) # fill in with player position
print("You can move {}".format(moves)) # fill in with available moves
print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in moves:
player = move_player(player, move)
else:
print("**Hey, that's a wall, stop walking into them!**")
continue
if player == door:
print("Congrats you escaped")
break
elif player == monster:
print("Damn dude, you got eaten!")
break
# If it's a good move, change the player's position
# If it's a bad move, don't change anything
# If the new player position is the door, they win!
# If the new player position is the monster, they lose!
# Otherwise, continue

Seth Kroger
56,413 PointsThe backticks need to be on lines of their own.
1 Answer

Seth Kroger
56,413 PointsAfter changing x or y you still have to put those coordinates back into player before returning or return the tuple (x, y), otherwise you're returning the original position of the player.

Blake Handson
1,642 PointsThanks for that Seth, oh and also for the formatting tip >_<
Blake Handson
1,642 PointsBlake Handson
1,642 PointsPs how do I add my code so that it is formatted ? Thanks.