Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial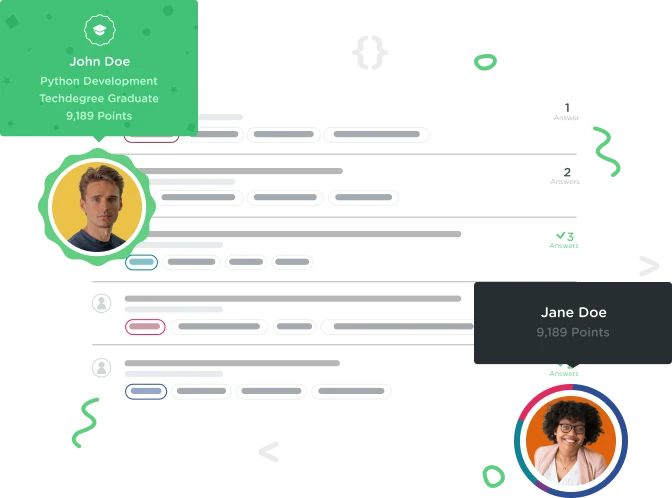

Einars Vilnis
8,050 Pointsplayers.py
Hi Guys !
Could You please help me with this challenge. Sorry . What I meant is, I don't understand How to put 'players' in to a class I am lost this is what I came up with
import re
string = '''Love, Kenneth: 20
Chalkley, Andrew: 25
McFarland, Dave: 10
Kesten, Joy: 22
Stewart Pinchback, Pinckney Benton: 18'''
players = re.search(r'''
^(?P<name>(?P<last_name>[\w ]*),\s(?P<first_name>[\w ]*)):\s
(?P<score>[\d]*)$
''', string, re.MULTILINE|re.X)
class Player:
def __init__(self, last_name, first_name, score):
self.last_name = last_name
self.first_name = first_name
self.score = score
def player(self, string):
players = re.compile(r'''
^(?P<name>(?P<last_name>[\w ]*),\s(?P<first_name>[\w ]*)):\s
(?P<score>[\d]*)$
''', re.MULTILINE|re.X)
for match in players.finditer(string):
return ('{first_name} {last_name} <{score}>'.format(**match.groupdict()))

Einars Vilnis
8,050 PointsI updated my question, could you tell me, where is the problem? please?
3 Answers
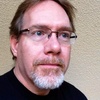
Chris Freeman
Treehouse Moderator 68,441 PointsYour solution is very close. If using **kwargs
you will need to unpack them, but if the challenge calls your __init__()
method without key word arguments, it will fail.
players = re.search(r'''
^(?P<last_name>[\w ]*),\s(?P<first_name>[\w ]*):\s
(?P<score>[\d]*)$
''', string, re.MULTILINE|re.X)
class Player():
def __init__(self, last_name, first_name, score): # <-- add expected parameters
self.last_name = last_name #<-- add missing right-hand expression
self.first_name = first_name
self.score = score
The __init__
parameters could also have been written using default values
def __init__(self, last_name='last_name', first_name='first_name', score='score'):

Einars Vilnis
8,050 Pointsthis is my latest try. I must be missing some understanding it shows"init has got an unexpected argument 'name'"
class Player:
def __init__(self, last_name, first_name, score):
players = re.compile(r'''
^(?P<name>(?P<last_name>[\w ]*),\s(?P<first_name>[\w ]*)):\s
(?P<score>[\d]*)$
''', re.MULTILINE|re.X)
self.last_name = players.search(string).groupdict('last_name')
self.first_name = players.search(string).groupdict('first_name')
self.score = players.search(string).groupdict('score')
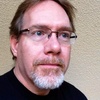
Chris Freeman
Treehouse Moderator 68,441 PointsYou don't need to re-evaluate the regex search for the 2nd part of the challenge. Simply assign the parameters to the class attributes.

Einars Vilnis
8,050 PointsSorry . What I meant is, I don't understand How to put 'players' in to a class

Einars Vilnis
8,050 PointsThank You! But I just don`t get it! I simply don't understand this

Einars Vilnis
8,050 PointsAm I getting closer?
```class Player:
players = re.compile(r'''
^(?P<name>(?P<last_name>[\w ]*),\s(?P<first_name>[\w ]*)):\s
(?P<score>[\d]*)$
''', re.MULTILINE|re.X)
def __init__(self, **kwargs):
self.last_name = players.group('last_name')
self.first_name = players.group('first_name')
self.score = players.group('score')
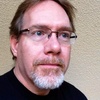
Chris Freeman
Treehouse Moderator 68,441 PointsMy original reply is a working solution. The biggest issue with your current solution is the way your are trying to use **kwargs
.
Your code will only work if the challenge grader calls the __init__()
method with keyword argument. Which it doesn't in this case. Look again at how I defined the parameters to the __init__()
method.
With parameters passed to the __init__()
method, you simply assign them each of the parameters directly to class attributes.
Lastly, the __init__()
method doesn't need any regex coding.

Einars Vilnis
8,050 PointsI must be missing something somewhere, coz i Just dont get it , this is my latest try
```class Player:
players = re.compile(r'''
^(?P<name>(?P<last_name>[\w ]*),\s(?P<first_name>[\w ]*)):\s
(?P<score>[\d]*)$
''', re.MULTILINE|re.X)
for match in players.finditer(string):
print('{first_name} {last_name} <{score}>'.format(**match.groupdict()))
def __init__(self, last_name = 'last_name', first_name = 'first_name', score = 'score'):
self.last_name = last_name
self.first_name = first_name
self.score = score
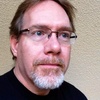
Chris Freeman
Treehouse Moderator 68,441 PointsIn your latest attempt, you've replaced re.search()
with re.compile()
which breaks the first part of the challenge.
For the second part, you've removed the class Player()
declaration, which breaks the second half. And you're still using regular expressions in the __init__()
to recreated the parameter values. The values are already being set by the grader as it uses your re.search pattern to get the matching groups.
The code necessary to pass the challenge will not run on it's own (without the grader). The grader runs the code, then tries to create an instance of Player()
using the output of the re.search
. If the attributes are assigned correctly in the Player instance, the challenge passes.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsWhat is confusing you? In software development you need to formulate your questions succinctly. "What is meant by...", "I don't understand what this error means...."