Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial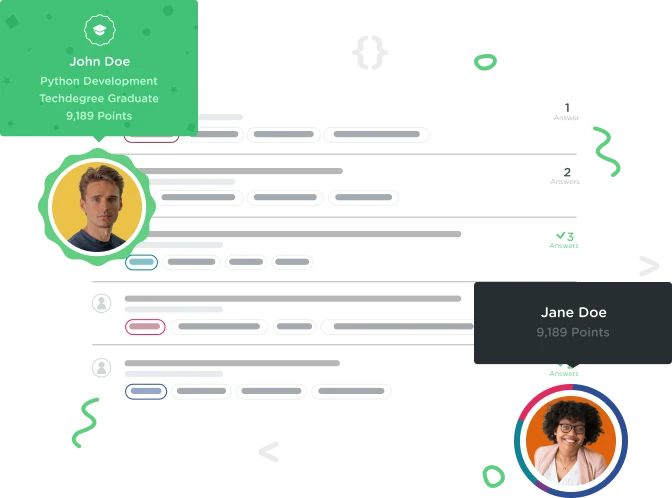

Anthony C
4,636 PointsPlaylist in the parameter songs
How do you store the playlist in the parameter songs????? how does the code know that the 'songs' parameter is refering to the playlist?
4 Answers

andren
28,558 PointsWhen you call a function the first argument you provide is automatically passed in and assigned to the first parameter of the function.
Since playList
is the first argument provided when the function is called, and songs
is the first parameter defined that is where the value of playList
ends up.
So this line:
printSongs(playList);
Is essentially just saying, take the contents of playList
and pass it in as the first argument. And this code:
function printSongs( Songs )
Is essentially saying, take the first argument passed to this function and assign it to a variable called Songs
within the function. At no point does JavaScript care about whether the names of the argument and parameter match up. That's simply not a part of how the pairing works, it is based entirely on the position of the argument and parameter.
If you had multiple arguments and parameters it would work in the same way. It would pair the second argument to the second parameter, the third argument to the third parameter and so on.

Anthony C
4,636 PointsThank you for the explanation!

Yu-Chien Huang
Courses Plus Student 16,672 Pointsi have further question for this: why cant we set the parameter the same name as the argument? Is it not more consistent in coding ?

andren
28,558 PointsYou certainly can name the argument and the parameter the same thing, nothing prevents you from doing so.
The reason why that was not done in this code is likely for two reasons:
- To make it clear that arguments and parameters are not linked based on their name. It's pretty common that people that start out with coding think that arguments and parameters have to be named the same thing to work.
- To make it more clear that the argument and parameter are not the same variable. In other words when you call a method with an argument what is passed is just the value stored in the variable, the variable itself is not passed. Meaning that changes to the parameter variable does not reflect back to the argument variable. Which is something people might think will be the case if they are named the exact same thing.
Basically take this code:
var message = "Hello";
function test(message) {
message = "World";
}
test(message);
console.log(message);
If you knew nothing about arguments and parameters it would not be too unlikely that you would guess that the argument gets assigned to the parameter based on them sharing the same name. And that the variables are linked such that when message
is assigned a value within the test
function that will result in the message
variable outside the function to change as well.
I've seen many students have that exact misunderstanding about arguments and parameters, so going out of your way to avoid that confusion is sensible for a teacher.
But if you changed the code for this lesson into this:
var playList = [
['I did it my way', 'Frank Sinatra'],
['Respect', 'Aretha Franklin'],
['Imagine', 'John Lennon'],
['Born to Run', 'Bruce Springstein'],
['Louie Louie', 'The Kingsmen'],
['Mabellene', 'Chuck Berry']
];
function print(message) {
document.write(message);
}
function printSongs(playList) {
var listHTML = '<ol>';
for (var i = 0; i < playList.length; i += 1) {
listHTML += '<li>' + playList[i][0] + ' by ' + playList[i][1] + '</li>';
}
listHTML += '</ol>';
print(listHTML);
}
printSongs(playList);
Then it would still work perfectly fine.

Yu-Chien Huang
Courses Plus Student 16,672 PointsWow, Arden, thank u so much for explaining it very clearly!!!!! I tried to ask around people about it.....somehow they just told me dont put the same name to parameter and argument without explanation!! Finally, i get it after your explanation!!! Thank u again!!