Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial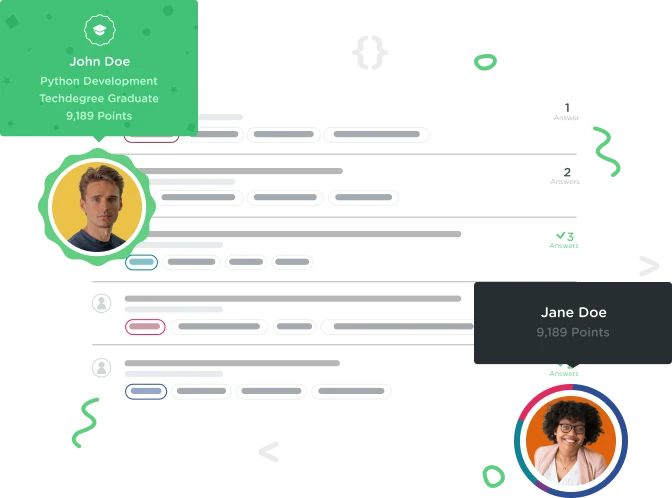

Florian Jensen
597 PointsplaySound() throwing NPE
When getting to the point that it is supposed to play the sound, I for some reason get a NPE.
My MainActivity.java:
package com.example.crystalball;
import android.app.Activity;
import android.graphics.drawable.AnimationDrawable;
import android.media.MediaPlayer;
import android.os.Bundle;
import android.view.View;
import android.view.animation.AlphaAnimation;
import android.widget.Button;
import android.widget.ImageView;
import android.widget.TextView;
public class MainActivity extends Activity {
private CrystalBall mCrystalBall = new CrystalBall();
private TextView mAnswerLabel;
private Button mGetAnswerButton;
private ImageView mCrystalBallImage;
/**
* Called when the activity is first created.
*/
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//Assigning Views from the layout file
mAnswerLabel = (TextView) findViewById(R.id.textView1);
mGetAnswerButton = (Button) findViewById(R.id.button1);
mCrystalBallImage = (ImageView) findViewById(R.id.imageView);
mGetAnswerButton.setOnClickListener(new View.OnClickListener() {
public void onClick(View arg0) {
String answer = mCrystalBall.getAnAnswer();
//Update the label with our dynamic answer
mAnswerLabel.setText(answer);
animateCrystalBall();
animateAnswer();
playSound();
}
});
}
private void animateCrystalBall() {
mCrystalBallImage.setImageResource(R.drawable.ball_animation);
AnimationDrawable ballAnimation = (AnimationDrawable) mCrystalBallImage.getDrawable();
if (ballAnimation.isRunning()) {
ballAnimation.stop();
}
ballAnimation.start();
}
private void animateAnswer() {
AlphaAnimation fadeInAnimation = new AlphaAnimation(0, 1);
fadeInAnimation.setDuration(1500);
fadeInAnimation.setFillAfter(true);
mAnswerLabel.setAnimation(fadeInAnimation);
}
private void playSound() {
MediaPlayer player = MediaPlayer.create(this, R.raw.crystal_ball);
player.start();
player.setOnCompletionListener(new MediaPlayer.OnCompletionListener() {
public void onCompletion(MediaPlayer mp) {
mp.release();
}
});
}
}
And the log from my test run:
05-04 14:44:36.987 14944-14944/com.example.crystalball I/dalvikvm-heap? Grow heap (frag case) to 15.194MB for 1825216-byte allocation
05-04 14:44:37.347 14944-14944/com.example.crystalball E/MediaPlayer? Unable to create media player
05-04 14:44:37.357 14944-14944/com.example.crystalball D/MediaPlayer? create failed:
java.io.IOException: setDataSourceFD failed.: status=0x80000000
at android.media.MediaPlayer._setDataSource(Native Method)
at android.media.MediaPlayer.setDataSource(MediaPlayer.java:1282)
at android.media.MediaPlayer.create(MediaPlayer.java:1006)
at com.example.crystalball.MainActivity.playSound(MainActivity.java:66)
at com.example.crystalball.MainActivity.access$400(MainActivity.java:13)
at com.example.crystalball.MainActivity$1.onClick(MainActivity.java:43)
at android.view.View.performClick(View.java:4480)
at android.view.View$PerformClick.run(View.java:18686)
at android.os.Handler.handleCallback(Handler.java:733)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:157)
at android.app.ActivityThread.main(ActivityThread.java:5872)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:515)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:852)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:668)
at dalvik.system.NativeStart.main(Native Method)
05-04 14:44:37.357 14944-14944/com.example.crystalball W/dalvikvm? threadid=1: thread exiting with uncaught exception (group=0x4170be18)
05-04 14:44:37.357 14944-14944/com.example.crystalball E/AndroidRuntime? FATAL EXCEPTION: main
Process: com.example.crystalball, PID: 14944
java.lang.NullPointerException
at com.example.crystalball.MainActivity.playSound(MainActivity.java:67)
at com.example.crystalball.MainActivity.access$400(MainActivity.java:13)
at com.example.crystalball.MainActivity$1.onClick(MainActivity.java:43)
at android.view.View.performClick(View.java:4480)
at android.view.View$PerformClick.run(View.java:18686)
at android.os.Handler.handleCallback(Handler.java:733)
at android.os.Handler.dispatchMessage(Handler.java:95)
at android.os.Looper.loop(Looper.java:157)
at android.app.ActivityThread.main(ActivityThread.java:5872)
at java.lang.reflect.Method.invokeNative(Native Method)
at java.lang.reflect.Method.invoke(Method.java:515)
at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:852)
at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:668)
at dalvik.system.NativeStart.main(Native Method)
05-04 14:44:40.147 14944-14944/com.example.crystalball D/Process? killProcess, pid=14944
05-04 14:44:40.147 14944-14944/com.example.crystalball D/Process? com.android.internal.os.RuntimeInit$UncaughtHandler.uncaughtException:131 java.lang.ThreadGroup.uncaughtException:693 java.lang.ThreadGroup.uncaughtException:690
This is using API version 19.
2 Answers

Florian Jensen
597 PointsAfter looking around, I have found the reason for the crash. For some reason, downloading via Safari didn't create a valid file. I simply re-downloaded the mp3 via wget, and replaced it in the raw. Now it works like a charm.
Endre Juhasz
613 PointsThat didn't help me.