Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial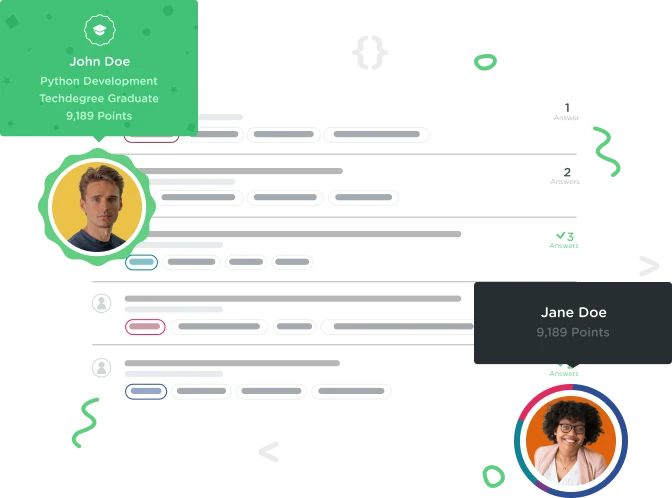

lukej
34,222 PointsplayToken, moveLeft and moveRight methods
I had to tweak the moveLeft and moveRight methods a bit to get them to work. The .style.left
property does not seem to accept numeric values for some reason.
moveLeft() {
if (this.columnLocation > 0) {
this.htmlToken.style.left = `${this.offsetLeft - 76}px`;
this.columnLocation -= 1;
}
}
moveRight(columns) {
if (this.columnLocation < columns - 1) {
this.htmlToken.style.left = `${this.offsetLeft + 76}px`;
this.columnLocation += 1;
}
}
Note: I had to assign this.htmlToken.style.left
a string value for example "195px"
instead of 195
as a numeric value as shown in the video.
Does anyone know what is going on here?
2 Answers
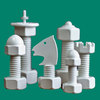
Steven Parker
231,275 PointsI think you may have discovered a bug in the course! I believe style properties are always strings, plus there's the added factor of needing to specify a unit (such as "px") for any value other than 0.
You may want to make a bug report to the Support department. And we can try tagging Ashley Boucher in case she would like to comment directly.
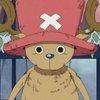
Elliott LaJoie
15,259 PointsThis is exactly what I needed! I haven't brushed up on my css in awhile so I appreciate that!
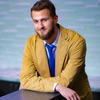
Alex Franklin
12,403 Pointshow did you get the play token / drop to work on keyDown - I am now stuck trying to figure that out... ANY ANSWERS??

lukej
34,222 PointsThis is the code that plays and drops the token. I don't know if it is different from the original though. I hope this helps:
playToken() method in the Game.js file:
/*
* find space object to drop token into; drop token
*/
playToken() {
const spaces = this.board.spaces;
const activeToken = this.activePlayer.activeToken;
const targetColumn = spaces[activeToken.columnLocation];
let targetSpace = null;
for (let space of targetColumn) {
if (space.token === null) {
targetSpace = space;
}
}
if (targetSpace !== null) {
console.log('token played');
game.ready = false;
// second argument is a callback function, the callback function is
// executed when the .drop function has finished.
activeToken.drop(targetSpace, () => {
game.updateGameState(activeToken, targetSpace);
});
console.log('token dropped');
}
}
drop() method in the Token.js file:
/*
* Drops html token into targeted board space.
* @param {Object} target - Targeted space for dropped token.
* @param {function} reset - function to call after animation is complete
*/
drop(target, reset) {
this.dropped = true;
$(this.htmlToken).animate({
top: (target.y * target.diameter)
}, 750, 'easeOutBounce', reset);
}
JASON PETERSEN
14,266 PointsJASON PETERSEN
14,266 PointsThank you so much! I was running into the same thing. left and right wasn't working. I kept thinking "isn't it supposed to have px on the end." Idk how many times I re-watched the video trying to find where my code differed.
Makes me wonder how her token was even able to move in the video....