Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial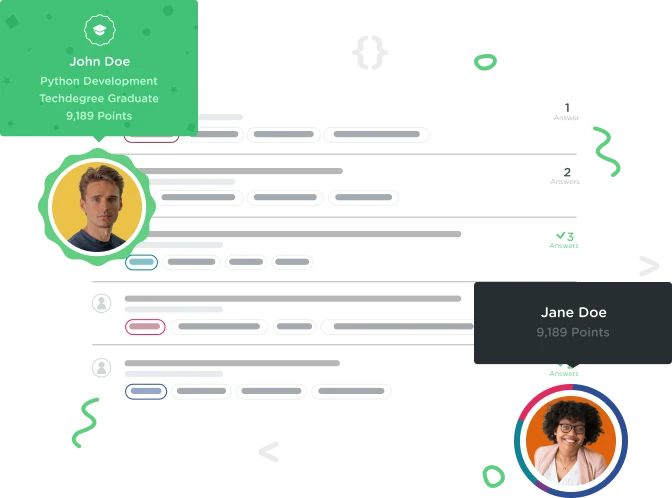

SHAWNELL HARRISON
16,282 PointsPlease can someone help
I have been stuck on this for days. Can someone please help?
1 Answer
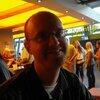
Robert Gulley
Front End Web Development Techdegree Student 10,722 PointsHi Shawnell! -
I'm not sure which question you are struggling with, or if you're struggling with all of them. The difference between while and do...while loops can be challenging at first. I'm going to go through each of the questions and try to give some explanation on each.
Given the code below, what will display in the console?
do {
console.log('Hello');
} while (false);
console.log('Goodbye');
This will display the following:
Hello
Goodbye
This is because when we get to this code, the condition is true. If this were a while statement, this code inside would not execute at all, but do...while loops always execute at least once before checking the condition. Therefore, the hello in this code will print.
Given the code below, what will display in the console?
while (false) {
console.log('Hello');
}
console.log('Goodbye');
This is similar to the example above, except this time we have a while loop instead of a do...while loop. Again, when we get to this code, the condition is true. While loops check their condition before running the code contained within, so since false does not equal true, the code inside the while loop never runs, and the display in the console will be only Goodbye.
let counter = 0;
do {
console.log('I love JavaScript!');
counter___;
} while ( counter <= 9)
The only thing that is missing here is the counter increment. You can do this one of two ways.
counter += 1;
counter++;
Either of the above is acceptable.
Question:
Which of the following is an example of an infinite loop?
Answer:
let counter = 0;
while ( counter >= 0) {
console.log(`The counter is: ${counter}.`)
counter += 1;
}
This is because counter is initially set to 0. When it gets to the while loop, it is checking if 0 >= 0, which it is. Once it goes through the loop, the counter variable is increased by one. It then checks the condition again, and 1 >= 0. This will continue infinitely, because counter will always be >= 0.
Do you always need to use a counter or specify an exact number of times that a loop must run?
Answer: No. All you need is a condition that, at some point, evaluates to false so that the loop can end.
Consider the following console output:
1
3
5
7
9
11
13
15
Complete the code in the do...while loop to display the above output in the console.
let i = 1;
do {
console.log( #${i}
);
i += 2;
} while ( i <= 15 )
I hope this helps!
Kevin Leong
1,664 PointsKevin Leong
1,664 Pointslet counter = 0; do { console.log('I love JavaScript!'); counter___; } while ( counter <= 9) The only thing that is missing here is the counter increment. You can do this one of two ways.
counter += 1; counter++;
Either of the above is acceptable.You mentioned that you can use
counter += 1;
, I have tried this about 10 times now and it doesn't work. I think it is looking forcounter++;