Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial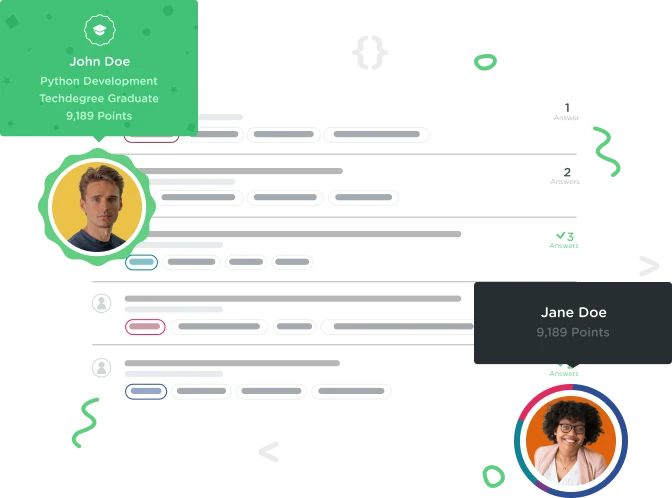

roshaan zafar
3,868 Pointsplease can you correct my code
please rectify me on the code block of method getCountOfLetter
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public int getCountOfLetter(char letter){
for( int count: tiles.toCharArray());
return getCountOfLetter;
}
public void addTile(char tile) {
tiles += tile;
}
public boolean hasTile(char tile) {
return tiles.indexOf(tile) != -1;
}
}
// This code is here for example purposes only
public class Example {
public static void main(String[] args) {
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
ScrabblePlayer player2 = new ScrabblePlayer();
player2.addTile('z');
player2.addTile('z');
player2.addTile('y');
player2.addTile('f');
player2.addTile('u');
player2.addTile('z');
int count = 0;
// This would set count to 1 because player1 has 1 'p' tile in her collection of tiles
count = player1.getCountOfLetter('p');
// This would set count to 2 because player1 has 2 'd'' tiles in her collection of tiles
count = player1.getCountOfLetter('d');
// This would set 0, because there isn't an 'a' tile in player1's tiles
count = player1.getCountOfLetter('a');
// This will return 3 because player2 has 3 'z' tiles in his collection of tiles
count = player2.getCountOfLetter('z');
// This will return 1 because player2 has 1 'f' tiles in his collection of tiles
count = player2.getCountOfLetter('f');
}
}
2 Answers
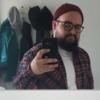
Gabe Olesen
1,606 PointsHey Roshaan,
I don't normally give full answers like below, but hopefully when I explain each line hopefully you'll understand!
So,
This task wants you to loop through each of the tiles, use an equality check, and then increment a counter if the tile and letter match.
From the above we can now see we will need the following:
- A statement that loops through each of the tiles created - This sounds like a For-Loop
- An equality check - Sounds like an if statement
- A counter that increments - We will need to declare an int variable to store the count of the tiles
OK so here public int getCountOfLetter(char letter) we've created a method that will return an int and takes an argument, we can safely assume the argument is the letter passed into the tile to make up the users tiles as it shows in the Example.java here:
ScrabblePlayer player1 = new ScrabblePlayer();
player1.addTile('d');
player1.addTile('d');
player1.addTile('p');
player1.addTile('e');
player1.addTile('l');
player1.addTile('u');
Next we have to create a variable of type int that will hold the count if the letter passed in matches the tile, so we create int count = 0; and set it to 0;.
Next, we want to create a loop that will loop through all of the users guesses and see if they are in the tiles. However we will need to use the .toCharArray() method on tiles as it's String. By doing this we're splitting up the string into an array of chars.
Now we have a loop we can now do an equality check to see if the letter passed in matches the tile(s) if TRUE we will increment else if it's false we'll just return the count at it's current state.
That's it !
public int getCountOfLetter(char letter) {
int count = 0;
for (char guess : tiles.toCharArray()) {
if (letter == guess) {
count ++;
}
}
return count;
}
I know this was a bit much but I didn't want to give the answer without a thorough explanation of each line.
Hope this helps!
~ Gabe

roshaan zafar
3,868 Pointsthank you so much Gabe