Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial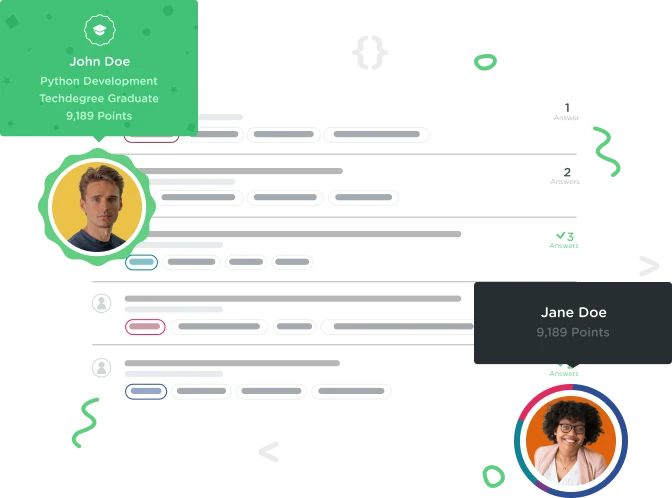
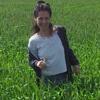
tal Shnitzer
Courses Plus Student 5,242 Pointsplease can you explain the following sentence from the module patter workshop video?
" Another thing we can do with our module pattern is import other globals to be
used within the module but with the reference, locally within the module. " what does it mean: "globals"? "reference, locally"?
at 4:56
2 Answers

Joseph Wasden
20,407 PointsNote: I think this is the workshop you are talking about.
The Module Pattern in JavaScript https://teamtreehouse.com/library/the-module-pattern-in-javascript-2
In the future, you will get more answers by including a link to the video in question. the best way to do this is to look for the Get Help button on the video page.
There was definitely a lot of information packed into that sentence. It may be helpful to study up on some of these terms. I've included some additional reading links at the end of this answer that helped me grasp what was being explained here.
The module being demonstrated is formed using an Immediately-invoked function expression, or IFFE. This looks something like this.
(function () {
/* Code goes here */
})()
notice there are two places to input arguements. We'll call them position1 and position2.
(function (position1) {
/* Code goes here */
})(postion2)
position2 is where we take some reference that is within the global scope and pass in the reference name to the module. (we'll talk about position1 in a second.)
So, lets imagine that our module is linked to an HTML page of our app. That HTML page loads both the underscore library and our module.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Our APP</title>
<!-- loads first underscore, then our module -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.8.3/underscore-min.js"></script>
<script src="ourModule.js"></script>
</head>
<body>
<!-- stuff goes here -->
</body>
</html>
The order that underscore and our module are loaded into the HTML file are important. Because underscore is loaded before our module, our module has access to the default global reference to the underscore library.
When the underscore library is loaded to the webpage, it uses the default reference of an underscore character, or _. Lets say we want to use underscore in our module. We need to pass the global reference of _ into our module at position2. We will also go ahead and give underscore a custom local reference, or the reference we will use for the underscore library inside our module, changing from the global of _ to underscore. we do that by passing the new reference name, underscore, to the module at position1.
(function (underscore) {
/* correct local reference to Underscore.js */
underscore.map([1, 2, 3], function(num){ return num * 3; });
/* incorrect local reference Underscore.js */
_.map([1, 2, 3], function(num){ return num * 3; });
})(_)
What has this accomplished? We have successfully imported the underscore library into the module, and we can now reference the underscore library inside our module. The underscore library will be referenced using the underscore reference instead of the _ reference. This reference is considered local, because it only applies to the code within our module. Remember how we said to imagine our module and the underscore library were both called from some HTML file? well, any JavaScript on that HTML page would still reference underscore using the default _ reference, because that is the default global reference for that library. code written there would only have access to the global reference, not the local reference we specified in our module.
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<meta http-equiv="X-UA-Compatible" content="ie=edge">
<title>Our APP</title>
<!-- loads first underscore, then our module -->
<script src="https://cdnjs.cloudflare.com/ajax/libs/underscore.js/1.8.3/underscore-min.js"></script>
<script src="ourModule.js"></script>
</head>
<body>
<script>
/* correct underscore reference, since we aren't in our module and are using the default global reference*/
_.map([1, 2, 3], function(num){ return num * 3; });
</script>
</body>
</html>
So why would we want to rename the global reference to a custom local one? at 5:24 in the video, the instructor explains more about that.
Hope that helps clear some things up. Keep asking questions if not.
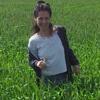
tal Shnitzer
Courses Plus Student 5,242 Pointswow! that is some good explanation, thanks!
so if I code within a module can I use global variables at all? do I need to import global variables if I want to use it like in the _ example?

Joseph Wasden
20,407 PointsIn the HTML example I provided, you should be able to access Underscore just fine in your module without importing it into the module. The reference for it would just be the default "_" character.