Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial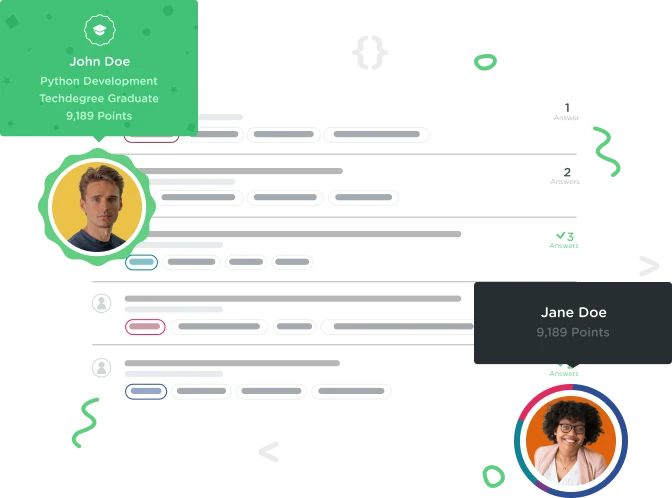

Federico Isabello
1,637 PointsPlease, can you help me realize were is the problem here? Thanks!
class Panda: species = 'Ailuropoda melanoleuca' food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
return ("Bao Bao eats {}.".format(food))
class Panda:
species = 'Ailuropoda melanoleuca'
food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
return ("Bao Bao eats {}.".format(food))
5 Answers
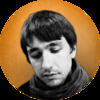
Kristjan Vingel
7,992 PointsYou are returning "Bao Bao" but you need to return the name attribute. Don't forget to add it to the format argument as well.
A class is a blueprint for creating objects. What if we want to create another Panda using that class and that panda will have a different name - like "Mao Mao" for example. Then the eat method would not make sense as it will also say: "Bao Bao eats ...". We want it to be re-usable so that we can create different pandas with different names and when we call the eat method on any one of those, it'll say the correct name - the name we initialized the object with :)

Federico Isabello
1,637 PointsOk, and where and how should I declare the name Bao Bao?

Federico Isabello
1,637 PointsThanks for your help! This is the code I wrote for now
class Panda: species = 'Ailuropoda melanoleuca' food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
name = 'Bao Bao'
return (f*"{name} eats {food}.")
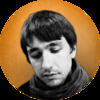
Kristjan Vingel
7,992 PointsYou don't need to declare the "Bao Bao" name in the eat method. If I understand correctly a new panda object is created under the hood by Treehouse workspace something along the line of this:
panda1 = Panda("Bao Bao", 10)
..and then they use the eat method on this new panda object - like that:
panda1.eat()
The name and age is passed in to the constructor / initializer when panda1 is initialized. This is is why we declare the name and age in the def init(self, name, age) thingie - then we can use these values in the methods (like the eat method).
With the class, we can create as many pandas as we want:
panda1 = Panda("Bao Bao", 10)
panda2 = Panda("Bao Mao", 6)
panda3 = Panda("Mao Mao", 4)
panda4 = Panda("Yao Yao", 3)
And as I mentioned - we can call the eat() method on any one of those, and it'll say the correct name.
But I realize the confusing part is that we don't initialize the object ourselves - we just have to create the blueprint (class), so that the Treehouse machine is able to use that class to initialize pandas and check if we know how to declare a class in Python.
Ps! I hope I did not mess anything up with Python's OOP. If i did, please someone smarter correct me.

Federico Isabello
1,637 PointsYes I understand your point, but I still can get it right! This is what Iยดve done
class Panda: species = 'Ailuropoda melanoleuca' food = 'bamboo'
def __init__(self, name, age):
self.is_hungry = True
self.name = name
self.age = age
def eat(self):
self.is_hungry = False
return (f*"{name} eats {food}.")
Panda.eat('Bao, Bao')
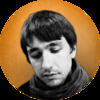