Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial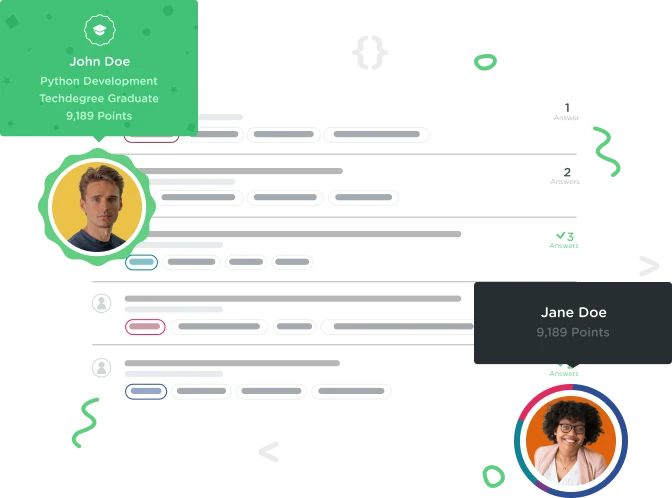

Michael North
2,561 PointsPlease check
Can somone please explain to me why my code doesnt work. Thank you
namespace Treehouse.CodeChallenges
{
class FrogStats
{
public static double GetAverageTongueLength(Frog[] frogs)
{
foreach(Frog frog in frogs)
{
int totalLengths = frog.TongueLength + totalLengths;
double tongueAverage = totalLengths / frogs.Length;
return tongueAverage;
}
}
}
}
namespace Treehouse.CodeChallenges
{
public class Frog
{
public int TongueLength { get; }
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
}
}
1 Answer

Jon Wood
9,884 PointsI see a couple of things that may cause it not to compile:
- You're using the
totalLengths
variable before it gets initialized. You can change this line:int totalLengths = frog.TongueLength + totalLengths;
to something like this:int totalLengths = 0; totalLengths = frog.TongueLength + totalLengths;
- You're returning inside of the
foreach
loop. You'll have to move it outside of the loop or else the compiler will yell about the method where not all paths have a return value. - For the algorithm itself, you're dividing while in the loop, as well. You just need the loop to get the sum of lengths and outside of it do the division.
You're doing great, though! Keep up the awesome job!