Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial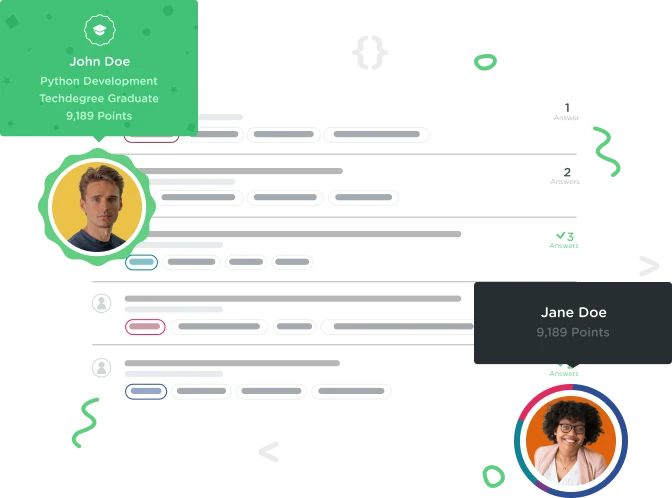
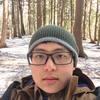
Pei Sheng Tan
Python Web Development Techdegree Student 2,600 PointsPlease check my code for redundancy? I expanded the script to also check for valid/invalid answers.
Hi! So I decided it would be fun to try and expand the script so that it will only take "yes", "no", "y", "n" as inputs. The code will loop until user provide a valid input to proceed to the next loop.
#This function checks if user gives a valid response
def convert_answer(text):
answer = text.lower()
if (answer == "y") or (answer == "yes"):
return("yes")
if (answer == "n") or (answer == "no"):
return("no")
else:
return("NA")
#prompts user for inputs
name = str(input("What's your name?: "))
prompt = str(input("{}, do you understand Python while loops?\n(Enter yes/no): ".format(name)))
#Using the previously defined function to check the answers
understanding = convert_answer(prompt)
# This while loop keeps going until understanding == "yes"
while understanding != "yes":
while understanding == "NA":
print("Your answer is invalid, please try again")
understanding = convert_answer(str(input("{}, do you understand Python while loops?\n(Enter yes/no): ".format(name))))
if understanding == "no":
print("Ok, {}, while loops in Python repeat as long as a certain boolean condition is met".format(name))
understanding = convert_answer(input("{}, do you understand while loops in Python now?\n(yes/no)".format(name)))
print("That's great, {}. I'm pleased that you understand while loops now. That was getting repetitive.".format(name))
So, my question: is there anyway to reduce the amount of code? Any redundancy?
Afaik, the code works as intended. So if you wanted to do the same thing I hope this helps! Took me an hour of trial and error tbh.
1 Answer
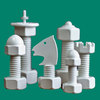
Steven Parker
229,785 PointsThis code looks pretty DRY already. I don't see any redundancies.
You can make it a tiny bit more compact by using f-strings instead of "format". And you can eliminate the "else:" in "convert_answer" since all the other paths end with a "return".
Example of f-string:
print("Hello, {}".format(name)) # using "format"
print(f"Hello, {name}") # using f-string