Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial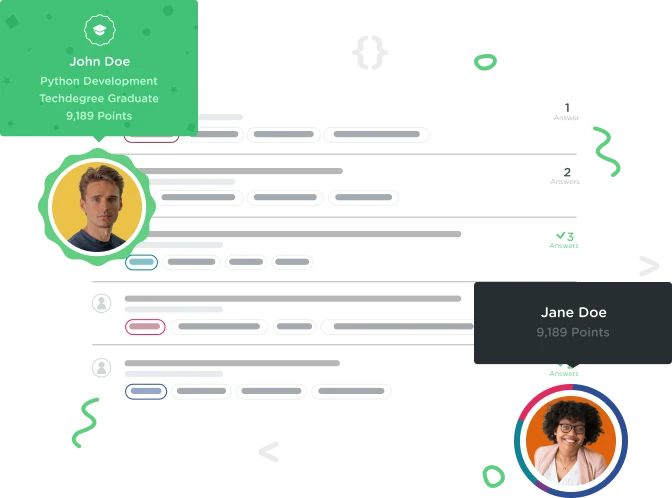

Matt Clarke
6,712 PointsPlease could someone help me complete this task?
Not getting any compiler errors but still cant figure out how to get it working.
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(distanceToFly)
{
bool EatFly = distanceToFly <= TongueLength && distanceToFly >= 0;
return EatFly;
}
}
}
3 Answers
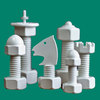
Steven Parker
230,274 Points
You just forgot to declare the type of argument that EatFly takes.
Other than that, good job!
Optionally, since you compute a boolean return value, you could just return it directly (and you can simplify the test a bit):
public bool EatFly(int distanceToFly)
{
return distanceToFly <= TongueLength;
}
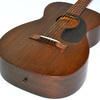
Charles Williams
2,680 PointsYou forgot to specify type of distanceToFly (int). Also it helps to separate boolean logic with paren to make it easier to read:
namespace Treehouse.CodeChallenges
{
class Frog
{
public readonly int TongueLength;
public Frog(int tongueLength)
{
TongueLength = tongueLength;
}
public bool EatFly(int distanceToFly)
{
bool EatFly = (distanceToFly <= TongueLength) && (distanceToFly >= 0);
return EatFly;
}
}
}

Cindy Lea
Courses Plus Student 6,497 PointsIt says you didnt create a parameter to method distanceToFly. Im getting an error.