Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial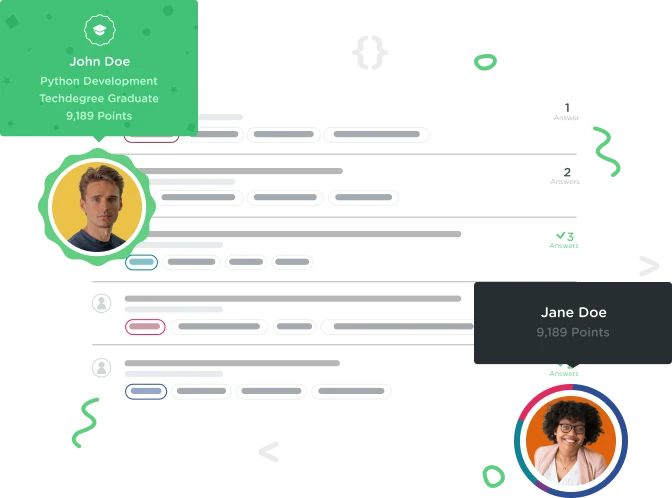
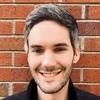
Jesse Vorvick
6,047 PointsPlease critique my code / Feedback
I was amazed at how hard this challenge seemed to be compared to the other ones. I worked on and off for about two weeks trying to push myself to figure it out without looking at the answer. I even did this entire track over from the beginning to review. I thought it was pretty clear during the previous video that the end goal was to make the program work/look the same way as he briefly showed it. Imagine how peeved I was to finally get it done and realize all I was supposed to do was... well what was shown in this solution video.
Well nothing to do now but move on. I hope I didn't get too off track with my take on the code, but please review it and tell me what you think. It seems to work the way (I thought) it was shown to be supposed to work in the previous video, but feedback would be appreciated for how I can improve.
var quiz = [
['Who was the third president of the United States?', 'Thomas Jefferson'],
['What is the correct term for a group of whales?', 'pod'],
['What was Einstein\'s first name?', 'Albert']
];
function print(message) {
document.write(message);
}
function printQuiz ( questions ) {
var score = 0;
var listHTMLcorrect = '<h2>' + 'You got these questions correct:' + '</h2>' + '<ol>';
var listHTMLwrong = '<h2>' + 'You got these questions wrong:' + '</h2>' + '<ol>';
for (i = 0; i < questions.length; i += 1) {
var guess = prompt( questions[i][0] );
if (guess.toLowerCase() === questions[i][1].toLowerCase()) {
score += 1;
listHTMLcorrect += '<li>' + quiz[i][0] + '</li>';
} else {
listHTMLwrong += '<li>' + quiz[i][0] + '</li>';
}
}
listHTMLcorrect += '</ol>';
listHTMLwrong += '</ol>';
document.write('You got ' + score + ' question(s) right');
print(listHTMLcorrect);
print(listHTMLwrong);
}
printQuiz(quiz);
2 Answers

Matthias Margot
7,236 PointsHi there Jesse,
From the point of view of functionality your code seems to be quite impeccable :) I don't have the context of the task you were supposed to complete, but I can tell you about general patterns which could help you improve the way you write JavaScript.
document.write
is considered an anti-pattern in browser-js why?maybe dividing the QnAs into
correct
andwrong
could be done separately before generating your HTML output (e.g. two arrays holding the right and wrong answers respectively), might make it a little easier to understand what's going on since right now you're doing 4 different operations on each iteration-step:
- prompting
- checking for correct answers
- incrementing the score
- sorting questions by right & wrong
- generating the HTML for it
This would also allow you not to start your html-string before the loop and end it after the loop creating more clearly defined areas in your code where something is going on.
- You might want to switch your quiz data-structure to something that has a little more semantic readability value because
quiz[i][0] / quiz[i][1]
doesn't say much on its own compared to
quiz[i].question / quiz[i].answer
You're forcing a reader of your code to search for the place you defined your data-structure at. This adds more code and increases verbosity a little bit but I'd say that it's worth it worth the increased ease of understanding that you get from it.
- on line
15
, where you're setting up your loop, this bit of code:
for (i = 0; ...
actually creates a variable called i
in the global scope (sorry for that word scope, this is a more advanced js topic not sure if you're familiar with it). You basically want to change that to:
for (var i = 0; ...
This is a wider topic, if you're interested in learning why that is you can check out this link: Javascript: Why you should use the βvarβ keyword
Hope I could help with something :)

KRIS NIKOLAISEN
54,972 PointsYou could run it through JSLint