Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial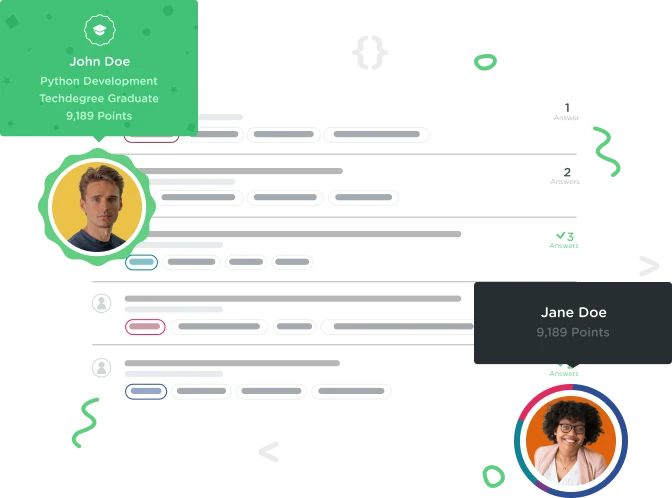

lakhwinder singh
Courses Plus Student 165 Pointsplease explain me this i dont get it
please expalin this coding
package com.teamtreehouse.vending;
import org.junit.Test;
import static org.junit.Assert.*;
public class CreditorTest {
@Test
public void addingFundsIncrementsAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(25);
creditor.addFunds(25);
assertEquals(50, creditor.getAvailableFunds());
}
@Test
public void refundingReturnsAllAvailableFunds() throws Exception {
Creditor creditor = new Creditor();
creditor.addFunds(10);
int refund = creditor.refund();
assertEquals(10, refund);
assertEquals(0, creditor.getAvailableFunds());
}
}
package com.teamtreehouse.vending;
public class Creditor {
private int funds;
public Creditor() {
funds = 0;
}
public void addFunds(int money) {
funds += money;
}
public void deduct(int money) throws NotEnoughFundsException {
if (money > funds) {
throw new NotEnoughFundsException();
}
funds -= money;
}
public int refund() {
int refund = funds;
funds = 0;
return refund;
}
public int getAvailableFunds() {
return funds;
}
}
1 Answer
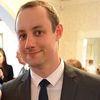
Benjamin Doyle
9,603 PointsWhich part are you having an issue with?
CreditorTest.java contains some junit tests, which test code so that if you continue working on Creditor.java, and the test breaks you know where and how (or a general idea on how) to fix it.
and Creditor.java is the code you are working on.
It is all a part of Test Driven Development, which you can find more info on TT or here: https://en.wikipedia.org/wiki/Test-driven_development