Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial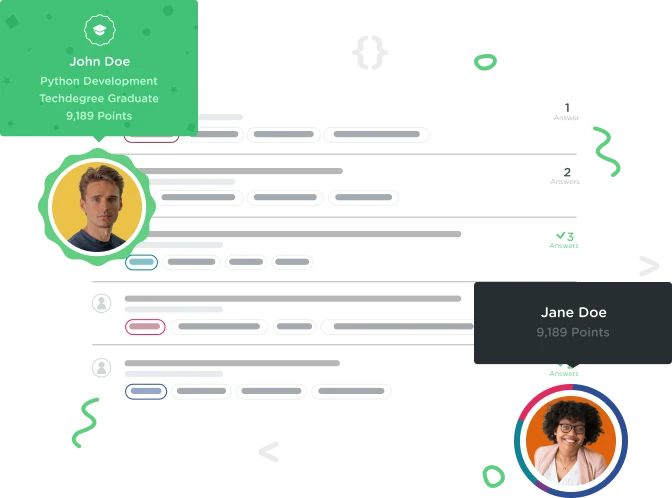
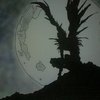
Lucas Santos
19,315 PointsPlease explain prompter.java. Do not understand him passing the Game object into the Prompter object.
Ok so here is the code:
Prompter.java
import java.io.Console;
//class
public class Prompter {
private Game mGame;
//constructor
public Prompter(Game game){
mGame = game;
}
public boolean promptForGuess(){
Console.console = System.console();
String guessAsString - console.readLine("Enter a letter: ");
char guess = guessAsString.charAt(0);
return mGame.applyGuess(guess);
}
}
Hangman.java
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter = prompter = new Prompter(game);
if(isHit){
System.out.println("We got a hit!");
} else {
System.out.println("Whoops that was a miss");
}
}
}
So I really do not understand in Prompter.java this part:
//class
public class Prompter {
private Game mGame;
//constructor
public Prompter(Game game){
mGame = game;
}
I get what is being passed but I do not understand. It looks like he first called a variable of mGame and said it was an object of Game. Then in the constructor he called another variable of the Game object and set it to the variable game. Then he set that variable to mGame.
Now why did he do that? please explain. Iv already went thought this course and this is the only part that I do not understand. I see him using the mGames through out the Prompter along with methods of Game.java which means he some how shared the methods of another class. I think this is how but I do not understand this part. Can someone please explain how this works.
I also do not understand in Hangman.java why he did
Prompter = prompter = new Prompter(game);
I understand Prompter is the class assigned to the instance variable of prompter as a new object of the class Prompter but why did he pass the variable "game" in the parameter?
I can see all this is to share methods of different classes but I do not understand how this is working or why.
2 Answers

Raymond Wach
7,961 PointsYes, in the line:
Prompter prompter = new Prompter(game);
The game
variable is a reference to the Game
object that was created on the previous line:
Game game = new Game("treehouse");
What's going on in these lines can be broken down in to two pieces, a declaration and an initialization. In other words, we can rewrite Prompter prompter = new Prompter(game);
as follows:
Prompter prompter; // Declare an instance of the class Prompter that is named prompter.
prompter = new Prompter(game); // Initialize the variable prompter to be a reference to the specific
// instance of Prompter returned by the Prompter constructor.
// More on 'game' below.
Because Java is a statically-typed, compiled language you need to declare the type of your variables before you use them. Optionally, at the class level, you can provide a scope keyword: private
, protected
, or public
, to control the visibility of the thing you are declaring. Functions are declared with a slightly different format where you specify the return type first, the name of the function, and then the parameter list, which is itself a sequence of declarations that will be used inside the function block to define the function's implementation. Note, in addition to the visibility modifiers there are other keywords which can precede a declaration such as static
and final
, but that doesn't have much bearing in this conversation.
To recap:
Given the class declaration (which Java requires to be in it's own file named Prompter.java
):
class Prompter {
private Game mGame;
public Prompter(Game game){
mGame = game;
}
}
What this is saying is that there is a class named Prompter
with a private variable of type Game
named mGame
and a single constructor for objects of type Prompter
that takes as an argument a variable of the type Game
named game
and inside the constructor it sets the value of the instance's private variable mGame
to be the value of game
. (I'm intentionally glossing over the reference vs. value discussion.)
Now inside the Hangman
class we have a main
method as follows:
public class Hangman {
public static void main(String[] args) {
Game game = new Game("treehouse");
Prompter = prompter = new Prompter(game);
// Rest of method elided for brevity.
}
}
The body of the main
method is the entry point of your program so this is the first code that will run, starting from the first line of main
executing each statement consecutively. So the first line declares and initializes the variable game
(what is its type?) and the second line uses the variable game
to initialize the variable prompter
that it just declared to have the type Prompter
.

Raymond Wach
7,961 PointsI haven't watched this video so I can't answer your question completely, but I can try to explain some of the parts that seem to be confusing you. The difficulty I think you are having (please correct me if I'm wrong) is due to the overlapping of some of the names assigned and the overloading of some words such as "prompt" and "game".
So at a high level, everywhere you see the word new
in Java you are creating a new instance of a class, or instantiating a new instance of that class. When this happens, the constructor for that class is run with whatever arguments are required. The constructor is denoted in a class by declaring a public method with the same name as the class and without a return value. Its return value is implicit: a new instance of the class. So the Prompter
class has one constructor with the parameter list: (Game game)
. In other words, in order to create a new instance of the Prompter
class you need to give it an instance of Game
to work with and inside the constructor, it will refer to that instance as game
. In the particular case of this constructor, all it does is to assign the private instance member (more specifically property, in this case) mGame
to game
.
So in the main
method of Hangman
, you first create a Game
, passing in the literal string argument "treehouse"
, then you create a new Prompter
that keeps a reference to that Game
which it privately refers to as mGame
.
I hope that my explanation is helpful and I am happy to clarify any further confusion you have. My advice would be to review the scoping rules for Java and to focus on the distinctions between classes and instances, arguments and parameters. Often, the only way to know what a particular name is, is by knowing the context in which that name is declared.
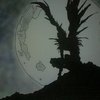
Lucas Santos
19,315 PointsOk I get what you're saying but there is still some confusion. So to let you know just incase to clarify on my end as well Game is a class in Game.java a file I did NOT include on here because I didn't think it was relevant. Now in Game.java it has a class with a constructor and couple of methods (Like any class would). But now my question is how did he use methods from Game.java in Prompter.java. I think I already know the answer but I really want to clarify because I am un sure. So let me break this down:
//class
public class Prompter {
private Game mGame;
//constructor
public Prompter(Game game){
mGame = game;
}
on top where you see private Game mGame
the first Game is refering to the class in Game.java named Game correct?
Now to the next question in Hangman.java:
public class Hangman {
public static void main(String[] args) {
// Enter amazing code here:
Game game = new Game("treehouse");
Prompter = prompter = new Prompter(game);
in this specific line:
//class __ instance variable__ = new __ class(parameter)
Prompter prompter = new Prompter(game);
the game parameter that is in Prompter(game)
is that a random variable or is that the actual instance variable that was created above in this line:
//class __ instance variable__ = new __ class(parameter)
Game game = new Game("treehouse");
Meaning was the parameter that is passed into the Prompter(game) the the object of Game class that was created above that line?
I got a good understanding on what you wrote but if you can clarify this then id fully understand whats going on here.
Lucas Santos
19,315 PointsLucas Santos
19,315 PointsAhh I see now ok the part that confused me the most was in prompted.Java in the fields where it said Game mGame; the first Game references to the class(type) Game.. Thanks a ton Raymond! Really appreciate you taking the time to help me understand this.