Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial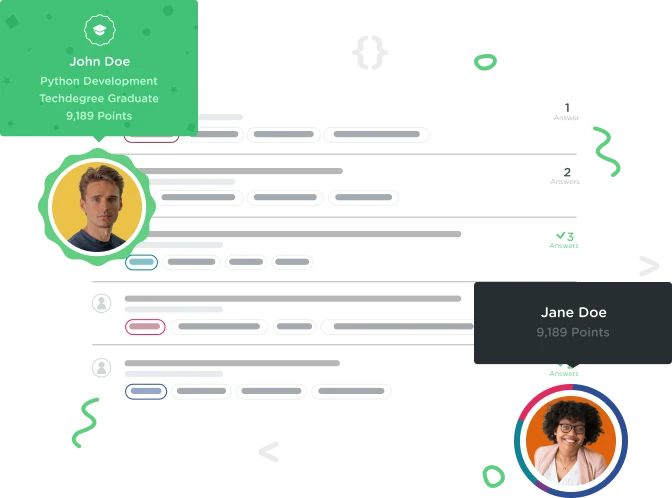
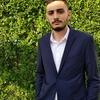
Vlad Tanasie
7,201 PointsPlease explain the last example with the CPS
Why is the result 65 and what does the (n)
argument in each callback refer to? The callback or the numbers that are given as arguments?
It is melting my non-mathematical brain.
2 Answers
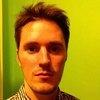
Brendan Whiting
Front End Web Development Techdegree Graduate 84,735 Pointsfunction add(x, y, callback) {
callback(x + y)
}
function subtract(x, y, callback) {
callback(x - y);
}
function multiply(x, y, callback) {
callback(x * y);
}
function calculate(x, callback) {
callback(x);
}
calculate(5, (n) => {
add(n, 10, (n) => {
subtract(n, 2, (n) => {
multiply(n, 5, (n) => {
console.log(n); // 65
});
});
});
});
On line 13 we're defining a function called calculate
.
It has two parameters, x
and callback
; x
will be a
number and callback
will be a function. Inside of the
body of calculate
, we're calling the function callback
passing
in the value of x
as the first argument for that function.
(I should note here that I'm using the terms parameter and argument to mean different things, as explained here):
The later on, on line 17, we call the calculate function. 5 is passed in as the first argument.
And a new anonymous arrow function is passed in as the 2nd argument, which is defining the
function in place. In the definition of that function, it takes a parameter n
. In this example, at runtime, n
will be 5,
because that's what we're passing in as the 1st argument to calculate
on line 17, and if we flip back to the function definition
on line 13, that number 5 will then be x
and then on line 14 x
gets passed in to the callback. So 5 is x
and x
is n
when things kick off here.
It's not that easy to follow. It took me a minute to untangle it. My preference is not to use this kind of 'continuation-passing style', which is also referred to less positively as "callback hell" or "pyramid of doom". Here's an alternative version without anonymous functions, defining those functions separately. It's still tricky to follow but at least it's an alternative version of the same thing that may help you connect some dots. (I also made all the functions into arrow functions for consistency)
const add = (x, y, callback) => {
callback(x + y)
}
const subtract = (x, y, callback) => {
callback(x - y);
}
const multiply = (x, y, callback) => {
callback(x * y);
}
const calculate = (x, callback) => {
callback(x);
}
/***** calbacks *****/
const onMultiply = (n) => {
console.log(n); // 65
}
const onSubtract = (n) => {
multiply(n, 5, onMultiply);
}
const onAdd = (n) => {
subtract(n, 2, onSubtract);
}
const composedCalculator = (n) => {
add(n, 10, onAdd);
}
calculate(5, composedCalculator);
It's kind of an abstract example. This isn't the ideal way of doing math in either case, but there are lots of other operations, like fetching data or handling click events, where you need to deal with a string of operations and pass functions as arguments to other functions as callbacks.
Let me know if you have any more questions.

gerardo keys
14,292 PointsJust a tip for anyone having trouble learning this like me. Go to http://pythontutor.com/visualize.html#mode=edit which is a visualizer for code, you can also do javascript. Copy + paste this code in there then you can visualize the execution step by step. It shows graphics of each function and shows step by step where in the program you are. You can fast forward or go back as well. This site has helped me immensely learn difficult topics by running it step by step and visualizing each step. Hope it helps!
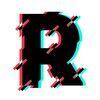
r22
6,809 PointsGerardo Keys thank you for that tool. Truly invaluable add!
Vlad Tanasie
7,201 PointsVlad Tanasie
7,201 PointsThanks for the clarification, still hard to digest but the second example is much more understandable, and thanks for the link with parameters and arguments.