Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial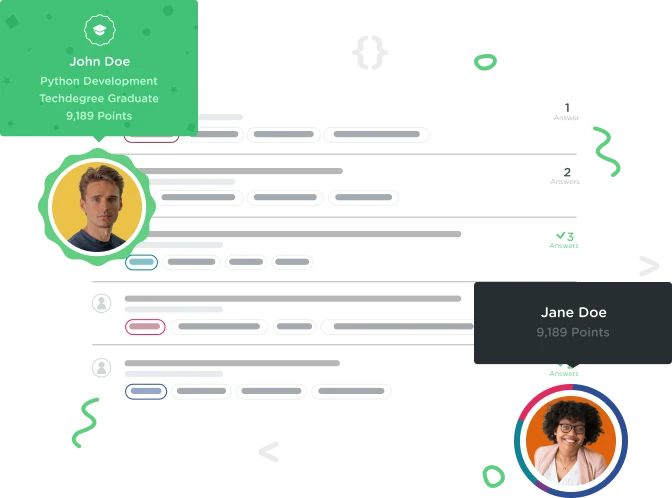

Kshatriiya .
1,464 PointsPlease explain the relationship between sort() and compareTo()
Hello, I'm banging my head on the table trying to comprehend a few things in this topic.
Here is my current understanding, first, we turned Tweet class into a Comparable interface so that we can override the interface's compareTo() method with our own.
Now here's when I got really confused:
in the Example we created two Tweets objects, treet and secondTreet:
Treet treet = new Treet("hello treehouse", "Josh", new Date(1929491489L)"
Treet secondTreet = new Treet("hello again", "John", new Date(1928194812L)"
Now we create a Treet array with the above two treets:
Treet[] treets = {treet, secondTreet]; //This make treets an array of Treet class containing two Treet class objects.
then when you make
Arrays.sort(treets) //Arrays.sort() takes Treet class object treets as its parameter.
From reading around the forum this treets is put into compareTo(treets) as the sort method calls compareTo().
Then why do we have
compareTo(Object obj)
instead of
compareTo(Tweet twt)?
Then you have
if(equals(other)) // what exactly is equals to other here? Also is other == {treet, secondTreet}?
in the same manner what does this mean?
mCreationDate.compareTo(other.mCreationDate) //how does this return anything as mCreationDate is an empty declaration while other.mCreationDate contains creationDates of {treet, SecondTreet} ?
Because we are comparing treet to secondTreet instead of if(equals(other)) shouldn't it be something like if(treet.equals(secondTreet) {} or treet.mCreationDate.compareTo(secondtreet.mCreationDate)?
Craig Dennis I know these are many questions but if someone could guide me through this, you'd have my upmost gratitude.
Thank you.
2 Answers

Craig Dennis
Treehouse TeacherHi Kshatriiya . !
Arrays.sort
takes each item in the array and compares it to the next item in the array. *
So if we had
// String implements the Comparable interface and therefore has defined a compareTo method.
String first = "Cherry";
String second = "Banana";
String third = "Apple";
String[] fruits = {first, second, third};
Array.sort(fruits);
// What happens in Arrays.sort is can be imagined something like this....
int result = first.compareTo(second);
// If the comparison of the first is larger than second ...
if (result < 0) {
// ... then swap the order
fruits[0] = second;
fruits[1] = first;
}
// And then it continues through...
result = second.compareTo(third);
That make more sense? Arrays.sort
only works on arrays of items that implement the Comparable interface, so it knows it can rely on using compareTo method which can be used to compare two objects together, the one it is processing, and another one.
I think you must be missing or haven't gotten to the part about generics (or parameterized types). You will soon see that we change to implement using Comparable
< Treet > this will require a method whose signature is what you are looking for, a compareTo(Treet treet)
instead of object.
I believe the other
you are talking about is because we did a type-cast like this:
public int compareTo(Object obj) {
// Cast the object into a treet
Treet other = (Treet) obj;
}
And when we introduce the generic I believe I just switched the signature and the parameter name to other so the code still worked:
public int compareTo(Treet other) { // See how here the name is other
Did that help clear things up?
- This is simplified a bit. The sort algorithm is more complex

Kshatriiya .
1,464 PointsSorry for being so incessant, could you elaborate on that one a little bit please? :) fruits is an array containing fruitOne and fruitTwo but when it is downcasted to tempFruits it is no longer an array?
Also if i do
if(equals(tempFruit));
Does it mean:?
if(fruitOne.equals(fruitTwo))

Craig Dennis
Treehouse TeacherNo need to apologize! I thought you might still be hung up there ;) Be incessant, it's a sign of a good developer!
Yes equals is a method on the instance that is being called, so it's more like
if (this.equals(fruitTwo))
Does that make sense? The array has items that are comparable because each instance has a compareTo
method. So the static
sort method on Arrays
knows that it can call on each instance the compareTo
method and it does just that.
When the downcasting is happening it's happening on an Object
, a single instance, not an array, that is passed into the a different instance's compareTo
method.
That help? I think it's around instance methods where your confusion is happening. compareTo
is a method that belongs to each object in that array. That compareTo
method is used to compare two objects, the existing one, and any other object that matches the method signature (depending what video you are on, that's Object
first, and Treet
after we use the Generic (parameterized type) version of the Comparable
interface)
Stay incessant! Thanks for asking your questions, please let me know if that clears things up for you!
Kshatriiya .
1,464 PointsKshatriiya .
1,464 Pointshi Craig, thank you very much for personally coming to address this for me. It does help a lot now.
So basically, if I do
Then
Then override compareTo()
Does the last line roughly translate to:
return "Apple".compareTo("Orange");
So Array.sort() takes the first element and compare it to second element when it is overriden with the following:
return mName.compareTo(tempFruits.mName);
Again thank you very much for your help, after reading through what you wrote, I'm starting to see how this process works behind the scene.
Craig Dennis
Treehouse TeacherCraig Dennis
Treehouse TeacherLooks like you got it! One quick thing just for clarity, the
tmpFruits
should be singulartmpFruit
. Just making sure you realize it's not an array.