Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial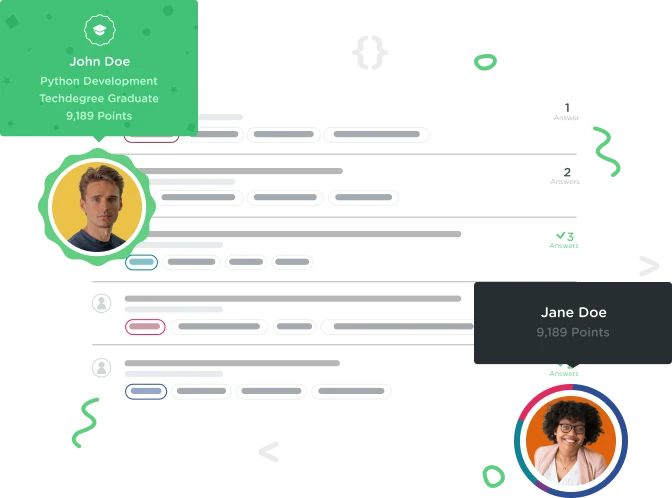
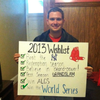
Jimmy Seeber
12,809 PointsPlease explain why my solution is marked incorrect. if(tiles.indexOf(tile) == -1) return false; return true;
The equivalent is also:
if(tiles.indexOf(tile) == -1){
return false;
}else{
return true;
}
which is also marked incorrect.
public class ScrabblePlayer {
// A String representing all of the tiles that this player has
private String tiles;
public ScrabblePlayer() {
tiles = "";
}
public String getTiles() {
return tiles;
}
public void addTile(char tile) {
tiles += tile;
// TODO: Add the tile to tiles
}
public boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
if(tiles.indexOf(tile) == -1){
return false;
}else{
return true;
}
}
}
1 Answer

Abu Muhariba
1,140 Pointspublic boolean hasTile(char tile) {
// TODO: Determine if user has the tile passed in
if(tiles.indexOf(tile) == -1){
return false;
}else{
return true;
}
The expression tiles.indexOf(tile) == -1
is a boolean expression. Therefore it returns a true or false statement. This means the if statement is redundant and we can simply return (tiles.indexOf(tile) == -1);
i.e. return a true or false
The -1 also needs to be changed to a 1 as -1 indicates the tile
is not present in tiles
.
Your solution is correct per se but that's not what this specific exercises is looking for. Thus if you were to run the exact code above Team Treehouse would state: While you could definitely solve this using an if statement, try returning the result of the expression.
[MOD: edited code block & changed comment to answer - srh]
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsI think you can test whether
tiles.indexOf(tile)
is either not -1 or is zero or more. That's the same test.You don't want to use the index of 1 as the indices are zero-based. The
indexOf
method returns the position in the string, or -1 if the character isn't present. Iftile
is the first character intiles
, it'll return zero. You are correct that the result of this expression just needs returning. Redundant tests are easy to add!So, there's two tests that should work here:
I hope that helps.
Steve.