Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial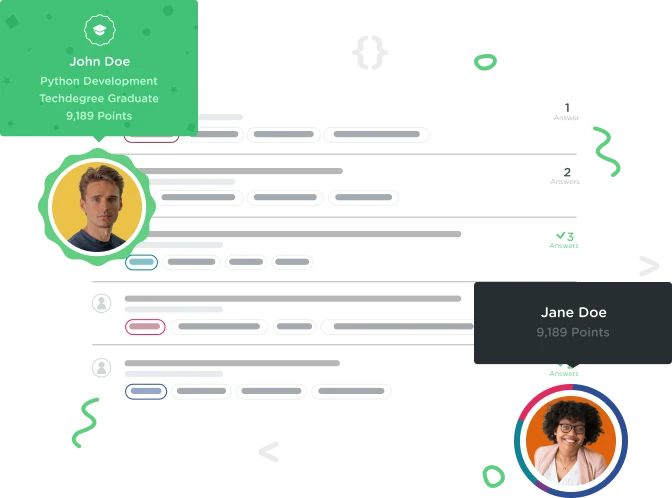

Naveed Sarwar
Courses Plus Student 1,081 PointsPlease, find the problem in this program.
I have been trying to solve this exercise for more than an hour. For some reason, the compiler says:
Expected 0 but getting 1.
Please, check if there is any error in my program. Your help would be really appreciated. Thanks in advance.
public class ScrabblePlayer {
private String mHand;
private int mCount;
public ScrabblePlayer() {
mHand = "";
mCount = 0;
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile) {
for (char letter : mHand.toCharArray()) {
if (tile == letter) {
mCount++;
}
}
return mCount;
}
}
2 Answers

Katherine Marino
3,286 PointsYour function will work as written ... exactly one time. You have your mCount variable declared as a property of the ScrabblePlayer, so think about what will happen if you call the getTileCount() function twice. (This is probably how the challenge is being evaluated, because again, it will work correctly once.) The first time it will count how many matching tiles there are, and increment mCount by that much. So say you have two matches, after getTileCount() is run, mCount will be equal to 2.
Now when you run the function again on a new letter, the function starts but mCount is already = 2. So you will actually be adding however many matches occur in this run of the function to the amount of matches in the last function run.
You can fix this by simply adding the line
mCount = 0;
to the start of the function. However, there really is no reason to have mCount declared outside the scope of the function, so it is preferable to just declare and initialize mCount inside the function. Look at my solution below:
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public int getTileCount(char tile) {
int mCount = 0;
for (char letter : mHand.toCharArray()) {
if (tile == letter) {
mCount++;
}
}
return mCount;
}
}
Hope that helps, let me know if you don't understand anything!

Naveed Sarwar
Courses Plus Student 1,081 PointsThank you, again. Have a nice day.

Katherine Marino
3,286 PointsGlad I could help, you too! :)
Naveed Sarwar
Courses Plus Student 1,081 PointsNaveed Sarwar
Courses Plus Student 1,081 PointsHmmm... That's interesting. Got it. One more thing I have to ask would be as we are declaring a normal variable in this case, then according to naming conventions, I should remove the letter 'm' in the beginning of this variable, am I correct in assuming that?
Thank you for your fast and correct response.
Katherine Marino
3,286 PointsKatherine Marino
3,286 PointsGood point, you are correct! :) If you're following the naming convention of mName for member variables then I believe it would indeed be preferable to just use "count" now.
Keep in mind that a lot of naming conventions come down to personal preference when developing your own applications. There are some conventions that are widely used and expected (such as ALL_CAPS for public static fields), and it might really confuse other developers who might help with your project if you don't use them... But I don't always see this particular convention in Java (and when I do, I think it's a lot common for Android apps). In this course they might expect you to follow certain conventions, but when writing your own programs it's really up to you and whether you feel like it helps you keep your variables straight! Just as long as you know what it means when you do come across it. :)