Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial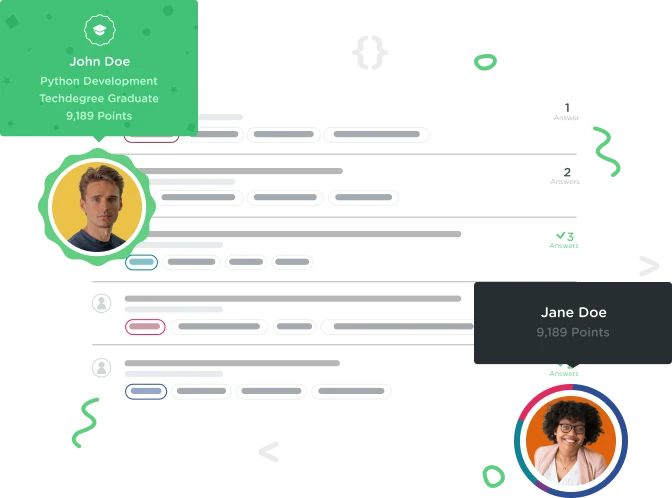

JASON PETERSEN
14,266 PointsPlease give feedback on this script
I would love some feedback on this script. What did I do well, where could I make improvements?
Some thoughts I had: Add a try statement have the ask input be a function
players = []
splayers = ''
ask = input('Would you like to add a player to the list? (Y/N) ')
while ask.lower() == 'y':
name = input('Enter the name of the player to add to the team: ')
players.append(name)
ask = input('Would you like to add a player to the list? (Y/N) ')
print('\nThere are {} players on the team.'.format(len(players)))
for i in range(len(players)):
print('Player {}:'.format(i+1), players[i])
keeper = int(input('\nPlease select the goal keeper by selecting the player number. (1-{}) '.format(len(players))))
print('\nGreat!! The goal keeper for the game will be {}.'.format(players[keeper-1]))
1 Answer

jb30
44,806 PointsYour code is clear and easy to follow. It has meaningful variable names. As long as you get at least one player name and valid input, your code will be fine.
splayers = ''
is declared near the beginning of your code, but you never use its value. It can be safely removed.
If the user's first input is n
, then no players are added to the players list. This is not a problem until you do players[keeper-1]
, which, for any int value of keeper, will give you an IndexError: list index out of range.
If your user's input is yes
, the value of ask.lower() == 'y':
will be False. This may or may not be what you intended. You could use ask.lower().startswith()
, or you could assume your users will follow directions and just type y
or Y
.
You could change your for loop to
for index, player in enumerate(players, 1):
print(f'Player {index}: {player}')
F-strings are another way of formatting strings, and may be faster than using .format
. Python for loops tend to be of the form for player in players:
to get at the items directly, and switch to using for item, index in enumerate(players):
if they need index numbers. Using enumerate(players, 1)
will start the index counter at 1.
What do you want to happen if the user doesn't type something that can be parsed as in int to your keeper variable? Right now, you get a ValueError: invalid literal for int() with base 10. You could use
try:
keeper = int(input('\nPlease select the goal keeper by selecting the player number. (1-{}) '.format(len(players))))
print('\nGreat!! The goal keeper for the game will be {}.'.format(players[keeper-1]))
except ValueError:
pass;
The length of the string keeper = int(input('\nPlease select the goal keeper by selecting the player number. (1-{}) '.format(len(players))))
is 115 characters, and print('\nGreat!! The goal keeper for the game will be {}.'.format(players[keeper-1]))
is 84 characters. You might want to keep your lines at a maximum of 80 characters to increase readability.
I would not necessarily make ask = input('Would you like to add a player to the list? (Y/N) ')
into a function, but I might want to have 'Would you like to add a player to the list? (Y/N) '
stored in a variable as to not need to retype it and have consistent spelling. You don't have spelling errors, but the more you type something out, the more likely it is that you will misspell something, and if you want to change the prompt, you would only have to change it in one place.
Chris Freeman
Treehouse Moderator 68,441 PointsChris Freeman
Treehouse Moderator 68,441 PointsNice feedback!
JASON PETERSEN
14,266 PointsJASON PETERSEN
14,266 PointsThanks so much for that great feedback