Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial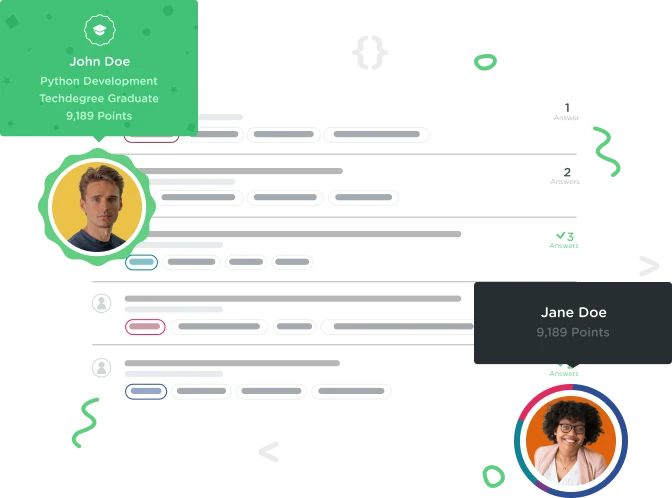
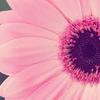
Carol Holmes
1,626 PointsPlease give me the answer to challenge task 2 of 2 in "creating reusable code with functions" /javascript.
I would benefit from the answer. Thanks.
function returnValue("My argument"){
return ("My argument");
}
returnValue("My argument");
var echo = returnValue();
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
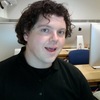
Nathan Smutz
9,492 PointsYour first attempt was very close. You might have missed the idea of function arguments.
In code you've probably seen functions used like:
i_equal_ten_now = multiply_by_two(5);
In this case, 5
was passed in as an "argument" and you might guess that 10
was "returned" out the other side.
When you make a function, you give a name to the thing(s) passed in so you can work with it. The multiply_by_two
function is made like this:
function multiply_by_two( i_am_an_argument ) {
var two_times_the_argument = 2 * i_am_an_argument;
return two_times_the_argument;
}
So, the argument (named argument
in this case) is a variable that gets assigned to whatever you put between the parentheses when you use ("call") the function. Here we've made another variable (two_times_the_argument
) and set it equal to (not surprisingly) 2 * i_am_an_argument
.
In the last part of the function, we "return" the value of the variable two_times_the_argument
. Whatever comes after the return
is the value you get when you call the function. So when we do something like:
I_equal_ten_now = multiply_by_two(5);
the variable I_equal_ten_now
has a value of 10.
Now the function we made above has an extra step in it. We didn't really need to make that extra variable in there. We could have just defined it like:
function multiply_by_two( argument ) {
return 2 * argument;
}
// And we use the function like this
var i_equal_ten_now = multiply_by_two(5);
Following through this example, when we called the function, multiply_by_two(5)
, looking inside the function, argument
now has a value of 5
. So, return 2 * argument;
means the same as return 2 * 5;
or return 10;
Now, the value "returned" by multiply_by_two(5)
is 10
In this case we set a variable (i_equal_ten_now
) to catch that value of 10
when we return it.
So, after running the line i_equal_ten_now = multiply_by_two(5);
the variable i_equal_ten_now
has a value of 10
.
In the case of this question, they're just checking that you understand arguments and return statements so the function is really simple and can look like:
function returnValue(i_am_an_argument) {
return i_am_an_argument;
{
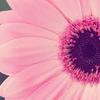
Carol Holmes
1,626 PointsThank you for such detailed response.