Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial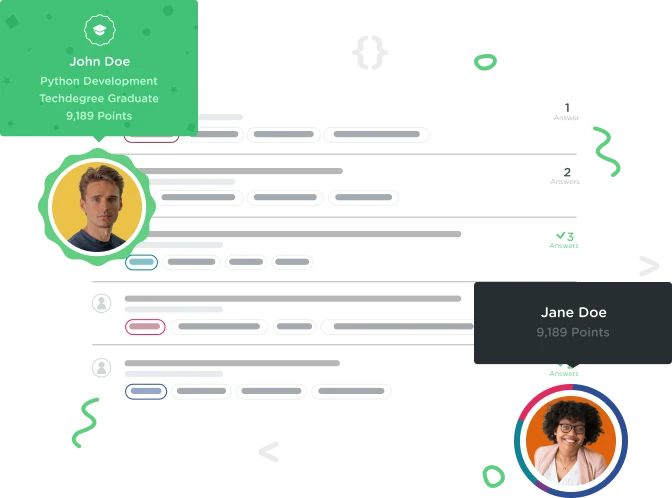

Greg Isaacson
Courses Plus Student 702 PointsPlease help!
Hi All...
Still getting used to writing my own functions
Please help me with this code...what am I doing wrong???
As I understand functions, you need to start with:
def "insert function name"()
My count is = 10
then print ("hi" * count or 10).
Then I need to call it again printer()...
thanks!:)
def printer():
count = 10
print("hi"*count)
printer()
1 Answer

Umesh Ravji
42,386 PointsHi Greg, your almost there, just two issues.
1.
The printer function should take one argument count
so make sure to include that in the definition too. Since this is passed into the function, you shouldn't declare a local variable count
, so remove that.
2
. You don't need to call the printer
function again.
def printer(count):
print("hi" * count)

Greg Isaacson
Courses Plus Student 702 PointsOk Starting to understand this better...
So def printer(This is kind of like giving a variable a name): print("hi" * count) --->> This "connects" the number of hi's in the print command to the value in count IN the () of def printer.
Hope im understanding this correctly:) Thanks!
Dustin James
11,364 PointsGreg,
I think you are getting it!
Define a function named printer. When you call the printer function you know you will pass in a number so your function can print 'hi' as many times as that number is. For that reason you put in an argument that you name count. Now you have a function you can use the same function multiple times for different reasons.
ten = 10
one = 1
def printer(count):
print('hi ' * count) # notice the space after 'hi_'
printer(ten) # this will print 'hi hi hi hi hi hi hi hi hi hi '
printer(20) # this will print 'hi hi hi hi hi hi hi hi hi hi hi hi hi hi hi hi hi hi hi hi '
printer(one) # this will print 'hi '
This is the beauty of functions. Making your life easier and not having to repeat the same code multiple times. Can you take this small function to another level? What if I wanted to input a string of my choosing into the printer function, how would you do that?
Hint, you will need to replace 'hi ' with something else.
Dustin James
11,364 PointsDustin James
11,364 PointsThe way this function would work, you would print 'hihihihihihihihihihi' every time you called printer(). What if I wanted to print 'hi' twenty times? I couldn't use this same function.