Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial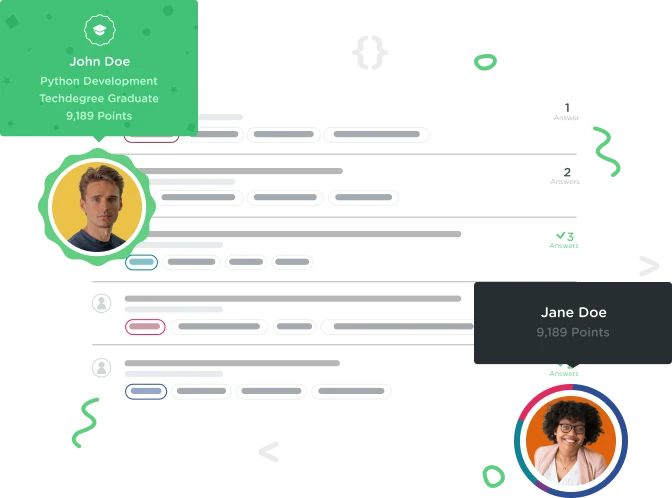

tafadzwa manzunzu
3,601 Pointsplease help
Create a method named WordsWithCountGreaterThan that takes an integer as a parameter. The method should return a dictionary of all of the words that have a count greater than the parameter passed in. The dictionary should contain the word and the word's count.
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
if (WordCount.ContainsKey(word))
{
WordCount[word]++;
}
else
{
WordCount.Add(word, 1);
}
}
public Dictionary<string, int> WordsWithCountGreaterThan(int i)
{
WordCount = WordCount.Where(p => p.Value > i).ToDictionary(p => p.Key, p => p.Value);
return WordCount;
}
}
}
4 Answers
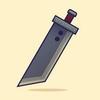
Allan Clark
10,810 PointsTry adding this to the top
using System.Linq;

Samuel Ferree
31,722 PointsYou're modifying your classes member dictionary in your getter. When that method is called, it doesn't just find the words, it removes all the other ones from your word counter. This might be messing with multiple calls to the method when the program tries to test it. Try assigning the results to a local variable, or returning in place
Local Variable Example
public Dictionary<string, int> WordsWithCountGreaterThan(int i)
{
Dictionary<string, int> results = WordCount.Where(p => p.Value > 1).ToDictionary(p => p.Key, p => p.Value);
return results;
}
Return In Place Example
public Dictionary<string, int> WordsWithCountGreaterThan(int i)
{
return WordCount.Where(p => p.Value > 1).ToDictionary(p => p.Key, p => p.Value);
}
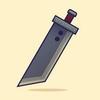
Allan Clark
10,810 PointsAlso this!

Matthew Miles
13,770 Points// this method will compare a dictionary item's value against a passed in integer, and build a new dictionary of those // keys with values greater than the passed in integer.
Dictionary<string, int> returnedDictionary = new Dictionary<string, int>();
public Dictionary<string, int> WordsWithCountGreaterThan(int numberOfWords)
{
foreach (KeyValuePair<string, int> code in WordCount)
{
if (code.Value > numberOfWords)
{
returnedDictionary.Add(code.Key, code.Value);
}
}
return returnedDictionary;
}

Kevin Gates
15,053 PointsThis works. Let me know if you have any questions:
using System.Collections.Generic;
namespace Treehouse.CodeChallenges
{
public class LexicalAnalysis
{
public Dictionary<string, int> WordCount = new Dictionary<string, int>();
public void AddWord(string word)
{
try
{
WordCount.Add(word, 1);
}
catch
{
WordCount[word] +=1;
}
}
public Dictionary<string,int> WordsWithCountGreaterThan(int count)
{
var resultSet = new Dictionary<string,int>();
foreach(KeyValuePair<string, int> entry in WordCount)
{
if ( entry.Value > count)
{
resultSet.Add(entry.Key,entry.Value);
}
}
return resultSet;
}
}
}