Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial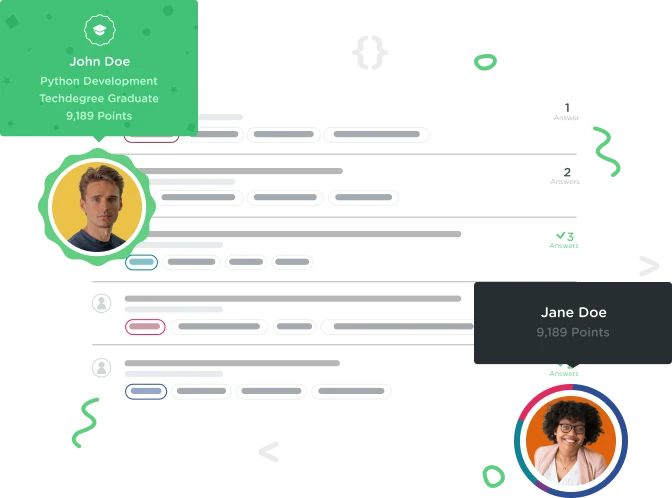

Ikechukwu Arum
5,337 PointsPlease help! Error message: wrong number of print.
import random
start = 5
def even_odd(num): # If % 2 is 0, the number is even. if num %2 ==0: print("{} is even".format(num)) # Since 0 is falsey, we have to invert it with not. #return not num % 2 print("{} is odd".format(num))
while start >5 : num = random.randint(1,99) even_odd(num) start -=1
import random
start = 5
def even_odd(num):
# If % 2 is 0, the number is even.
if num %2 ==0:
print("{} is even".format(num))
# Since 0 is falsey, we have to invert it with not.
#return not num % 2
print("{} is odd".format(num))
while start >5 :
num = random.randint(1,99)
even_odd(num)
start -=1
2 Answers

Manish Giri
16,266 PointsA couple of things -
- You have
start = 5
at the top, but your while loop condition iswhile start > 5
. Sincestart
is not greater than5
, thewhile
loop will never execute. - It looks like you've modified the
even_odd
method itself. You were required to use this method in yourwhile
loop to check if the random number was odd or even, then print accordingly. With the changed method that you have -
def even_odd(num):
# If % 2 is 0, the number is even.
if num %2 ==0:
print("{} is even".format(num))
# Since 0 is falsey, we have to invert it with not.
#return not num % 2
print("{} is odd".format(num))
If the number is even, you'll get two prints instead of one, since there's no return
after the if
block. It prints the statement in the if
, and then the one at the last line too.

Ikechukwu Arum
5,337 Pointsok i figured it out. Thanks for your help
Ikechukwu Arum
5,337 PointsIkechukwu Arum
5,337 Pointsthanks for the reply. I understand what you were saying about the while loop condition but i don't understand what you mean by getting two prints and etc
Manish Giri
16,266 PointsManish Giri
16,266 PointsLet's take an example -
If you run this code (here,
even_odd
is exactly the same as what you have), you'll get two print statements -Why?
When
num
is even, like in this case with4
, thisif
block executes-and you get the first print
4 is even
.But then the function continues executing, because there's no
return
orbreak
. The next line of code encountered after theif
block isprint("{} is odd".format(num))
. Which is why you get4 is odd
, even though4
is not odd.To fix this -