Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial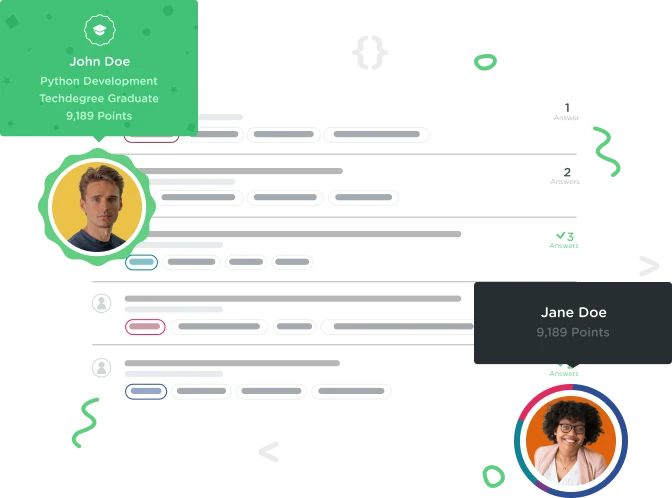

D Anthony
6,045 PointsPlease help, I do not understand this code challenge.
The first part of this code challenge is easy. It simply wants us to create a class called D20 that extends the Die class. Simple. However, I am struggling with the second part of the challenge. I initialized the hand class with the type of dice being D20, imported from the dice.py file. I then set self.die_type to equal D20.
In the roll method I initialized it with the num_dice, being the number of dice to be rolled, as 1. Then used a for loop to append each roll value to self using the D20 object. Lastly the challenge gave us the total property.
Now when I run this in my terminal it seems to work fine. I am able to import both the dice and hand classes and I am able to create an instance of the Hand class no problem. When I check my instance I can see that there are values in my list equal to the number of dice rolled. I am even able to use the roll method on the instance of Hand class as well as use the total property on my instance.
While this seems to all work properly, I get errors when I attempt to submit the code challenge. The main one I usually get is 'Bummer: 'Hand' doesn't have roll method'. I am confused as to what I am missing in my code or if I even implemented it the way that they wanted. I would greatly appreciate if anyone could help me figure out what I am doing wrong. Thank you.
import random
class Die:
def __init__(self, sides=2):
if sides < 2:
raise ValueError("Can't have fewer than two sides")
self.sides = sides
self.value = random.randint(1, sides)
def __int__(self):
return self.value
def __add__(self, other):
return int(self) + other
def __radd__(self, other):
return self + other
class D20(Die):
def __init__(self, sides=20):
super().__init__(sides)
from dice import D20
class Hand(list):
def __init__(self, die_type=D20):
super().__init__()
self.die_type = die_type
def roll(self, num_dice=1):
for _ in range(num_dice):
self.append(self.die_type())
@property
def total(self):
return sum(self)
1 Answer

Richard Li
9,751 PointsThe instruction said that he will use Hand.roll(2) then it return a instance of the Hand class which makes Hand.roll not a regular method but a @classmethod.
A class method is a method who lives inside a class but can create the class itself when called.