Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial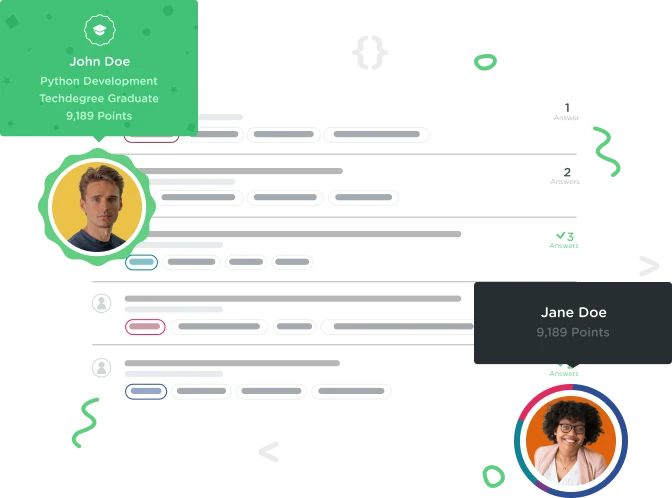
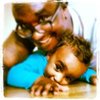
Matthew Denton
598 PointsPlease help me fix the "getLineFor" Method
I have modeled an assistant for a tech conference. Since there are so many attendees, registration is broken up into two lines. Lines are determined by last name, and they will end up in line 1 or line 2. Please help me fix the getLineFor method.
Thanks in Advance
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int lineOne = 1, lineTwo = 2;
if ( lastName = char 'A' > 'M') {
return lineOne;
} else {
return lineTwo;
}
}
}
6 Answers
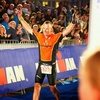
Steve Hunter
57,712 PointsHi Matthew,
Let's see what we can do for you here.
The question is asking you to send people either down line one or two depending on the first letter of their last name.
You've correctly thought an if
statement would do the trick. First, though, we need to get the first letter of the surname. The name is passed in as the method parameter called lastName
- it is a String object. So we can work with that. A String object has a method called charAt()
which lets you get, you've guessed it, the character at the number you choose in the brackets. So, to get the first letter, this being Java, you ask for charAt(0)
- that'll be the first letter.
Right, so we have that - so we now need to compare it to the letters that decide which queue to use. Any letter from A to M goes to the first queue - the rest go to the other. We can compare letters just like numbers, thankfully, so we don't have to go through every letter of the alphabet! So, A
is less than M
but M
is also OK to go to queue one. So everything from A
to M
can be expressed as any letter that's either M
or less than it.
Now we're getting somewhere.
if(lastname.charAt(0) <= 'M')
is our condition - we just need to send them to line one.
if(lastName.charAt(0) <= 'M') {
line = 1;
} else {
// do something else
}
So, what to put in the else
bit? The only other option - there's only two lines of people:
if(lastName.charAt(0) <= 'M') {
line = 1;
} else {
line = 2
}
Make sure you return
the result of line
and we're done!
The complete code looks like:
public class ConferenceRegistrationAssistant {
public int getLineFor(String lastName) {
/* If the last name is between A thru M send them to line 1
Otherwise send them to line 2 */
int line = 0;
// here's your code
if(lastName.charAt(0) <= 'M') {
line = 1;
} else {
line = 2;
}
return line;
}
}
That should do it for you.
Steve.

Sandy Woods
1,082 PointsSteve, that was such an eloquent and well worded way of breaking that code challenge down. Treehouse, please hire this guy!
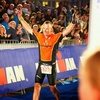
Steve Hunter
57,712 PointsThanks Sandy - your kind words are appreciated! :-) I'm not sure Ben Jakuben has any vacancies. ;-)

Ben Jakuben
Treehouse TeacherYes, that is a wonderful answer, Steve! There may not be any vacancies currently on our job board, but look for more in 2016! :-D

Sandy Woods
1,082 PointsHey Steve, I'd like to know if you'd be interested in helping me with future snags that I'm sure I'll come across on this Java track? If so, can I get your contact info, like e-mail address? I'm not sure if there is a way I can contact you directly here in the Treehouse community.
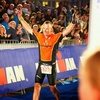
Steve Hunter
57,712 PointsYep. No problem. My profile has my Facebook, Twitter and company website. My email address will be on my site, I'm sure.
Happy to help out where I can.
Steve.

Sandy Woods
1,082 PointsOk. Thanks. On the Big Dog website, I saw this email address enquiries@bigdogconsultants.co.uk which doesn't seem to be specific to you, I assume. I'll pitch my first question there and just let me know if you'd like me to send it to another address
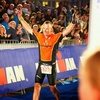
Steve Hunter
57,712 PointsSorry, I thought I was on there directly. Use steve.hunter@ and I'll definitely get that.
It's my company and there's only me!

miguelcastro2
Courses Plus Student 6,573 PointsI would recommend learning Regular Expressions to help you match characters. Regular expressions give you the ability to look for patterns within strings in a very effective way. The below example looks for any lastname that starts with the range of letters from A through M:
if (lastName.matches("^[A-Ma-m].*")) {
System.out.println("Within A-M");
} else {
System.out.println("Not Within A-M");
}

Craig Dennis
Treehouse TeacherWhoops. Miguel not quite yet;)
Matthew Denton
598 PointsMatthew Denton
598 PointsHi Steve
Just wanted to say thank you, not just for answering my question but also the thorough explanation, It's really helped my understanding.
Thanks
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsNo problem at all - glad to be of help! And I appreciate you taking the time to say that - thanks! :-)
Steve.
Nnenna Iheke
6,260 PointsNnenna Iheke
6,260 PointsSteve you da man thanks. I was giving up.
Steve Hunter
57,712 PointsSteve Hunter
57,712 PointsHey, no problem, Nnenna.
You can refine my solution still further. The
else
is pointless:That's not as clear, but does the same with fewer lines. That's not always a good thing - I prefer the first solution. :-)
Steve.