Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial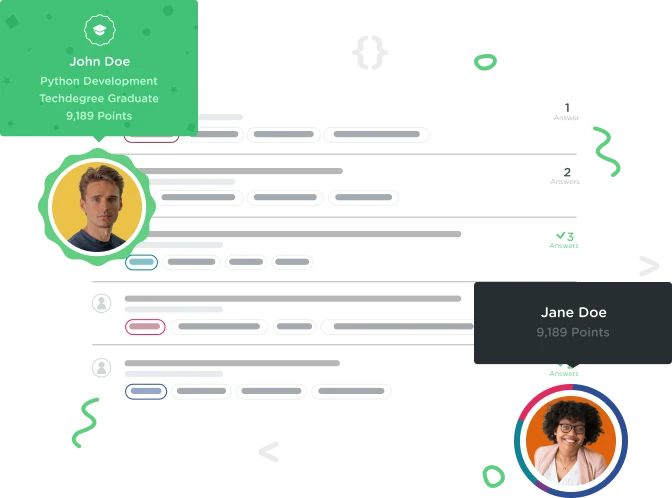

Dena G
441 PointsPlease HELP me! I'm stuck on the Mutual Friendships video.
When the user clicks on the 'Accept Friendship' button a flash message is displayed stating that they are now friends with me. However, it does not update on the users Friends page. It still shows that the user has a 'Friendship requested' and to accept it. Somehow it is not updating
It shows that the friendship started on my end however, it wont update for the other user. It still says that the person has a Friendship request.
Please see my code below. I'm not sure if its because I am not using the attribute in my Model since I am on Rails 4. Thank you in advance.
User_Friendship Model
class UserFriendship < ActiveRecord::Base
belongs_to :user
belongs_to :friend, class_name: 'User', foreign_key: 'friend_id'
state_machine :state, initial: :pending do
after_transition on: :accept, do: [:send_acceptance_email, :accept_mutual_friendship!]
state :requested
event :accept do
transition any => :accepted
end
end
def self.request(user1, user2)
transaction do
friendship1 = create!(user: user1, friend: user2, state: 'pending')
friendship2 = create!(user: user2, friend: user1, state: 'requested')
friendship1.send_request_email
friendship1
end
end
def send_request_email
UserNotifier.friend_requested(id).deliver
end
def send_acceptance_email
UserNotifier.friend_request_accepted(id).deliver
end
def mutual_friendship
self.class.where({user_id: friend_id, friend_id: user_id}).first
end
def accept_mutual_friendship!
# Grab the mutual friendship and update the state without using the state machine, so as
# not to invoke callbacks.
mutual_friendship.update_attribute(:state, 'accepted')
end
end
UserFriendship Controller
class UserFriendshipsController < ApplicationController
before_filter :authenticate_user!
def index
@user_Friendships = current_user.user_friendships.all
end
def accept
@user_friendship = current_user.user_friendships.find(params[:id])
if @user_friendship.accept_mutual_friendship!
flash[:success] = "You are now friends with #{@user_friendship.friend.name}"
redirect_to user_friendships_path
else
flash[:error] = "That friendship could not be accepted"
redirect_to user_friendships_path
end
end
def new
if params[:friend_id]
@friend = User.find(params[:friend_id])
raise ActiveRecord::RecordNotFound if @friend.nil?
@user_friendship = current_user.user_friendships.new(friend: @friend)
else
flash[:error] = "Friend required"
end
rescue ActiveRecord::RecordNotFound
render file: 'public/404', status: :not_found
end
def create
if params[:user_friendship] && params[:user_friendship].has_key?(:friend_id)
@friend = User.find(params[:user_friendship][:friend_id])
@user_friendship = UserFriendship.request(current_user, @friend)
if @user_friendship.new_record?
flash[:error] = "There was a problem creating this friend request."
else
flash[:success] = "Friend request sent."
end
redirect_to user_path(@friend)
else
flash[:error] = "Friend required"
redirect_to root_path
end
end
def edit
@user_friendship = current_user.user_friendships.find(params[:id])
@friend = @user_friendship.friend
end
def user_friendship
params.require(:user_friendship).permit(:user_id, :friend_id, :user, :friend, :state, :user_friendship)
end
end
1 Answer

Dena G
441 PointsI figured out the problem. The code should read:
def accept
@user_friendship = current_user.user_friendships.find(params[:id])
if @user_friendship.accept_mutual_friendship!
@user_friendship.friend.user_friendships.find_by(friend_id: current_user.id).accept_mutual_friendship!
flash[:success] = "You are now friends with # {@user_friendship.friend.name}"
redirect_to user_friendships_path
else
flash[:error] = "That friendship could not be accepted"
end
end