Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial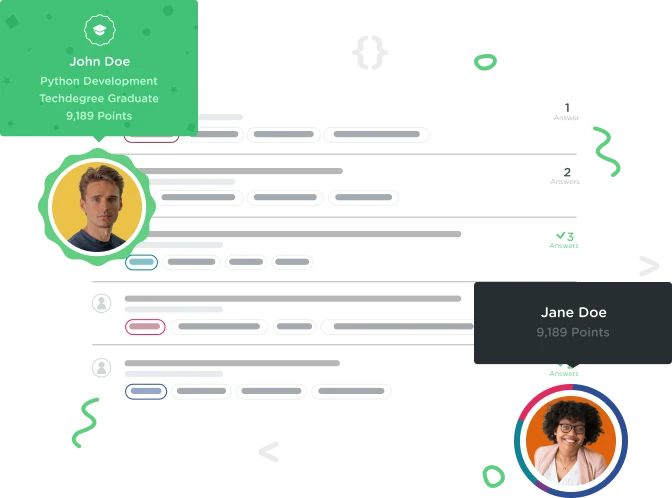
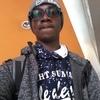
Elvin Mazwimairi
15,541 PointsPlease help me out with the correct code for this challenge
Almost done!
Now I need you to update the create_user method so that it sets the User instance's password using the User.hash_password method you just created.
import datetime
from argon2 import PasswordHasher
from itsdangerous import (TimedJSONWebSignatureSerializer as Serializer,
BadSignature, SignatureExpired)
from peewee import *
DATABASE = SqliteDatabase('courses.sqlite')
HASHER = PasswordHasher()
class User(Model):
username = CharField(unique=True)
email = CharField(unique=True)
password = CharField()
class Meta:
database = DATABASE
@classmethod
def create_user(cls, username, email, password, **kwargs):
email = email.lower()
try:
cls.select().where(
(cls.email == email) | (cls.username**username)
).get()
except cls.DoesNotExist:
user = cls(username=username, email=email)
user.password = cls.set_password(password)
cls.HASHER.hash(user.password)
user.save()
return user
else:
raise Exception("User with that email or username already exists.")
@staticmethod
def verify_auth_token(token):
serializer = Serializer(config.SECRET_KEY)
try:
data = serializer.loads(token)
except (SignatureExpired, BadSignature):
return None
else:
user = User.get(User.id == data['id'])
return user
@staticmethod
def hash_password(password):
return HASHER.hash(password)
def set_password(password):
return HASHER.hash(password)
def verify_password(self, password):
return HASHER.verify(self.password, password)
def generate_auth_token(self, expires=3600):
serializer = Serializer(config.SECRET_KEY, expires_in=expires)
return serializer.dumps({'id': self.id})
class Course(Model):
title = CharField()
url = CharField(unique=True)
created_at = DateTimeField(default=datetime.datetime.now)
class Meta:
database = DATABASE
class Review(Model):
course = ForeignKeyField(Course, related_name='review_set')
rating = IntegerField()
comment = TextField(default='')
created_at = DateTimeField(default=datetime.datetime.now)
created_by = ForeignKeyField(User, related_name='review_set')
class Meta:
database = DATABASE
def initialize():
DATABASE.connect()
DATABASE.create_tables([User, Course, Review], safe=True)
DATABASE.close()
Jason Anders
Treehouse Moderator 145,860 PointsJason Anders
Treehouse Moderator 145,860 PointsModerator note: All duplicate posts regarding this topic / question have been deleted. Please refrain from multiple postings regarding the same subject to the community, especially in the span of 30 minutes.
There are many students from many time zones that participate in the Community, so there may be times when it take a bit for a post to be addressed, but flooding the Community with duplicates only clutters up the Forum and can frustrate many students trying to search the Community for answers.
Thank you for your cooperation.
Jason ~Treehouse Community Moderator