Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial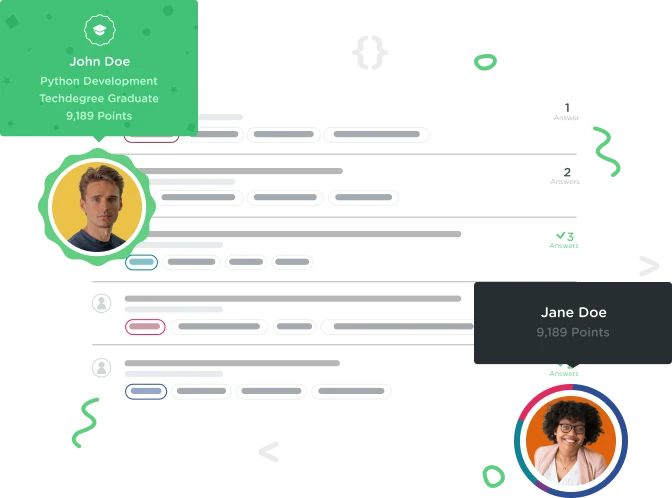
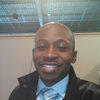
Brian Johnson
Front End Web Development Techdegree Graduate 20,818 PointsPlease help me see what i am doing wrong here! my code seems to match his.
//Please check this out and let me know what i missed thanks
document.addEventListener('DOMContentLoaded', () => { //Use this EVERYTIME YOU START JAVASCRIPT CODING
const form = document.getElementById('registrar');
const input = form.querySelector('input');
const mainDiv = document.querySelector('.main');
const ul = document.getElementById('invitedList');
const div = document.createElement('div');
const filterLabel = document.createElement('label');
const filterCheckbox = document.createElement('input');
filterLabel.textContent = "Hide those who haven't responded";
filterCheckbox.type = 'checkbox';
div.appendChild(filterLabel);
div.appendChild(filterCheckbox);
mainDiv.insertBefore(div, ul);
filterCheckbox.addEventListener('change', (e) =>{
const isChecked = e.target.checked;
const lis = ul.children;
if(isChecked){
for(let i = 0; i < lis.length; i += 1){
let li = lis[i];
if(li.className === 'responded'){
li.style.display = '';
}else{
li.style.display = 'none';
}
}
}else{
for(let i = 0; i < lis.length; i += 1){
let li = lis[i];
li.style.display = '';
}
}
});
function createLi(text) {
function createElement(elementName, property, value) {
const element = document.createElement(elementName);
element[property] = value;
return element;
}
function appendToLi(elementName, property, value){
const element = createElement(elementName, property, value);
li.appendChild(element);
return element;
}
const li = document.createElement('li');
appendToLi('span', 'textContext', text);
appendToLi('label', 'textContent', 'Confirmed')
.appendToLi(createElement('input', 'type', 'checkbox'));
appendToLi('button', 'textContent', 'edit');//created a edit button to edit an invitee
appendToLi('button', 'textContent', 'remove');//created a remove button to remove an invitee
return li;
}
form.addEventListener('submit', (e) => {
e.preventDefault(); // call the prevent default method on the event object referred to by the (e) parameter
const text = input.value; //assign the input value into a variable called text
input.value = ''; // clears the input field back after you user clicks or enters the input value
const li = createLi(text);
ul.appendChild(li);
});
//adding a delegated handler that marks guest off when they responded to our user's RSVPs
ul.addEventListener('change', (e)=>{
const checkbox = event.target;
const checked = checkbox.checked;
//change the class of the list item when the checkbox is checked
//first grab a reference tp the list item ** its the checkbox's grandparent because the label is a child of the list item
const listItem = checkbox.parentNode.parentNode;
if(checked){
listItem.className = 'responded'
} else{
listItem.className = '';
}
});
// user can edit, save & remove names once a particular button is clicked
ul.addEventListener('click', (e)=>{
if (e.target.tagName === 'BUTTON'){
const button = e.target;
const li = button.parentNode;
const ul = li.parentNode;
if(button.textContent === 'remove'){
ul.removeChild(li);
} else if (button.textContent === 'edit'){
const span = li.firstElementChild;
const input = document.createElement('input');
input.type = 'text';
input.value = span.textContent;
li.insertBefore(input, span);
li.removeChild(span);
button.textContent = 'save';
} else if (button.textContent === 'save'){
const input = li.firstElementChild;
const span = document.createElement('span');
span.textContent = input.value;
li.insertBefore(span, input);
li.removeChild(input);
button.textContent = 'edit';
}
}
});
});
Steven Parker
231,275 PointsSteven Parker
231,275 PointsTo facilitate a complete replication of your issue, make a snapshot of your workspace and post the link to it here.
It would also help if you describe what the issue is you are experiencing.