Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial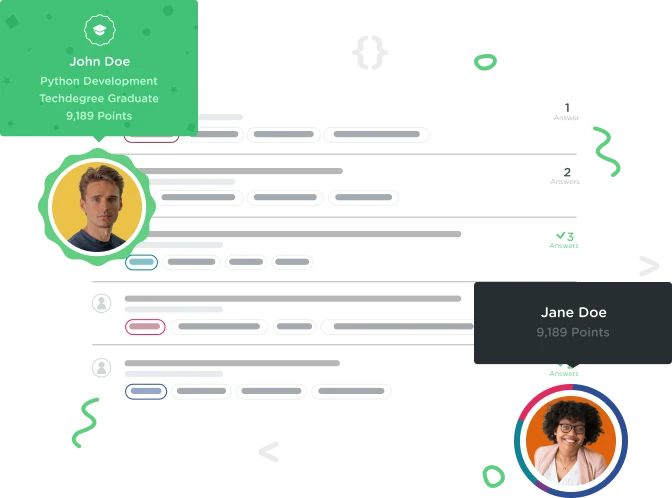
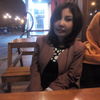
Ayesha Ghayas
1,443 PointsPlease help me solve it
Okay, so let's work on the User object now. I really didn't get too far. Can you please add the private fields to store the parameters defined in the constructor? Ooh while you're at it can you add the appropriate getters? Thanks!
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
2 Answers
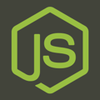
Granit Berisha
14,150 PointsThis is for the User class, hope it helps:)
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
}
public void setFirstName(String firstName) {
mFirstName = firstName;
}
public void setLastName(String lastName) {
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
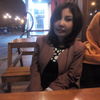
Ayesha Ghayas
1,443 PointsThank you !

Matt Bloomer
10,608 PointsI am kind of confused...why do we set the strings as private if we are just going to turn around and put them as public in the constructor?
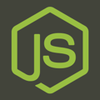
Granit Berisha
14,150 PointsSo we typically set fields to private because we wont everybody to see what is the status of that field and it can go further and change the status
example in Class Person
public String name = " Granit ";
he can easy change the status Person.name = " Something else ";
so we set this in private so he can see or change and then we set a constructor / method to access this field why because there we set parameters when will it change or not , so you didn't understand i guess because Iam saying the same thing + some other work let me explain in another example
In another Class Bank we have a field with the total amount of money so when he will add some money to he's account he can easily do
public Int money = 0; money +=10; money +=30;
and when he need the money
money -=40;
and yes he can do money -=50; because no rules will block to do that so he can have money as much as he want in the bank so we set this in private and set methods to check if he has money that give it to him if not dont let it go minus
so private Int money = 50; public void getmoney(int howmuchmoney){ if ( money >= howmuchmoney){ money -=howmuchmoney; System.out.println("Here your money "+howmuchmoney+" and the money left " + money); } else { System.out.println("You dont have that amount of money "); } }
So getmoney(40); will print "Here your money 40 and the money left 10"
If the type getmoney(55);
will print "You dont have that amount of money"
I'm sorry if i writted to much I hope this will help to understand
Ayesha Ghayas
1,443 PointsAyesha Ghayas
1,443 Pointsstuck on challenge 2 of 4: