Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial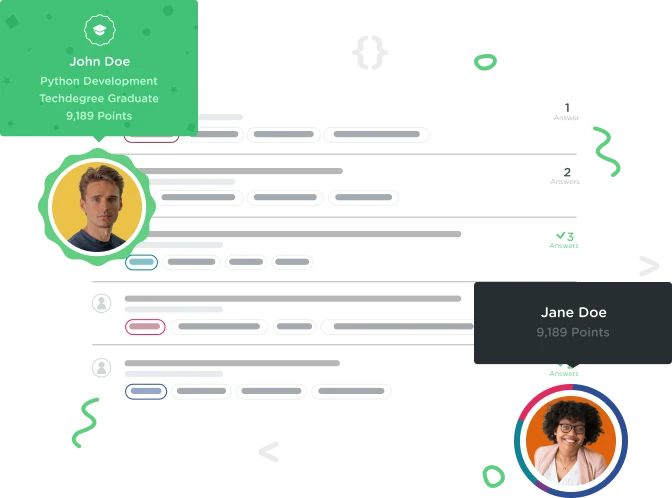
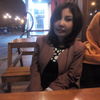
Ayesha Ghayas
1,443 PointsPLEASE HELP ME SOLVE THIS STUCK HERE FOR MONTHS
So at your new Java job, you've written a brand new Shopping Cart system for the website. After some developers have used the objects you created for a while, they ask you to make it easier to add things to the cart.
Check out more instructions in Example.java. Once you implement their request, check your work, and you will pass the challenge.
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
}
}
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
public class Product {
/* Other code omitted for clarity, but you could imagine
it would store price, options like size and color
*/
private String mName;
public Product(String name) {
mName = name;
}
public String getName() {
return mName;
}
}
11 Answers

Uthman EbdEl Khalek
Courses Plus Student 5,460 PointsI think this quiz should be rephrased, as it wasn't very clear to me as well. As it's been two days since. Below is what worked for me, It's my first post and I am not sure if I am suppose to post answers or no, but here it is anways.
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
public void addItem(Product item)
{
addItem(item,1);
}
}
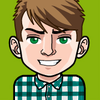
Harry James
14,780 PointsHey Ayesha!
Sorry you're still having difficulties with the challenge - I'm going to give you the answer here so that you can still finish the course. Please do check what I have written in the comments and make sure you understand what is going on rather than just placing it straight into the Code Challenge:
Answer below (Spoiler alert!)
Example.java
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
Product pez = new Product("Cherry PEZ refill (12 pieces)");
cart.addItem(pez, 5);
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the following code work, as well as keeping
* the ability to add a product and a quantity.
*/
Product dispenser = new Product("Yoda PEZ dispenser");
// Uncomment this line after using method signatures to solve their request in ShoppingCart.java
cart.addItem(dispenser);
// The only change made here was that I uncommented cart.addItem(dispenser);
}
}
ShoppingCart.java
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
// New code:
public void addItem(Product item) {
// This time, we're only going to take in the item - we don't pass in the quantity.
addItem(item, 1);
// So that we don't repeat ourselves (Maybe we wanted to change how addItem() works at a later date?), we'll just run the original method from here.
}
}
Thank you for your time and sorry for the wait! If there's anything else you don't understand, give me a shout and I'd be happy to help again :)
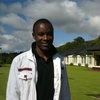
Admire Dhodho
5,411 Pointshurry you make learning much better liz can give me your email mine is thescholar9 [at] gmail .com
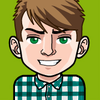
Harry James
14,780 PointsHey Admire!
Glad to hear that you liked my answers and I really appreciate your kind words :)
If you wish to email me about anything, you can do so at harryjamesuk+treehouse [at] gmail .com (Remove any spaces and replace the [at]) or you can tag me on here with @Harry James
. I always answer any emails/tags so I'll definitely get around to you - this is a good way to get your question answered if you've been waiting for a long time for a forum response (Sometimes when the forums are busy, unanswered questions get pushed back to later pages).
Also, I've updated your email in the comment to the same format as me just to hopefully stop any web crawlers from sending you spam :)

Han Wu
927 PointsHi, I know it's been five months but I was also stuck on that challenge and found this thread. I'm not really sure how Product and item are parameters. Thanks.
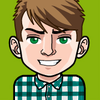
Harry James
14,780 PointsHey Han!
In this challenge, you want to uncomment the cart.addItem(dispenser);
line and make it work.
Right now, if you look in ShoppingCart.java, you can see that the addItem() method takes in a item and a quantity. We want to make it so that this still works, but we can also just pass in just the item and the code will still work.
Remember how we can have more than one method with the same name but with different parameters? Yeah! That's what you're going to have to use ;)
Hopefully this should help explain the question for you but if you get stuck along the way, give me a shout and I'll help you out a bit more :)

Han Wu
927 PointsOh, I see. Thanks.

Emmet Lowry
10,196 Pointswhats the difference between classes and methods. thx
and what does shoppingCart cart mean
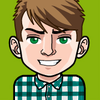
Harry James
14,780 PointsHey again Emmet!
A class is where we store all of our methods - we can have as many of them as we want and we can get data and run methods in other classes throughout our code.
The ShoppingCart itself is a class, as shown here:
public class ShoppingCart {
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
}
If you take a look at the first line, we can see the class declaration, public class ShoppingCart
.
So, the keyword public
is used to mark the visibility of this class. If we had the class as private
, no other classes would be able to see the class - or use any of the code inside of it. public
however, means that any class has access to this class (Any class can create a ShoppingCart object). These keywords are called access modifiers and there are a few more of them, which you'll likely come across in later projects.
Next, we say that this is a class
and then finally, we give the class a name (In this case, ShoppingCart
).
Note that I may refer to the ShoppingCart class as an object - this is because Java is an object-orientated programming language and we'll soon learn that classes are also objects, much like String
s and int
s are.
A method however, is contained within a class. Methods run whatever code is inside them and they can take in parameters so that we can pass something into a method and that object we pass in can then be referred to again in that method.
A bit of a spoiler here for this Code Challenge but, we can have more than 1 method in a class with the same name, as long as they take in different parameters. Likewise, we can also have as many methods with different names in the class as we want.
Now, on the line ShoppingCart cart = new ShoppingCart();
, we're actually creating a new variable which we're going to call cart
(It could be anything but this is an appropriate name). This variable is of the data type ShoppingCart
(That's our class that we were using before! And, as it's public
, we have access to it). So, we must then set this variable to a ShoppingCart
object as, that's what the data type is.
So, we set it to a new ShoppingCart()
- but what does this mean? Well, the new
keyword is used to create a new instance of this ShoppingCart class (Take a look at my answer here if you want to learn more about instances) and then we use the () to run the constructor of this method.
We've used constructors before in this course - it means that when we create a new instance of the object, some code is ran and we can also take in parameters into the constructor. You can think of a constructor as being a lot like a default method - a method that's ran whenever the class is called.
Note that this class does not contain a constructor however, Java actually creates one for us. If we don't provide our own constructor, Java will make one but, this constructor won't do anything apart from return a ShoppingCart
object.
So, what is a ShoppingCart
object? Well, an object is just like a real-life object. Objects can have properties and, we can set the properties of this object. So, if we were to make member variables in this class (Variables that can be accessed throughout the class within all method scopes), we can create an instance of this object and then set properties for this object (Values for the member variables).
If any of this confuses you right now (I know, there's a lot of text here but I wanted to explain things best I could so that you wouldn't have too many questions though you've probably already got a load in your head right now!) then don't worry - it should all start coming into place when you're actually programming.
You're just starting to learn the language and that takes time so, as long as you have the basic gist of what's going on, all of the other stuff will start piecing together.
If you do however, have some questions you really want answered beforehand, please do share them with me and I'll be happy to try my best to explain them. Likewise, if there's something you want me to explain a bit better here, let me know and I'll try to rephrase it in a more understandable manner :)
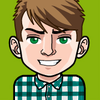
Harry James
14,780 PointsHey Ayesha!
Sorry to hear you're having problems with this code challenge but me and the other forum users will definitely help you out!
So, the problem is that the developers often find themselves adding 1 of a product to the basket. They always have to provide this quantity however, and they want to be able to provide only the product in the method and it would automatically know that they want to add 1 of that item.
So, to do this, we can use our knowledge of methods. Take a look in ShoppingCart.java at this method:
public void addItem(Product item, int quantity) {
System.out.printf("Adding %d of %s to the cart.%n", quantity, item.getName());
/* Other code omitted for clarity */
}
We want to be able to do the same thing here but with a method that only takes in the product and will then automatically know that 1 of that item is to be added.
Hopefully this should clarify the question but, if you still don't understand what to do, I'll provide you with a few more hints :)
Also, I updated your question as you provided the code twice - when reporting a problem from the Code Challenge, it automatically includes the code :)
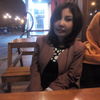
Ayesha Ghayas
1,443 PointsNo this doesn't help I need clear full answer
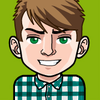
Harry James
14,780 PointsOk Ayesha.
Remember how we can have more than one constructor with the same name yet they take in different parameters? We can do the same with method calls.
In Example.java, we can see the line of code that runs the method (Make sure to uncomment this if you haven't already!):
cart.addItem(dispenser);
So, we want to create another method that only takes in the product, the dispenser. I'd like you to try and do the rest (You don't learn much if I just straight-away tell you the answer) but, I'll start you off with the first line of the second method:
ShoppingCart.java
public void addItem(Product item) {
// We want this to do the same thing as our other addItem() method that takes in a quantity as well. Only this time, we should make the code add 1 of the product to the cart.
}
If you still can't get the challenge after this, for my 3rd reply I will tell you the answer which you can then work through to find out how I answered the question but, I do encourage you to try it yourself.
Thanks for your patience!
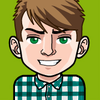
Harry James
14,780 PointsI'll tag Craig Dennis here to take a look at this as a few of you found this unclear. Perhaps something like this would be better?
Example.java
public class Example {
public static void main(String[] args) {
ShoppingCart cart = new ShoppingCart();
// This code is used to add a quantity of a product to the cart:
Product pez = new Product("Cherry PEZ refill");
cart.addItem(pez, 5)
/* Since a quantity of 1 is such a common argument when adding a product to the cart,
* your fellow developers have asked you to make the code commented below work, as well
* as keeping the ability to add a product and a quantity.
*/
// ** Uncomment these lines after using method signatures to solve their request in ShoppingCart.java: **
// Product dispenser = new Product("Yoda PEZ dispenser");
// cart.addItem(dispenser);
}
}
I updated the comments and moved some things around slightly, as well as removing the quantity of "Cherry PEZ refill" as that could also confuse students.
Ayesha Ghayas and Uthman EbdEl Khalek - let me know if this is better explained and/or if you have any better feedback for this. Note that this doesn't mean the challenge will definitely be rephrased, this is only feedback.
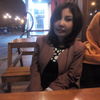
Ayesha Ghayas
1,443 PointsI'm tired nothing is working for me my answer
public void addItem(Product item) {
addItem(item,1);
}
Please please help me

Emmet Lowry
10,196 PointsHi Harry could you explain the challange a bit more having trouble understanding it.
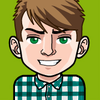
Harry James
14,780 PointsHey Emmet!
In basic terms, the challenge wants us to make this method work:
ShoppingCart cart = new ShoppingCart();
Product dispenser = new Product("Yoda PEZ dispenser");
cart.addItem(dispenser);
So, to explain this method, we're running addItem()
from the ShoppingCart
class and as the parameter, we're passing in our Product
, dispenser
.
If you take a look in this class right now, it has one addItem()
method but, this takes in a quantity and a product - we just want a method so that if we don't supply a quantity, it will default to 1
.
Hopefully this will explain what you have to do but, if there's still something you don't understand, give me a shout or look at my other answers on this question :)

Emmet Lowry
10,196 Pointsthx so much for the answer that was really helpful and i appreciate that you took the time you took to write this answer. Thanks Harry

Emmet Lowry
10,196 Pointssry for this harry but would you mind explaining member values a bit more. thanks
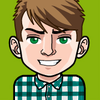
Harry James
14,780 PointsSure! I did go over member variables very briefly so here's a better explanation:
Member variables are variables that can be accessed throughout the class. So, we could do something like this:
public class ExampleClass {
String mName = "Harry";
public void exampleMethod1() {
System.out.printf("My name is " + mName);
// Would return "My name is Harry".
mName = "Emmet";
System.out.printf("My name is " + mName);
// Would return "My name is Emmet".
}
}
Showing how we created a string variable and used it in our method.
Notice how I also prefixed the variable name with a lowercase m
. This isn't required (Our code would run just fine if we didn't) however, it's a common programming convention to do this, so it's recommended that you do follow this convention!
But, what if we did this:
public class ExampleClass {
public void exampleMethod1() {
String mName = "Harry";
}
public void exampleMethod2() {
System.out.printf("My name is " + mName);
}
}
What would this return, try and think this through yourself.
...
Maybe you said it would say "My name is Harry" again? The thing is, it wouldn't. This isn't actually a member variable so, I shouldn't put the m
before name in this case (It wouldn't change the program but, it's not following the convention correctly).
We just defined this variable in the scope of exampleMethod1(). So, we can only see this variable in the two curly braces { }
. If we try to access mName
past these braces (Like we did in exampleMethod2(), this would give us an error as, the variable doesn't exist in that scope!
However, because in the first example we declared it in the curly braces of the class, we can use the variable throughout that class (Including any methods in the class). When we declared the variable in the curly braces of the method, we can use the variable throughout that method (And if you were wondering, yes, we can have methods in methods and the variable would still be valid in any child methods).
So, to conclude this, a member variable is a variable declared at the class scope. When a variable is declared, it can be used by any of its children (So, anything within the curly braces still). I'd like to show this with a final example:
public class ExampleClass {
public void exampleMethod1() {
String name = "Harry"; // Defined in exampleMethod1()
public void exampleMethod3() {
System.out.printf("My name is " + mName);
// This would work!
}
} // ExampleMethod1() ends here so we can use it anywhere above up to the starting curly brace {
public void exampleMethod2() {
System.out.printf("My name is " + mName);
// This would not work :'(
}
}
Note: name
a normal variable - not a member variable. I've made sure to show this by not prefixing with an m
Hopefully this is a good explanation but, again, if there's anything that doesn't quite make sense, give me a shout again and I'd love to help out :)

Uthman EbdEl Khalek
Courses Plus Student 5,460 PointsBetter than before. Thanks.
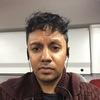
Akash Sharma
Full Stack JavaScript Techdegree Student 14,147 PointsAyesha it works for me.

Steve McNutt
2,562 PointsThanks for all of the answers and explanations to this challenge. It is very poorly worded and does not give you any good clues as to what you are supposed to be doing.
Andrew Winkler
37,739 PointsAndrew Winkler
37,739 PointsUthman is right. The challenge question was very vague. I would've grasped what the question was asking for much better if it had been worded: Make the default quantity of the addItem method 1. That's really all we are doing in this exercise.