Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial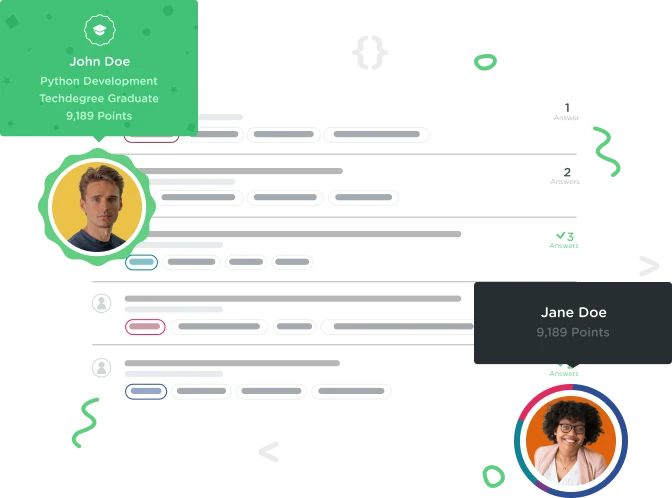
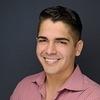
ian izaguirre
3,220 PointsPlease help me understand how to fix a fizz buzz answer I made?
I found a challenge online that says:
"Write a program that prints the numbers from 1 to 100.
But for multiples of three print “Fizz” instead of the number
and for the multiples of five print “Buzz”.
For numbers which are multiples of both three and five print “FizzBuzz”."
I wanted to create an answer that took the least amount of JavaScript code AND was flexible.
This was my attempt (which works):
function numberRange(j) {
let i = 1;
const print = message => console.log(message);
const sweepDividibleBy = someNumber => i % someNumber === 0;
while ( i < j ) {
if ( sweepDividibleBy(15) ) {
print( i + ' FizzBuzz' );
}
else if ( sweepDividibleBy(5) ) {
print( i + ' Buzz' );
}
else if ( sweepDividibleBy(3) ) {
print( i + ' Fizz' );
}
else {
print( i );
}
i++;
}
}
numberRange(101);
With my code, If I remove sweepDividibleBy(15)
from the first position and place it last, like this:
function numberRange(j) {
let i = 1;
const print = message => console.log(message);
const sweepDividibleBy = someNumber => i % someNumber === 0;
while ( i < j ) {
if ( sweepDividibleBy(3) ) { // Moved
print( i + ' Fizz' );
}
else if ( sweepDividibleBy(5) ) {
print( i + ' Buzz' );
}
else if ( sweepDividibleBy(15) ) { // Moved
print( i + ' FizzBuzz' );
}
else {
print( i );
}
i++;
}
}
numberRange(101);
Then my program stops working properly since sweepDividibleBy(15)
will not activate and thus 'FizzBuzz' will not print to the console at all.
How Can I fix my code so the order of the sweepDividibleBy()
numbers is not dependent on its location in my programs code?
2 Answers
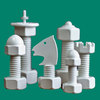
Steven Parker
231,269 PointsI don't think you can; but since the nature of programs is that they (generally) perform steps sequentially, it only makes sense to use the order to get the desired result.
Why would you want to do otherwise?
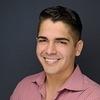
ian izaguirre
3,220 PointsMy final solution is :
"use strict";
const scanNumberRange = topNumber => {
var message;
var i;
const multipleOf = someNumber => i % someNumber === 0;
const print = foundMessage => console.log ( foundMessage || i );
for ( i = 1; i <= topNumber; i++ ) {
message = '';
if ( multipleOf(3) ) {
message += 'fizz';
}
if ( multipleOf(5) ) {
message += 'buzz';
}
print(message);
}
};
scanNumberRange(100);
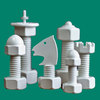
Steven Parker
231,269 PointsThat's exactly what I was talking about. See how the order is still important? If you tested for 5 first, it would print "buzzfizz" instead of "fizzbuzz" for the 15's. But it's clean and concise ... good job!
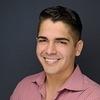
ian izaguirre
3,220 PointsThank you :-)
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsThank you for answering, you have consistently been incredibly helpful with all my questions :) . I was asking, because I think ( but don't know if its a correct assumption?) making the order not matter would make the program more flexible and thus better. I was imagining a scenario where I had 20 or more blocks of multiples I would need to add to my code, and then I knew that it would get messy having to make sure all the number block statements where in order, since my program was dependent on the code being ordered for it to work properly.
So I thought it would be best to ask if there was another way to make my program work properly, so that it could work correctly, regardless of the order of the number block statements.
Having explored more solutions, I came up with a different answer that solves my problem. Instead of a while loop, and instead of using "else-if" and "else" statements, a new solution that works, regardless of the number block order, is found by using one "for" loop and a series of "if" statements (even though that is horrible performance wise, since there is no "else-if" or "else" in that new solution)
Steven Parker
231,269 PointsSteven Parker
231,269 PointsThere are always alternative approaches to a program, the main idea is to find one that produces a reasonably compact solution that performs well and is easy to maintain. Among approaches that all fit those criteria there may not be any single "best" one.
I considered an approach that involved a series of conditionals that would eliminate the need to test specifically for 15's, but it still was order-sensitive, isn't yours? If the order of mine were rearranged it would print "BuzzFizz" for the 15's instead of "FizzBuzz".
ian izaguirre
3,220 Pointsian izaguirre
3,220 PointsI posted my final solution below , which like you said, is best if it eliminates the need to write "15" or the need to write a " 5 & 3" statement. I was proud of this lol I came up with it as an answer in an interview for a coding bootcamp, which I think I am going to do. I like this solution because I find it readable and flexible, but I could be bias because I wrote it :-).