Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial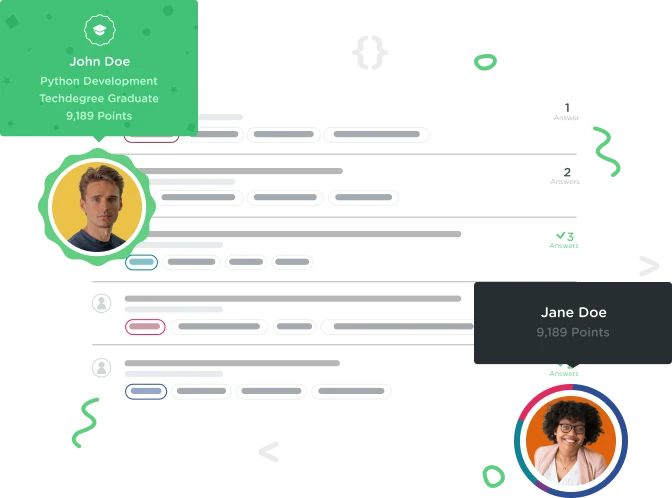

Vlatko .
2,526 PointsPlease help me understand pointers. Why can't I just use a variable instead of a pointer that points to it?
I've rewatched this video over 5 times and I still can't understand what the use of pointers is or how they work.
The tutorial's code starts with:
char *letter;
char c = 'k';
letter = &c;
printf("%c is always the same as %c\n", c, *letter);
To my understanding, *letter is a pointer and can point to a memory address of a variable. What does it mean it can point to a memory address? What is that memory address?
In the second line, a char variable is created with a value of k.
In the third line, letter points to the address of c. Is "letter" considered a pointer here? If so, why doesn't it have the preceding asterisk? Also, the ampersand is pointing to the address of the variable c, what does that mean? What's its address?
Looking at the code above, in the print statement, it seems that *letter is just pointing to the value of c, which is k.
When the value of c is changed from k to q in the example below, the value of of *letter is changed as well to q, correct?
c = 'q';
printf("%c is always the same as %c\n", c, *letter);
This happens because *letter is pointing to &c, and because the value of c changed to q, *letter is now q as well. However, what confuses me is, if this happens, couldn't we just write the code like so and keep it simple?
c = 'q';
printf("%c is always the same as %c\n", c, c);
If the pointer *letter just equals the same value of c, and if the value of c is changed, why can't we just print the value of c by using the variable c both times instead of using the pointer the second? Why is the pointer *letter needed at all?
Actually, while writing this question out, I realized that the pointer *letter will work with any char variable's address, so if we make a new char variable and point *letter to its address, it'll take on the value of the new variable. I guess this could be useful if you want to only write a pointer once then use it to point to different variables down the line, but I'm still wondering why you wouldn't just use the variables themselves instead of using a pointer. I hope someone can clear that out for me using a different example.
1 Answer

ixrevo
32,051 PointsHi!
There is good article on Wikipedia about pointers http://en.wikipedia.org/wiki/Pointer_(computer_programming)
I hope it will help you better understand what and why it is.
In a couple of words, pointer needs a very small amount of memory to be stored. And if you have a huge string variable or a massive with a lot of elements and you send it to a function just as pointer, it is faster and cheeper for memory, whether you send it in normal way as value.
Another time, when you call someFunction( hugeVariable )
, this function will copy the value of hugeVariable. But when you call someFunction( &pointerToHugeVariable )
, the function won't copy the value of the hugeVariable, instead it will have access directly to this variable with no need to copy it. And to grant this access, you use pointer.
Pointer is a kind of variable that just keeps the memory address (imagine computer memory as an apartment building or hotel, and memory address is a number of some room or apartment in this building).
Oh! There is the better example. Computer memory like a spread sheet. Every cell has own particular address, such as A1, C23, CG456. And pointer is a note with the address of particular cell. So, if someone needs access or value of the cell, to tell him address (pointer) is much faster than write down this value and send it to this someone.
But in some cases, for example, if value is small, you have no need in pointers, you can just copy this value easily.
I hope I was helpful and didn't confused you more than you was.
Toby Hodkinson
4,425 PointsToby Hodkinson
4,425 PointsThanks, that was a really good answer!
Vlatko .
2,526 PointsVlatko .
2,526 PointsThank you, I think I got it now:
A pointer just points to the location where a function needs to find the variable's value, but if we use the variable instead of a pointer, the variable makes a copy of its value inside the function, Since the variable has been declared/used before, there will now be multiple copies of the variable in the code, taking up more space in memory. More space in memory equals slower programs.
House Trigo
36,620 PointsHouse Trigo
36,620 PointsThis is a really helpful and spot on definition of a pointer. Well done!
georgekiknadze
Courses Plus Student 6,307 Pointsgeorgekiknadze
Courses Plus Student 6,307 PointsI was confused but this is really great answer. Thanks !
kavinduwijesuriya
3,103 Pointskavinduwijesuriya
3,103 PointsThanks. Best answer !