Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial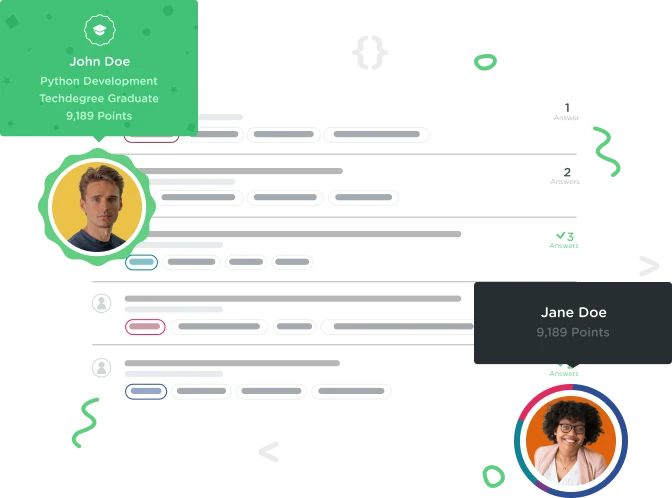

Duc Bui
14,546 PointsPlease help me with this challenge
Challenge Using the Python language, have the function KaprekarsConstant(num) take the num parameter being passed which will be a 4-digit number with at least two distinct digits. Your program should perform the following routine on the number: Arrange the digits in descending order and in ascending order (adding zeroes to fit it to a 4-digit number), and subtract the smaller number from the bigger number. Then repeat the previous step. Performing this routine will always cause you to reach a fixed number: 6174. Then performing the routine on 6174 will always give you 6174 (7641 - 1467 = 6174). Your program should return the number of times this routine must be performed until 6174 is reached. For example: if num is 3524 your program should return 3 because of the following steps: (1) 5432 - 2345 = 3087, (2) 8730 - 0378 = 8352, (3) 8532 - 2358 = 6174.
For example: Input:2111
Output:5
Input:9831
Output:7
def KaprekarsConstant(num):
# code goes here
import math
count = 0
def numminusreversednum(anum):
a = math.floor(anum/1000)
b = math.floor((anum - a * 1000)/100)
c = math.floor((anum - a * 1000 - b * 100)/10)
d = num - a * 1000 - b * 100 - c * 10
alist = [str(int(d)), str(int(c)), str(int(b)), str(int(a))]
reversednum = int(''.join(alist))
output = abs(anum - reversednum)
return output
while num != 6174:
num = numminusreversednum(num)
count += 1
return count
# keep this function call here
print KaprekarsConstant(raw_input())
Please correct my codes, tks!
1 Answer

Eduardo Valencia
12,444 PointsHi. This is not exact what you asked, but here is how I did it. Maybe this will help you out:
def sort_number(num, direction):
''' Takes a number and puts the digits in ascending order.'''
ascending: list = []
for digit in str(num):
ascending.append(int(digit))
ascending.sort(reverse=True if direction is 'descending' else False)
sort_number: str = ''
for digit in ascending:
sort_number += str(digit)
return int(sort_number)
def difference_between_ascending_descending(num):
''' Gets the number with its digits in ascending order and in descending order. It returns the
smallest of the two subtracted from the largest. '''
ascending = sort_number(num, 'ascending')
descending = sort_number(num, 'descending')
if ascending == descending:
return 0
elif ascending > descending:
return ascending - descending
else:
return descending - ascending
def to_x_places(num, places):
''' Multiplies a number by an power of ten so that it is a certain number of places long. '''
current_places = len(str(num))
return num * 10 ** (places - current_places)
def kaprekars_constant(num):
''' Returns how many times it takes to turn a four digit number into 6_174 if you
calculate its digits in ascending order and in descending order, and then subtract
the smallest from the largest. Its output goes through the same process until it
turns into 6_174. '''
current_num = num
iterations = 0
while current_num != 6_174:
current_num = difference_between_ascending_descending(
to_x_places(current_num, 4))
iterations += 1
if iterations == 1000:
break
return iterations
By the way, you should use underscores (_
) to separate words in Python, as this makes your code more readable.