Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial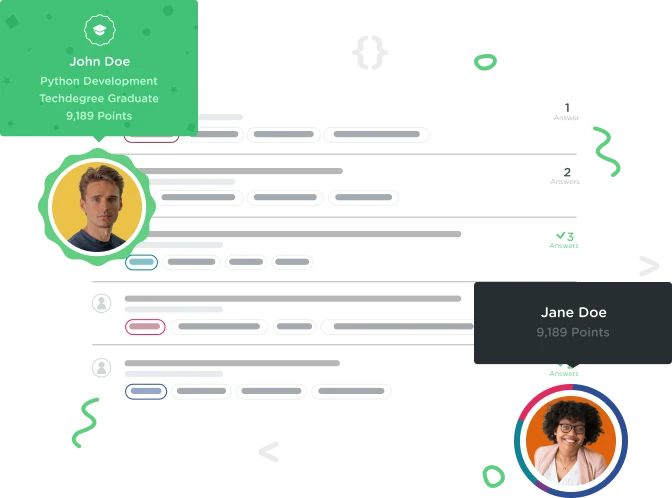

kasaltrix
9,491 PointsPlease help me with this error handling code
I have completed the challenge but I am trying to take it one step further. I am trying to check whether the value for a key in the dictionary is nil or not using the second guard statement, but it doesn't work as expected. According to the code, it should throw an invalidKey error but it doesn't. I don't know what am I doing wrong. Any help would be quite useful!!!
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard ((data) != nil) else {
throw ParserError.emptyDictionary
}
guard ((data?["someKey"]) != nil) else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do{
try parser.parse()
}
catch ParserError.emptyDictionary{
print("Empty Dictionary")
}
catch ParserError.invalidKey{
print("No valid data")
}
2 Answers

Dhanish Gajjar
20,185 PointsThe implementation of the parse function is much simpler ! This should work !
enum ParserError: Error {
case emptyDictionary
case invalidKey
}
struct Parser {
var data: [String : String?]?
func parse() throws {
guard let data = data else {
throw ParserError.emptyDictionary
}
guard let _ = data["someKey"] else {
throw ParserError.invalidKey
}
}
}
let data: [String : String?]? = ["someKey": nil]
let parser = Parser(data: data)
do {
try parser.parse()
} catch ParserError.emptyDictionary {
print("Nil Data")
} catch ParserError.invalidKey {
print("Invalid Key")
}

Angus Muller
iOS Development Techdegree Graduate 12,473 PointsHi kasaltrix did you ever find your answer?I have written code that passes the task but I find it won't print nil data when there is nil. Gus
kasaltrix
9,491 Pointskasaltrix
9,491 PointsThanks for the reply Dhanish, but this is not what I asked for. As I wrote in my question, I have already completed the challenge and the code that you have provided is for completing the challenge. My question was how to check whether the value passed to someKey is nil or not using the guard condition. If you have know the answer to this, please reply back. Once again thank you for your time :)