Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial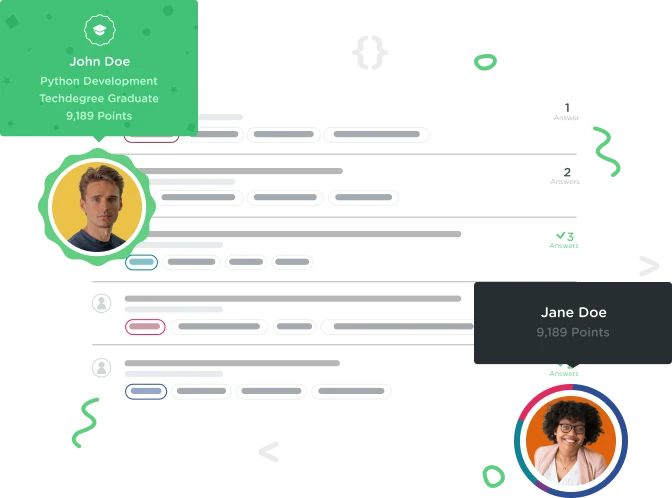

Razvan Sighinas
2,018 PointsPlease help me with this errors
package razvansighinas.funfactsapl;
import android.app.Activity;
import android.os.Bundle;
import android.view.Menu;
import android.view.MenuItem;
import android.view.View;
import android.widget.Button;
import android.widget.TextView;
import java.util.Random;
public class funfacts2 extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_funfacts2);
// Declare our View variables and assign them the views from the Layout file
final TextView factLabel = (TextView) findViewById(R.id.factTextView);
Button showFactButton = (Button) findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View view) {
// The button was clicked so update the fact label with a new fact.
String fact = "";
// randomly select a fact
Random randomGenerator = new Random(); //Construct a new Random number generator
int randomNumber = randomGenerator.nextInt(3);
/* convert the random number into txt fact.
0.Salsa e un dans frumos dar nu la fel ca bachata.
1.Cand dansez uit de tot ce e rau in lume si in viata mea.
2.Kizomba e noul dans pe care vreau sa-l imbunatatesc.
*/
//if randomnumber equals 0 then
if (randomNumber == 0) {
// set fact equal to Salsa fact
fact = "Salsa e un dans frumos dar nu la fel ca bachata.";
} else if (randomNumber == 1) {
fact = "Cand dansez uit de tot ce e rau in lume si in viata mea.";
} else if (randomNumber == 2) {
fact = "Kizomba e noul dans pe care vreau sa-l imbunatatesc.";
} else {
fact = "Imi pare rau dar este o eroare.";
}
//update the label with our dynamic fact
factLabel.setText(fact);
}
;
showFactButton.setOnClickListener<listener>
}
@Override
public boolean onCreateOptionsMenu (Menu menu){
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.funfacts2, menu);
return true;
}
@Override
public boolean onOptionsItemSelected (MenuItem item){
// Handle action bar item clicks here. The action bar will
// automatically handle clicks on the Home/Up button, so long
// as you specify a parent activity in AndroidManifest.xml.
int id = item.getItemId();
if (id == R.id.action_settings) {
return true;
}
return super.onOptionsItemSelected(item);
}
}
Error:(60, 56) error: <identifier> expected this error is for<listener>
showFactButton.setOnClickListener<listener>
Error:(83, 6) error: reached end of file while parsing
Error:Execution failed for task ':app:compileDebugJava'.
> Compilation failed; see the compiler error output for details.
Please help me
thank you
2 Answers

Daniel Hartin
18,106 PointsHi
It looks to me like you have simply used <> brackets when you needed to use normal parenthesis like the below
showFactButton.setOnClickListener(listener);
Hopefully this should clear it up for you
Thanks Daniel

aakarshrestha
6,509 PointsHi,
Any argument must be within "( )" not on "<>". Also, if you read the logcat, it is displaying where the error is. Change "showFactButton.setOnClickListener<listener>" to "showFactButton.setOnClickListener(listener)". It should work.
Happy coding!
Razvan Sighinas
2,018 PointsRazvan Sighinas
2,018 PointsAfter I made the change like you said when I try to run it gives me 9 errors.
Daniel Hartin
18,106 PointsDaniel Hartin
18,106 PointsHi Sorry for the late reply, looks like you've got a random semi-colon in your code which will be throwing things. I have copied the entire class below and made changes
Hopefully this solves it, if you were still struggling
Thanks Daniel
}